Spring Security is a highly flexible and customizable security framework designed for Java applications, particularly those developed using the Spring Framework. It offers extensive security services for enterprise-level Java EE-based software applications. At its Core, Spring Security is concerned with two vital aspects of software application security: authentication, which involves verifying users’ identities, and authorization, which involves determining the actions that users are allowed to perform.
In this article, We will look into 30+ Spring Interview Questions and Answers tailored for both freshers and experienced professionals with 1, 5, and 10 years of experience. Here we cover everything about Spring Security Interview Questions including the basics of authentication and authorization, configuration strategies, cross-site scripting prevention, securing REST APIs, and best practices for using OAuth2 and JWT with Spring Security.
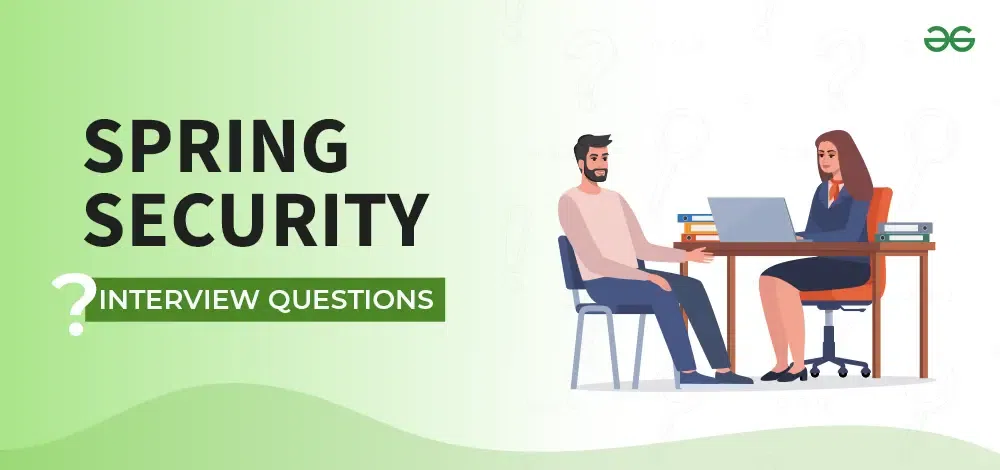
Spring Security Interview Questions And Answer
Spring Security Interview Questions for Freshers
1. What is Spring Security?
Spring Security is an effective, customizable Java framework for authentication, authorization, and access control in Spring-primarily-based applications. It protects our applications from unauthorized access and also manages user permissions.
- It performs error handling, protects the resources, and manages login authentication. Those are the principal functions of Spring Security.Â
- It has a flexible configuration, secure applications, and smooth and easy integration.
- This is broadly used in enterprise applications.
2. What are the key features of Spring Security?
Some of the core features of Spring Security are depicted below:
- Authentication: Process of Identifying the person trying to access the application.
- Authorization: Process of Identifying if the person is allowed to do this operation.
- Principal: To know, is the person that we have identified through an authentication process. It is also called the Currently logged-in user that we store in session.
- Protection: This protects against most common threats like cross-site scripting and SQL injection.
- Granted Authority: These are the groups of permissions that are allowed for a user.
3. Difference between Authentication and Authorization in Spring Security.
Features
| Authentication
| Authorization
|
---|
Definition
| It is a process used to verify user’s identity.
| It determines that within the application, which task a user is allowed to do.
|
Working
| Checks credentials like username and password provided by user against stored credentials.
| To determine resources, it uses user’s identity and pre-defined access control rules.
|
Performance
| It is fast.
| After authentication, it authorizes.
|
Result
| User gets authenticated and security token granted to them.
| Users get access to specific features based on the rules.
|
Below is a simple diagram to know about Authentication and Authorization.
