Types of References in Java
Last Updated :
06 Oct, 2023
In Java there are four types of references differentiated on the way by which they are garbage collected.
- Strong References
- Weak References
- Soft References
- Phantom References
Prerequisite: Garbage Collection
- Strong References: This is the default type/class of Reference Object. Any object which has an active strong reference are not eligible for garbage collection. The object is garbage collected only when the variable which was strongly referenced points to null.
MyClass obj = new MyClass ();
Here ‘obj’ object is strong reference to newly created instance of MyClass, currently obj is active object so can’t be garbage collected.
obj = null;
//'obj' object is no longer referencing to the instance.
So the 'MyClass type object is now available for garbage collection.
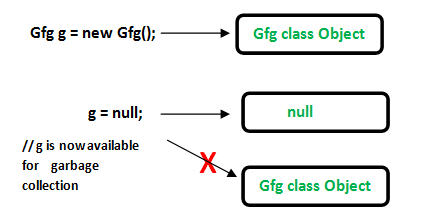
class Gfg
{
}
public class Example
{
public static void main(String[] args)
{
Gfg g = new Gfg();
g = null ;
}
}
|
- Weak References:Â Weak Reference Objects are not the default type/class of Reference Object and they should be explicitly specified while using them.
- This type of reference is used in WeakHashMap to reference the entry objects .
- If JVM detects an object with only weak references (i.e. no strong or soft references linked to any object object), this object will be marked for garbage collection.
- To create such references java.lang.ref.WeakReference class is used.
- These references are used in real time applications while establishing a DBConnection which might be cleaned up by Garbage Collector when the application using the database gets closed.
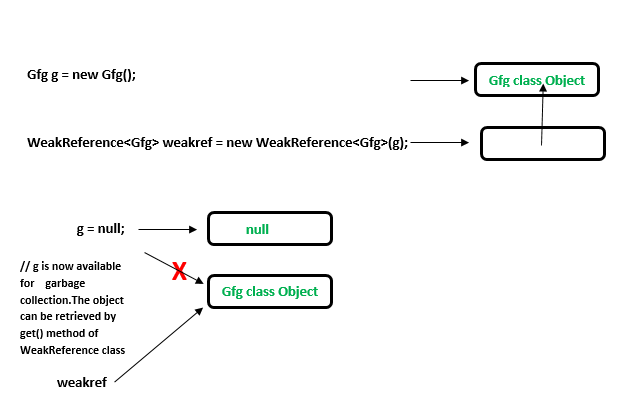
import java.lang.ref.WeakReference;
class Gfg
{
public void x()
{
System.out.println( "GeeksforGeeks" );
}
}
public class Example
{
public static void main(String[] args)
{
Gfg g = new Gfg();
g.x();
WeakReference<Gfg> weakref = new WeakReference<Gfg>(g);
g = null ;
g = weakref.get();
g.x();
}
}
|
Output:
GeeksforGeeks
GeeksforGeeks
Two different levels of weakness can be enlisted: Soft and Phantom
Share your thoughts in the comments
Please Login to comment...