SQLAlchemy Cascading Deletes
Last Updated :
17 Apr, 2023
SQLAlchemy is a powerful ORM (Object-Relational Mapping) library for Python that allows developers to interact with relational databases using Python objects. It provides a rich set of tools for querying, updating, and manipulating data, making it a popular choice for building web applications and other software that requires working with databases.
One of the most useful features of SQLAlchemy is its support for cascading deletes. This feature allows you to automatically delete related records in a database when you delete a record that has dependencies. In this article, we will discuss SQLAlchemy cascading deletes in more detail and provide examples to help you better understand how it works.
Explanation:
- Cascading deletes are a way to maintain referential integrity in a database. Referential integrity ensures that relationships between records in different tables are maintained and that orphaned records are not left behind. When you delete a record from a table that has dependencies, you need to make sure that the dependent records are also deleted or updated. This can be a time-consuming and error-prone process, which is where cascading deletes come in.
- SQLAlchemy provides support for cascading deletes through the use of cascading delete and delete-orphan options. These options are used to specify how related records should be treated when a parent record is deleted.
- The cascading delete option is used to specify that related records should be deleted when a parent record is deleted. This option is set on a relationship between two tables. For example, if you have a table called “users” and a table called “posts”, and each post belongs to a user, you would set the cascading delete option on the relationship between the two tables. This would ensure that when a user is deleted, all of their posts are also deleted.
- The delete-orphan option is used to specify that related records should be deleted when they are orphaned. An orphaned record is a record that no longer has a parent record. For example, if you have a table called “users” and a table called “comments”, and each comment belongs to a user, you would set the delete-orphan option on the relationship between the two tables. This would ensure that when a user is deleted, all of their comments are also deleted.
For this article, we will be using a simple database with two tables: “users” and “posts“. Each post belongs to a user, and each user can have multiple posts. The database schema is shown below:
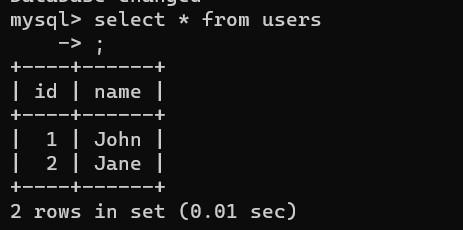
Users
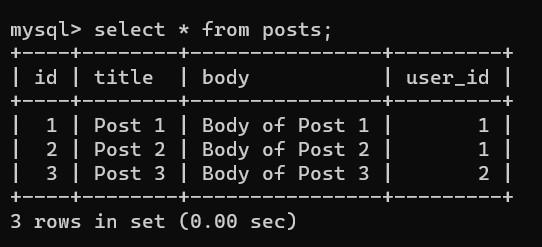
Posts
The “posts” table has a foreign key constraint that references the “users” table. The ON DELETE CASCADE option specifies that when a user is deleted, all of their posts should also be deleted.
To perform SQLAlchemy Cascading Deletes on the tables created in the above code, we can use the following Python code:
Don’t forget to update the code with your password and database name respectively
Python3
from sqlalchemy import create_engine, ForeignKey, Column, Integer, String, Text
from sqlalchemy.orm import sessionmaker, relationship
from sqlalchemy.ext.declarative import declarative_base
engine = create_engine(
Session = sessionmaker(bind = engine)
session = Session()
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key = True )
name = Column(String( 50 ), nullable = False )
posts = relationship( 'Post' , backref = 'user' , cascade = 'all, delete' )
class Post(Base):
__tablename__ = 'posts'
id = Column(Integer, primary_key = True )
title = Column(String( 100 ), nullable = False )
body = Column(Text, nullable = False )
user_id = Column(Integer, ForeignKey( 'users.id' ), nullable = False )
user = session.query(User).filter_by(name = 'John' ).first()
session.delete(user)
session.commit()
user = session.query(User).filter_by(name = 'John' ).first()
print (user)
posts = session.query(Post).filter_by(user_id = 1 ). all ()
print (posts)
|
Updated Table after performing SQLAlchemy Cascading:
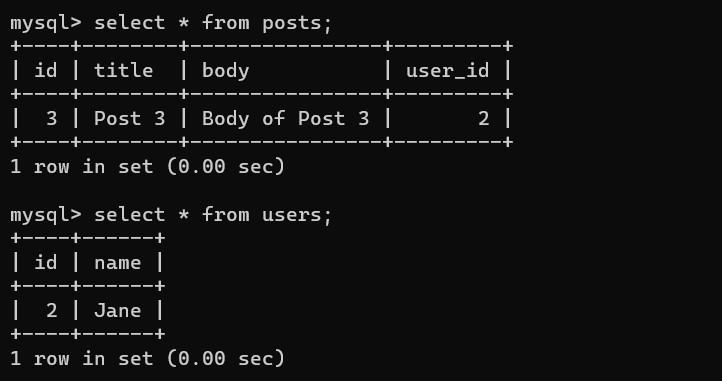
Output Image after executing code
Share your thoughts in the comments
Please Login to comment...