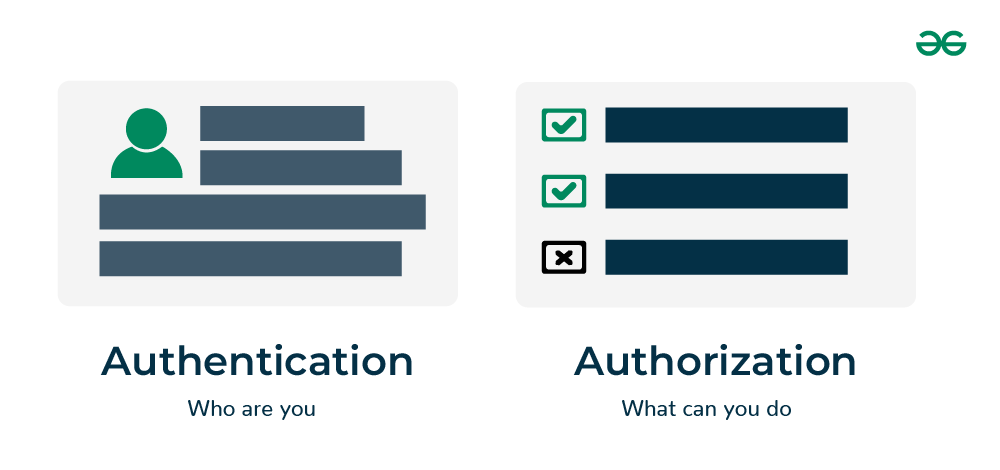
Know more about Authentication and Authorization in Spring Security
4. How to configure Authentication in Spring Security?
To configure Authentication in Spring Security (not basic authentication) we need to follow below procedure:
- Extend WebSecurityConfigureAdapter in a custom class and use @EnableWebSecurity annotation.
- Overrides configure(AuthenticationManagerBuilder) method.
- Configure type of auth, user, password and role.
- Now, this will automatically create and configure a new Authentication Manager and then Spring Security will use this Authentication Manager instead of using the default one.
5. How to configure Authorization in Spring Security?
To configure Authorization in Spring Security, follow the below procedure:
- Extend WebSecurityConfigureAdapter in a custom class and use @EnableWebSecurity annotation.
- Overrides configure(HttpSecurity) method.
- Now Configure http.authorizeRequests()
Java
@Override
protected void configure(HttpSecurity http) throws Exception
{
http.authorizeRequests()
.antMatchers("/author/admin")
.hasRole("ADMIN")
.antMatchers("/author/user")
.hasRole("USER")
.antMatchers("/")
.permitAll()
.and()
.formLogin();
}
6. What is the Latest Version of Spring Security and What’s New in It?
Spring Security 6 is the latest version of Spring Security and it has introduced many new features. Following are the most useful features that are introduced in Spring Security 6.
- Now we can automatically enable .cors() if CorsConfigurationSource bean is present
- It simplifies the configuration of the OAuth2 Client component model
- It will improve the detection of CVE-2023-34035
- It added OIDC Back-channel Logout Support for OAuth 2.0
- Make Configurable RedirectStrategy status code
- Make Configurable HTTP Basic request parsing
7. Explain basic authentication in Spring Security.
Below are the steps to implement basic authentication using username and password.
- Add Spring Security Starter Dependency i.e. spring-boot-starter-security to pom.xml file.
- Extend WebSecurityConfigureAdapter in a custom class and use @EnableWebSecurity annotation.
- Override configure(HttpSecurity) method to specify security rules.
Java
@EnableWebSecurity
public class MySecurityAppConfig
extends WebSecurityConfigurerAdapter {
// Configure the basic authentication through configure
// method
@Override
protected void configure(HttpSecurity http)
throws Exception
{
http.authorizeHttpRequests()
.anyRequest()
.authenticated()
.and()
.httpBasic();
}
}
Now, the application has basic authentication using the provided username and password.
Know more about Basic Authentication in Spring Security
8. How to Enable and Disable CSRF in Spring Security?
Cross-Site Request Forgery (CSRF) is an attack. End users performs unwanted actions on a web application in which they are currently authenticated, and it is caused due to this web attack. It is one of the most severe vulnerabilities in Spring Security. If we want to enable or want to disable the CSRF protection in Spring Security, we have to configure it in the application’s security configuration class. Below is the code sample for enable and disable this:
Java
@Configuration
public class GFG extends WebSecurityConfigurerAdapter {
--------Your Code-- -------@Value(
"${security.enable-csrf}")
private boolean csrfEnabled;
--------Your Code-- -------
@Override protected void
configure(HttpSecurity http) throws Exception
{
super.configure(http);
if (!csrfEnabled) {
http.csrf().disable();
}
}
}
Note: In Spring Security, by default the CSRF (Cross-Site Request Forgery) protection is enabled.
