Spring Security – Custom Form Login with Database Authentication
Last Updated :
17 Aug, 2023
In this article, Spring Security Basic Authentication, we have demonstrated the Basic Authentication using In-Memory Authentication. But what if we are required to authenticate the user from the database? And also what if we are required to login with the custom form? In this article, we will explain how to set up, configure, and customize Custom Form Login with Database Authentication in Spring. We’re going to build on top of the simple Spring MVC example and secure the UI of the MVC application with the Custom Form Login with Database Authentication.
Note: We are going to use the MySQL database for JDBC Authentication in this article.
Example Project
Step 1: Create Your Project and Configure Apache Tomcat Server
Note: We are going to use Spring Tool Suite 4 IDE for this project. Please refer to this article to install STS in your local machine How to Download and Install Spring Tool Suite (Spring Tools 4 for Eclipse) IDE.
Step 2: Create Schema and Tables in MySQL Workbench and Put Some Sample Data
Go to your MySQL Workbench and create a schema named gfgspringsecuritydemo and inside that create two tables users and authorities and put some sample data as shown in the below image.
Note: It is strictly recommended that you should create the tables as per the schema. The column name must be the same.
users Table:
Here is the users Table Schema
- username varchar_ignorecase(50) not null primary key,
- password varchar_ignorecase(50) not null,
- enabled boolean not null
Please refer to the below image for reference.
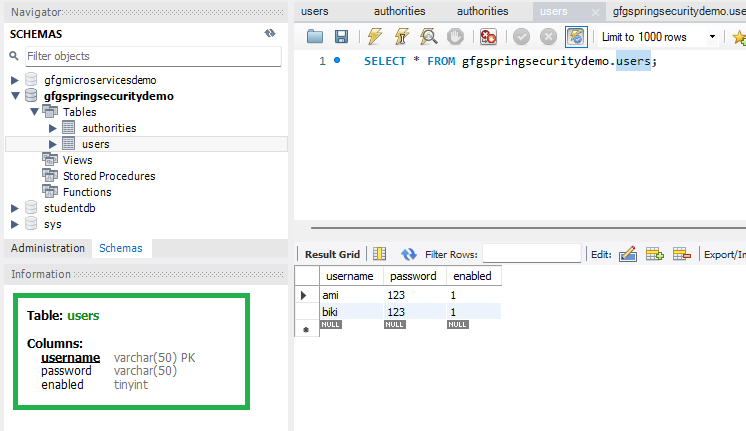
authorities Table:
Here is the authorities Table Schema
- username varchar_ignorecase(50) not null,
- authority varchar_ignorecase(50) not null,
- constraint fk_authorities_users foreign key(username) references users(username)
Please refer to the below image for reference.
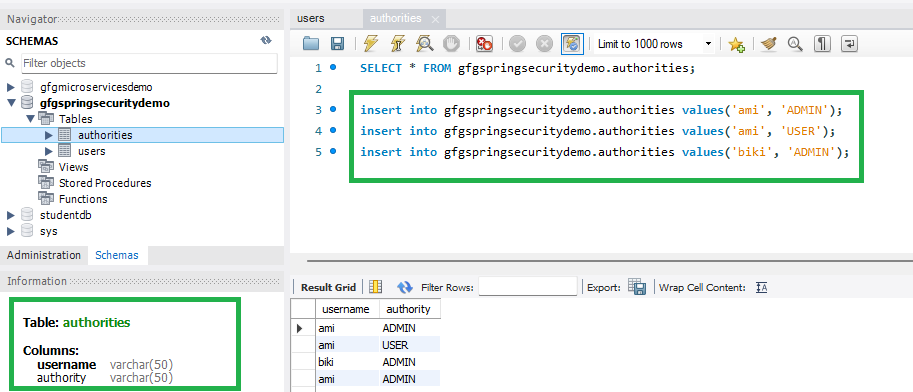
Step 3: Folder Structure
Before moving to the project let’s have a look at the complete project structure for our Spring MVC application.
