Ternary Operator in Programming
Last Updated :
26 Mar, 2024
The Ternary Operator is similar to the if-else statement as it follows the same algorithm as of if-else statement but the ternary operator takes less space and helps to write the if-else statements in the shortest way possible. It is known as the ternary operator because it operates on three operands.
What is a Ternary Operator?
The ternary operator is a conditional operator that takes three operands: a condition, a value to be returned if the condition is true, and a value to be returned if the condition is false. It evaluates the condition and returns one of the two specified values based on whether the condition is true or false.
Syntax of Ternary Operator:
The Ternary operator can be in the form
variable = Expression1 ? Expression2 : Expression3;
It can be visualized into an if-else statement as:Â
if(Expression1)
{
variable = Expression2;
}
else
{
variable = Expression3;
}
Working of Ternary Operator :
The Ternary operator can be in the form
variable = Expression1 ? Expression2 : Expression3;
The working of the Ternary operator is as follows:
- Step 1: Expression1Â is the condition to be evaluated.
- Step 2A: If the condition(Expression1) is True then Expression2 will be executed.
- Step 2B: If the condition(Expression1) is false then Expression3 will be executed.
- Step 3:Â Results will be returned
Flowchart of Conditional/Ternary Operator:
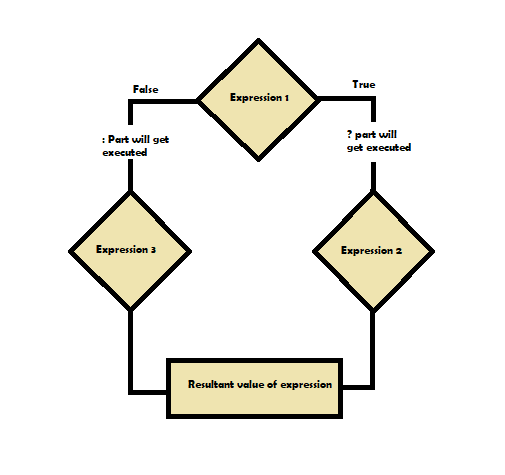
Flowchart of ternary operator
Ternary Operator in C:
Here are the implementation of Ternary Operator in C language:
C
#include <stdio.h>
int main() {
// Define two variables
int a = 5;
int b = 9;
// Use ternary operator to find the maximum
int max = (a > b) ? a : b;
// Output the maximum value
printf
Ternary Operator in C++:
Here are the implementation of Ternary Operator in C++ language:
C++
#include <iostream>
using namespace std;
int main() {
// Define two variables
int a = 5;
int b = 9;
// Use ternary operator to find the maximum
int max = (a > b) ? a : b;
// Output the maximum value
cout << "Maximum: " << max << endl; // Output: Maximum: 9
return 0;
}
Ternary Operator in Java:
Here are the implementation of Ternary Operator in java language:
Java
public class TernaryOperatorExample {
public static void main(String[] args) {
// Define two variables
int a = 5;
int b = 9;
// Use ternary operator to find the maximum
int max = (a > b) ? a : b;
// Output the maximum value
System.out.println("Maximum: " + max); // Output: Maximum: 9
}
}
Ternary Operator in Python:
Here are the implementation of Ternary Operator in python language:
Python3
# Define two variables
a = 5
b = 9
# Use ternary operator to find the maximum
max_val = a if a > b else b
# Output the maximum value
print("Maximum:", max_val) # Output: Maximum: 9
Ternary Operator in C#:
Here are the implementation of Ternary Operator in C# language:
C#
using System;
class Program {
static void Main() {
// Define two variables
int a = 5;
int b = 9;
// Use ternary operator to find the maximum
int max = (a > b) ? a : b;
// Output the maximum value
Console.WriteLine("Maximum: " + max); // Output: Maximum: 9
}
}
Ternary Operator in Javascript:
Here are the implementation of Ternary Operator in javascript language:
JavaScript
// Define two variables
let a = 5;
let b = 9;
// Use ternary operator to find the maximum
let max = (a > b) ? a : b;
// Output the maximum value
console.log("Maximum: " + max); // Output: Maximum: 9
Applications of Ternary Operator:
- Assigning values based on conditions.
- Returning values from functions based on conditions.
- Printing different messages based on conditions.
- Handling null or default values conditionally.
- Setting properties or attributes conditionally in UI frameworks.
Best Practices of Ternary Operator:
- Use ternary operators for simple conditional expressions to improve code readability.
- Avoid nesting ternary operators excessively, as it can reduce code clarity.
- Use parentheses to clarify complex conditions and expressions involving ternary operators.
Conclusion:
The conditional operator or ternary operator is generally used when we need a short conditional code such as assigning value to a variable based on the condition. It can be used in bigger conditions but it will make the program very complex and unreadable.
Share your thoughts in the comments
Please Login to comment...