Know more about:
9. What is a Filter Chain in Spring Security?
To perform most of the security features, Spring Security utilizes the filter chain. Internally Spring Security maintains a filter chain with each filter having a specific responsibility. Filters are added or removed from the system depending on needed services. This is how the filter chain works in a web application:
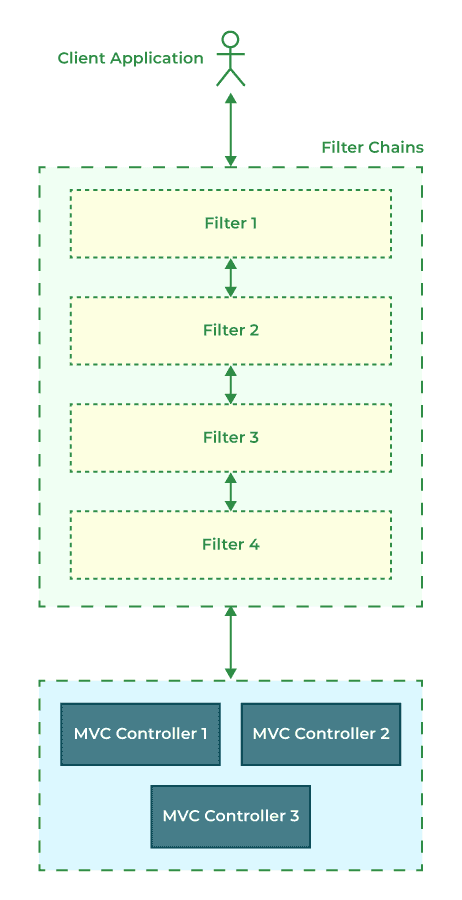
In the above image,
- Client sends the request for a resource.
- The application container creates a filter chain to execute an incoming request.
- After that each requests i.e. the HttpServletRequest passes through that filter chain based upon the path of the request URI.
Know more about Spring Security – Filter Chain with Example
10. When to Use Spring Security antMatcher()?
The antMatchers() is used to configure the URL paths. The URL paths either can be permitted or denied to the user’s http request. It will be done according to the role or the authorization of that particular user. Following are the rules applied on antmatchers():
- ? – matches one character.
- * – matches zero or more characters.
- ** – matches zero or more directories in a path.
Following are the rules applied on antmatchers():
- hasAnyRole()
- hasRole()
- hasAuthority()
- hasAnyAuthority()
- authenticated()
- anonymous()
Know more about Spring Security – Securing Endpoints Using antMatchers()
11. How to implement Spring Security in a simple Spring Boot application?
To implement Spring Security in any Spring Boot application is by adding the following starter dependency in the pom.xml file.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
<version>6.2.0</version>
</dependency>
Know more about Simple Authentication in Spring Boot
12. How to configure Spring Security in Spring MVC application?
To configure Security in a Spring MVC application, follow the below steps:
- Step 1: Add spring-boot-starter-security dependency in the pom.xml file.
- Step 2: Configure Spring Security by extending WebSecurityConfigureAdapter (WSCA).
- Step 3: For custom login functionality, create a simple login page.
- Step 4: Now Run the Spring MVC application, Spring Security will automatically authorize and authenticate the application.
13. How to Deny Access to All URLs in Spring Security?
To Deny Access to All URLs in Spring Security we can use denyAll() Spring Security Expressions.
Spring’s expression-based access Control has an expression called denyAll which evaluation is always false. To know that how to use this expression follow the below syntactical example:
Java
@Override
protected void configure(HttpSecurity http) throws Exception
{
http.authorizeHttpRequests()
.anyRequest()
.denyAll()
.and()
.httpBasic();
}
Know more about Spring Security – Deny Access to All URLs
14. How to Get the Current Logged in User Details in Spring Security?
We can get the Current Logged in User Details in Spring Security with the help of the Authentication class. A sample code is given below.
