Top Interview Questions and Answers on Quick Sort
Last Updated :
13 Mar, 2024
Quick Sort is a popular sorting algorithm used in computer science. In our article “Top Interview Questions and Answers on Quick Sort“, we present a collection of essential coding challenges focused on Quick Sort algorithms. These problems are carefully selected to help you sharpen your problem-solving skills and prepare effectively for interviews. Let’s begin on this journey together and master the art of Quick Sort algorithms by solving top Quick Sort problems.
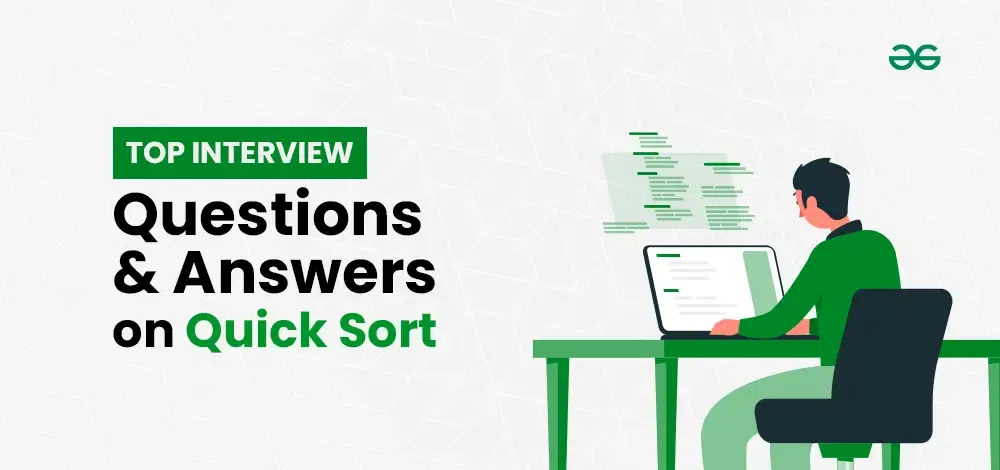
Top Interview Questions and Answers on Quick Sort
Top Interview Questions and Answers on Quick Sort:
Question 1: What is the Quick Sort algorithm?
Answer: Quicksort uses a divide-and-conquer approach, dividing an array into subarrays based on a pivot element. The pivot is positioned with elements less than it on the left and greater than it on the right. This process is repeated recursively until each subarray contains only one element. The sorted subarrays are then combined to form the final sorted array.
Question 2: How does QuickSort work?
Answer: QuickSort is a divide-and-conquer sorting algorithm that works as follows:
- Choose a pivot element: Select an element from the array as the pivot.
- Partition the array: Rearrange the array such that all elements smaller than the pivot are on the left side of the pivot, and all elements larger than the pivot are on the right side. The pivot element is placed in its correct sorted position.
- Recursively sort the two subarrays: Apply QuickSort to the left subarray (elements smaller than the pivot) and the right subarray (elements larger than the pivot).
Question 3: What do you understand about partitioning in the context of the Quick Sort algorithm?
Answer: The process of splitting an array into two smaller arrays is known as partitioning. Using partitioning, the Quick Sort algorithm splits an array into two smaller arrays: one with elements smaller than the pivot element and the other with elements larger than the pivot element. Each of these smaller arrays is then sorted recursively by the Quick Sort algorithm.
Question 4: Is Quick Sort suitable for use on large datasets? Why or why not?
Answer: The worst-case time complexity of Quick Sort is O(n^2), which occurs when the array is already sorted or reverse sorted. In such cases, Quick Sort may not be the most efficient choice. Hence, for large dataset using this algorithm is not an effective choice.
Question 5: What are the advantages and disadvantages of using Quick Sort over other sorting algorithms?
Answer: The Quick Sort algorithm has several advantages over other sorting algorithms:
Advantages of QuickSort over other sorting algorithms:
- Efficiency: QuickSort has an average time complexity of O(n log n), which is optimal for most sorting scenarios.
- In-place sorting: QuickSort sorts the array in-place, meaning it doesn’t require additional memory space.
- Simplicity: The algorithm is relatively easy to understand and implement.
Disadvantages of QuickSort over other sorting algorithms:
- Worst-case performance: QuickSort has a worst-case time complexity of O(n^2), which occurs when the array is already sorted or nearly sorted.
- Tail recursion: QuickSort uses tail recursion, which can lead to stack overflow errors for very large arrays.