Java
// Principal means username
@GetMapping("/")
public String helloGfg(Principal principal,
Authentication auth, Model model)
{
// Get the Username
String userName = principal.getName();
System.out.println("Current Logged in User is: "
+ userName);
// Get the User Roles/Authorities
Collection<? extends GrantedAuthority> authorities
= auth.getAuthorities();
System.out.println("Current Logged in User Roles are: "
+ authorities);
model.addAttribute("username", userName);
model.addAttribute("roles", authorities);
return "home-page";
}
Know more about Spring Security – Get the Current Logged in User Details
15. What is Spring Security Password Encoder?
Servlet support in Spring Security includes storing passwords securely by integrating a PasswordEncoder. We can configure the PasswordEncoder implementation of Spring Security by exposing the PasswordEncoder Bean. A sample code is given below.
Java
@Configuration
@EnableWebMvc
@ComponentScan("com")
public class MySecurityAppConfig {
@Bean PasswordEncoder getPasswordEncoder()
{
return new BCryptPasswordEncoder();
}
}
There are various types of Password encoder available in Spring Security
- BCryptPasswordEncoder
- StandardPasswordEncoder
- NoOpPasswordEncoder
- Pbkdf2PasswordEncoder
- Argon2PasswordEncoder
Know more about Spring Security – Password Encoder
16. Explain the purpose of @EnableWebSecurity in Spring Security.
@EnableWebSecurity annotation gives signals to Spring to enable its web security support.
- This makes the application secured.
- This is used in conjunction along with the @Configuration annotation.
Java
@Configuration
@EnableWebSecurity
public class SecurityConfig
extends WebSecurityConfigureAdapter {
@Override
protected void configure(HttpSecurity http)
throws Exception
{
http.authorizeRequests()
.antMatchers("/hello")
.permitAll()
.anyRequest()
.authenticated()
.and()
.formLogin();
}
}
17. What is JWT in Spring Security?
JWT stands for JSON Web Token. It is widely used to exchange information between two ends as a JSON object. It securely transforms informations. It is mostly used in the case of Authorization and Information Exchange. It consists of three parts:
For example, a JWT looks like below:
NiIsInR5cCI6IkpXVCJ9 . eyJzdWIiOiIibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ . SflKxwRJSMeKKF2QT4fwpMeJf36
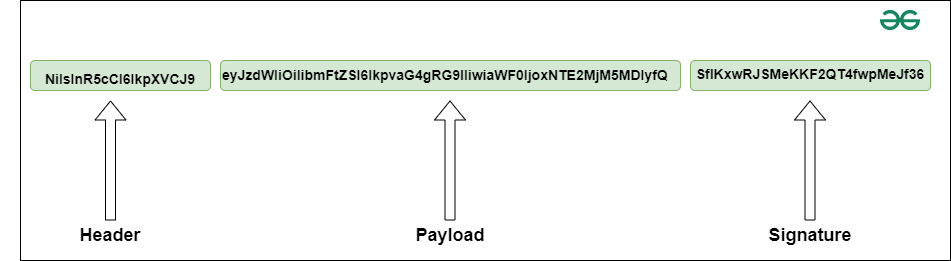
- NiIsInR5cCI6IkpXVCJ9: This is the header part and contains the algorithm and what type of token it is.
- eyJzdWIiOiIibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ: This is the data part (payload).
- SflKxwRJSMeKKF2QT4fwpMeJf36: This is the signature part. It is used to verify that the data or message does not change during the information transformation.
To know more please refer to these articles:
18. What is OAuth and OAuth2 in Spring Security?
OAuth is an open standard protocol and it is used for authorization. OAuth2 is a version of OAuth.
- OAuth2 is an authorization framework, and it enables applications such as Facebook, Twitter for obtain limited access to user accounts on HTTP service.
- OAuth2 provides authorization flows and not the authentication.
- This works by assigning the user’s authentication to the service that manages the user account and authorizes third-party applications to access that user account.
- OAuth2 is the most widely used form of OAuth.
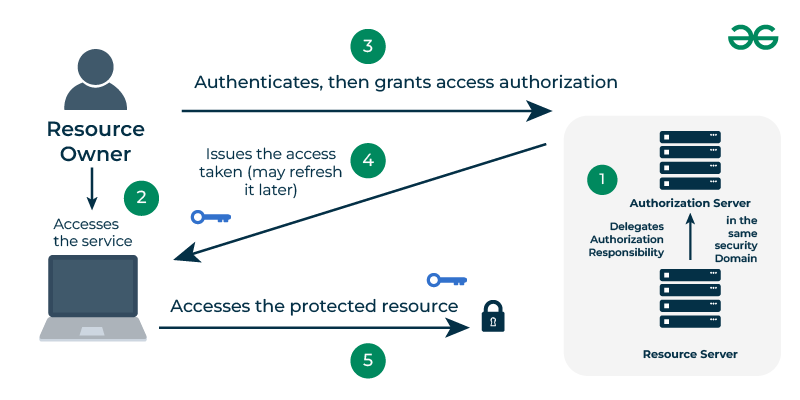
19. What is Keycloak and explain the integration of keycloak with Spring Security?
Keycloak is an open-source IAM (Identity and Access Management) solution. It is developed by Red Hat. By using this,
- We can add authentication to applications and secure services with very minimal effort.
- We don’t have to deal with user retention or user adoption.
- It provides strong authentication, user management, authorization etc.
- We can configure Keycloak with Spring Security by adding the Spring Security Adapter.
For example:
Add the dependency:
<dependency>
<groupId>org.keycloak</groupId>
<artifactId>keycloak-spring-security-adapter</artifactId>
<version>21.1.2</version>
</dependency>
Java Configuration Class:
Java
@KeycloakConfiguration
public class SecurityConfig
extends KeycloakWebSecurityConfigurerAdapter {
@Autowired
public void
configureGlobal(AuthenticationManagerBuilder auth)
throws Exception
{
auth.authenticationProvider(
keycloakAuthenticationProvider());
}
@Bean
@Override
protected SessionAuthenticationStrategy
sessionAuthenticationStrategy()
{
return new RegisterSessionAuthenticationStrategy(
buildSessionRegistry());
}
@Bean protected SessionRegistry buildSessionRegistry()
{
return new SessionRegistryImpl();
}
@Override
protected void configure(HttpSecurity http)
throws Exception
{
super.configure(http);
http.authorizeRequests()
.antMatchers("/customers")
.hasRole("USER")
.antMatchers("/admin")
.hasRole("ADMIN")
.anyRequest()
.permitAll();
}
}
To know more please refer to these articles:
20. What is the role of an AuthenticationProvider in Spring Security?
Authentication Providers perform the actual authentication work.
- AuthenticationManager is the API that describes that how Spring Security’s filters perform authentication.
- ProviderManager is the mostly used implementation of AuthenticationManager.
Java
@Override
public void configure(AuthenticationManagerBuilder auth)
throws Exception
{
auth.inMemoryAuthentication()
.withUser("admin")
.password("{noop} geeksforgeeks")
.authorities("USER");
}
Here, we are injecting the Authentication Provider that is in order to authenticate a user for the application using inMemoryAuthentication().
Know more about Spring Security – Authentication Provider
21. How to Secure an Endpoint in Spring Security?
In Spring Security, we can secure an endpoint by following below steps:
Step 1: Configure Security
We will create a configuration class that should extend WebSecurityConfigurerAdapter and override the configure() method. For example:
Java
@Configuration
@EnableWebSecurity
public class SecurityConfig
extends WebSecurityConfigurerAdapter {
@Override
private void configure(HttpSecurity http)
throws Exception
{
// complete code
}
@Override
private void
configure(AuthenticationManagerBuilder auth)
throws Exception
{
// complete as per requirement
}
}
Step 2: Secure Specific Endpoints
We can use annotations like @PreAuthorize, @Secured @RolesAllowed, etc. to secure specific method or classes. For example:
Java
@RestController
public class MyController {
@GetMapping("/securedEndpoint")
@Secured("ROLE_USER")
public String securedEndpoint()
{
//.....
}
}
Configure Method Security:
We can use the @EnableGlobalMethodSecurity annotation for method level security.
22. Explain UserDetailsService and UserDetails in Spring Security.
In Spring Security, the UserDetailsService is an interface used for loading user-specific data.
- This interface is responsible for retrieving user information from a backend data source, such as a database.
- This returns an instance of the UserDetails interface.
- It has a single method called loadUserByUsername(). It takes a username as a parameter, and it returns a UserDetails object.