- Not stable: QuickSort is not a stable sorting algorithm, meaning it may change the order of equal elements in the array.
Question 6: What’s the difference between merge sort and quick sort?
Answer: The main difference between Merge Sort and Quick Sort is their approach to sorting:
Merge Sort:
- Uses a divide-and-conquer strategy by dividing the array into two halves, sorting each half, and then merging them.
- Guarantees a time complexity of O(n log n) in all cases.
- Requires additional space for merging the sub-arrays.
Quick Sort:
- Uses a divide-and-conquer strategy by selecting a pivot, partitioning the array based on the pivot, and then recursively sorting the sub-arrays.
- Has an average time complexity of O(n log n) but can degrade to O(n^2) in the worst case.
- It is an in-place sorting algorithm, meaning it doesn’t require additional space for sorting.
Question 7: What’s the best way to perform the partition step during Quick Sort?
Answer: The best way to perform the partition step during Quick Sort is by selecting a good pivot element, typically the median of the first, middle, and last elements of the array. Using a random pivot is also a common and effective strategy in Quick Sort. By randomly selecting a pivot element from the array, you can reduce the likelihood of encountering worst-case scenarios and improve the overall performance of the algorithm. This approach can help achieve a good balance in partitioning the array and enhance the efficiency of Quick Sort.
Question 8: Are there any limitations with Quick Sort? If yes, then what are they?
Answer: Yes, Quick Sort has limitations:
- Worst-case time complexity: Quick Sort can have a worst-case time complexity of O(n^2) if the pivot selection is poor, leading to inefficient performance.
- Not stable: Quick Sort is not a stable sorting algorithm, meaning the relative order of equal elements may not be preserved.
Question 9: What happens if all the items being sorted have the same value?
Answer: If all the items being sorted have the same value, Quick Sort’s performance degrades to its worst-case time complexity of O(n^2). This occurs because the pivot selection becomes ineffective in partitioning the array, leading to inefficient sorting.
Question 10: What are some ways that Quick Sort could be optimized?
Answer: Some ways to optimize Quick Sort include:
- Choosing a good pivot: Selecting a good pivot element, such as the median of three elements, can improve partitioning and reduce the likelihood of worst-case time complexity.
- Randomized pivot selection: Using a random pivot can help avoid worst-case scenarios and improve the overall performance of Quick Sort.
- Optimizing the base case: Implementing an alternative sorting algorithm for small sub-arrays can reduce the overhead of recursion in Quick Sort.
Question 11: Is it possible to modify the pivot element while performing the partition step?
Answer: Yes, it is possible to modify the pivot element while performing the partition step in Quick Sort.
Question 12: Do you think Quick Sort is a stable algorithm? Why or why not?
Answer: No, Quick Sort is not a stable sorting algorithm.
Stability refers to the property that elements with equal values retain their original order after sorting. In Quick Sort, the order of equal elements may change during the partitioning step.
Question 13: What is the time complexity of QuickSort in the average case?
Answer: The average-case time complexity of QuickSort is O(n log n) due to its efficient, balanced partitioning.
Question 14: What is the time complexity of QuickSort in the Worst case?
Answer: The worst-case time complexity of QuickSort is O(n^2) due to its efficient, balanced partitioning.
Question 15: What is the difference between Quick Sort and Merge Sort?
Answer: Quick Sort is an in-place sorting algorithm with an average time complexity of O(n log n), while Merge Sort is a stable sorting algorithm with a guaranteed time complexity of O(n log n).
Question 16: What is the difference between Quick Sort and Heap Sort?
Answer: Quick Sort is a divide-and-conquer sorting algorithm, while Heap Sort is a selection sort algorithm. Quick Sort has an average time complexity of O(n log n), while Heap Sort has an average time complexity of O(n log n).
Question 17: When should Quick Sort be used?
Answer: Quick Sort should be used when sorting large arrays where efficiency is a priority.
Question 18: When should other sorting algorithms be used instead of Quick Sort?
Answer: Other sorting algorithms should be used when stability or worst-case performance is a concern.
Question 19. What is the iterative version of Quick Sort?
Answer: The iterative version of Quick Sort uses a stack to implement the recursion. It is more efficient than the recursive version for large arrays.
Question 20: How can Quick Sort be parallelized?
Answer: Quick Sort can be parallelized by partitioning the array into multiple subarrays and sorting each subarray concurrently.
Related Articles:
Share your thoughts in the comments
Please Login to comment...