- The UserDetails object represents the authenticated user, and it contains all the details such as the username, password, authorities (roles) etc.
Know more about Spring Security – UserDetailsService and UserDetails with Example
23. What is method-level security in Spring Security?
Method-Level security in Spring Security is done by using @PreAuthorize and @PostAuthorize annotations.
- By using these two annotations, we can easily secure the methods.
- We can ensure that only the authorized users can have access to them.
@PreAuthorize: It is used to secure a method before it is executed.
Java
@PreAuthorize("hasRole('ADMIN')")
public void deleteEmployee(Long id)
{
// code
}
Here, only users with Admin role can access the deleteEmployee() method.
@PostAuthorize: It is used to secure a method after it is executed.
Java
@PostAuthorize("hasRole('ADMIN')")
public List<Employee> getAllEmployees()
{
// code
}
Here, only users with Admin role can view the list of all employees.
Know more about Method-Level security in Spring Security
24. Difference between hasRole() and hasAuthority().
Features
| hasRole()
| hasAuthority()
|
---|
Working
| It is the shortcut for specifying URLs require for a particular role.
| It specifies that URLs require a particular authority.
|
Prefix
| Do not have to add role prefix (default “ROLE_”).
| Have to add role prefix (default “ROLE_”).
|
Syntax
| The role is required (i.e. ADMIN, USER etc.).
| The authority to require (i.e. ROLE_ADMIN, ROLE_USER, etc.)
|
Spring Security Interview Questions for Experienced
25. What is SessionManagement in Spring Security?
At the time of login, user will enter username and password. If that is valid a HTTP session is created servers. That session creation, managing, destroying everything is called Session Management.
- It secures and manages multiple user’s sessions against the requests.
- To control HTTP sessions, SessionManagementFilter and SessionAuthenticationStrategy are used.
- Authentication strategy takes care of session timeout, session ids etc.
- It is actually going to verify is that object is null, and it exists or not.
26. What is Diligatingfilterproxy in Spring Security?
DiligatingFilterProxy is a Servlet filter that intercepts all the incoming requests sent to an application.
- It allows bridging between the Servlet Container’s lifecycle and Spring’s ApplicationContext.
- The Servlet Container allows to registering Filters using its own standards.
- It can be even registered by using Servlet Container mechanism by using web.xml and we can then define a filter tag.
.png)
<filter>
<filter-name>springSecurityFilterChain</filter-name>
<filter-class>org.springframework.web.filter.DelegatingFilterProxy</filter-class>
</filter>
<filter-mapping>
<filter-name>springSecurityFilterChain</filter-name>
</filter-mapping>
27. How to implement Two-Factor Authentication (2FA) in Spring Security?
To implement two-factor authentication (2FA) follow the below steps:
- Step 1: Add spring-boot-starter-security dependency in the pom.xml file.
- Step 2: To configure Spring Security, extend WebSecurityConfigureAdapter (WSCA).
Java
@EnableWebSecurity
public class SecurityConfig
extends WebSecurityConfigurerAdapter
- Step 3: Override the configure method.
Java
@Override
protected void configure(HttpSecurity http) throws Exception
{
// write code here
}
- Step 4: Now implement two-factor authentication by extending AbstractAuthenticationProcessingFilter. (You may create a custom filter)
Java
public class TwoFactorAuthenticationFilter
extends AbstractAuthenticationProcessingFilter
- Step 5: Register the custom filter in Security Configuration.
Java
.addFilterBefore(
new TwoFactorAuthenticationFilter(),
UsernamePasswordAuthenticationFilter.class);
- Step 6: Based on the application requirements, enable two-factor authentication for specific users or groups.
Know more about Two-Factor Authentication in Spring Security
28. Explain Hashing in Spring Security.
Generally, end-users register the details at the same time provides password as well. That password we store in the database as it is that is called Plain text.
- Storing Plain text in the database is not recommended standard, so it should be converted to unreadable format that is called encrypting the password.
- Storing encrypted password in the database called Password Hashing.
- To convert it into unreadable format, there are so many hashing algorithms like Message Digester Algorithm (MD4, MD5), Security Hashing Algorithm – SHA (SHA256, SHA128) ETC.
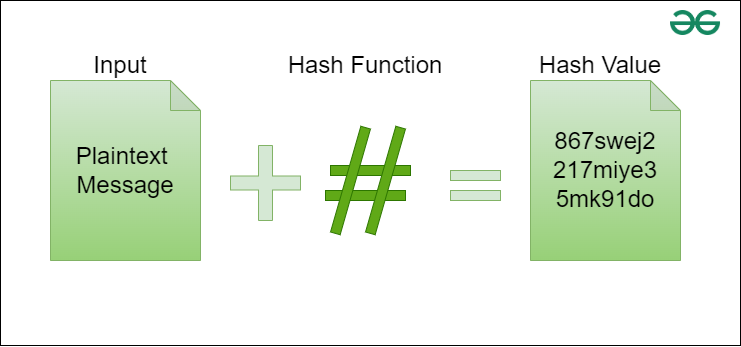
29. What is Spring Expression Language (SpEL) and Tell Some Spring Security Annotations that are involved with this?
SpEL (Spring Expression Language) is a powerful expression language and also this supports querying and manipulating an object graph at runtime. It can be used within Spring annotations, and it provides dynamic values based on runtime data. There are some Annotations that are involved with Spring Security
- @PreAuthorize
- @Secured
- @PostAuthorize
- @PostFilter
- @PreFilter
Know more about Spring Expression Language (SpEL)
Bonus Spring Security Questions and Answers
30. How Spring security handles user authentication?
Spring security handles user authentication by verifying and managing credentials, filters. Spring Security has UserDetailsService, this service takes the username and returns an object with the user details.
31. Explain potential web application vulnerabilities and how Spring Security mitigates them?
Spring Security protects against common web application vulnerabilities like:
- SQL injection
- Cross Site Scripting (XSS)
- Cross Site Request Forgery (XSRF)
Spring Security mitigates them through filters and Content Security Policy.
32. How to implement Spring Security with in-memory user storage.
To implement Spring Security with in-memory user storage, follow the below steps:
Step 1: Add Starter dependency in XML file.
spring-boot-starter-security
Step 2: In Spring Security configuration, enable in-memory authentication.
auth.inMemoryAthentication()
Know more about Spring Security – In-Memory Authentication
33. Explain @PreAuthorize and @PostAuthorize annotations.
- @PreAuthorize: It is used to secure a method before it is executed.
- @PostAuthorize: It is used to secure a method after it is executed.
Know more about Spring Security – Annotations
34. How to troubleshoot common Spring Security errors?
To troubleshoot common Spring security errors,
- Enable debug logging
- Check Dependencies
- Thoroughly check error messages
- Check roles and authorities
- Password encoding Verification
- Check Session Management
35. Explain Salting and its usage.
- Salting is a process in Spring Security to combine random data with a password before password hashing.
- By increasing its uniqueness and complexity, it improves Hashing.
Note: Salting is automatically applied since Spring Security version 3.1.
Conclusion
In conclusion, mastering Spring Security interview questions is essential for both beginners and seasoned professionals. These questions help you understand the framework and its importance in creating secure software. By exploring these topics, you not only prepare for interviews but also improve your knowledge of vital security principles.
Remember, learning Spring Security is an ongoing process, whether you’re new to the field or have years of experience. Embrace the challenge, enhance your skills, and face each interview with confidence. With persistence and practice, you can excel in Spring Security interviews at any career stage.
Spring Security Interview Questions – FAQs
What is the role of Spring Security in application development?
Spring Security provides authentication and authorization services for Java applications, ensuring secure access to resources.
What types of authentication does Spring Security support?
Spring Security supports a variety of authentication methods including form-based authentication, LDAP, basic authentication, OAuth2, and JWT among others.
What are the essential configurations required for Spring Security?
Essential configurations include setting up a SecurityContextHolder, AuthenticationManager, and configuring HttpSecurity to specify URL access rules and linked authentication and authorization requirements.
How can I prepare for a Spring Security interview?
Prepare by understanding core concepts of Spring Security, practicing with real-world scenarios, and familiarizing yourself with common problems and their solutions in Spring Security.
How can I stand out in a Spring Security interview?
Demonstrate a deep understanding of security principles, share experiences of solving complex security problems, and show your familiarity with the latest updates and best practices in Spring Security.
Share your thoughts in the comments
Please Login to comment...