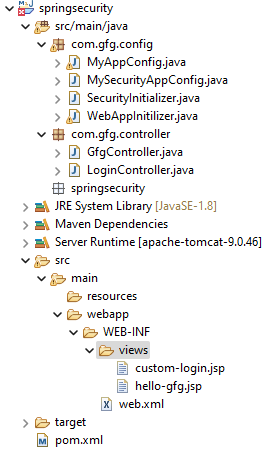
Step 4: Add Dependencies to pom.xml File
Add the following dependencies to your pom.xml file
- Spring Web MVC
- Java Servlet API
- Spring Security Config
- Spring Security Web
- Spring JDBC
- MySQL Connector Java
XML
< dependencies >
< dependency >
< groupId >org.springframework</ groupId >
< artifactId >spring-webmvc</ artifactId >
< version >5.3.24</ version >
</ dependency >
< dependency >
< groupId >javax.servlet</ groupId >
< artifactId >javax.servlet-api</ artifactId >
< version >4.0.1</ version >
< scope >provided</ scope >
</ dependency >
< dependency >
< groupId >org.springframework.security</ groupId >
< artifactId >spring-security-config</ artifactId >
< version >5.7.3</ version >
</ dependency >
< dependency >
< groupId >org.springframework.security</ groupId >
< artifactId >spring-security-web</ artifactId >
< version >5.7.3</ version >
</ dependency >
< dependency >
< groupId >org.springframework</ groupId >
< artifactId >spring-jdbc</ artifactId >
< version >5.3.24</ version >
</ dependency >
< dependency >
< groupId >mysql</ groupId >
< artifactId >mysql-connector-java</ artifactId >
< version >8.0.28</ version >
</ dependency >
</ dependencies >
|
Below is the complete pom.xml file. Please cross-verify if you have missed some dependencies.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< groupId >com.gfg.springsecurity</ groupId >
< artifactId >springsecurity</ artifactId >
< version >0.0.1-SNAPSHOT</ version >
< packaging >war</ packaging >
< name >springsecurity Maven Webapp</ name >
< properties >
< project.build.sourceEncoding >UTF-8</ project.build.sourceEncoding >
< maven.compiler.source >1.7</ maven.compiler.source >
< maven.compiler.target >1.7</ maven.compiler.target >
</ properties >
< dependencies >
< dependency >
< groupId >junit</ groupId >
< artifactId >junit</ artifactId >
< version >4.11</ version >
< scope >test</ scope >
</ dependency >
< dependency >
< groupId >org.springframework</ groupId >
< artifactId >spring-webmvc</ artifactId >
< version >5.3.24</ version >
</ dependency >
< dependency >
< groupId >javax.servlet</ groupId >
< artifactId >javax.servlet-api</ artifactId >
< version >4.0.1</ version >
< scope >provided</ scope >
</ dependency >
< dependency >
< groupId >org.springframework.security</ groupId >
< artifactId >spring-security-config</ artifactId >
< version >5.7.3</ version >
</ dependency >
< dependency >
< groupId >org.springframework.security</ groupId >
< artifactId >spring-security-web</ artifactId >
< version >5.7.3</ version >
</ dependency >
< dependency >
< groupId >org.springframework</ groupId >
< artifactId >spring-jdbc</ artifactId >
< version >5.3.24</ version >
</ dependency >
< dependency >
< groupId >mysql</ groupId >
< artifactId >mysql-connector-java</ artifactId >
< version >8.0.28</ version >
</ dependency >
</ dependencies >
< build >
< finalName >springsecurity</ finalName >
< pluginManagement >
< plugins >
< plugin >
< artifactId >maven-clean-plugin</ artifactId >
< version >3.1.0</ version >
</ plugin >
< plugin >
< artifactId >maven-resources-plugin</ artifactId >
< version >3.0.2</ version >
</ plugin >
< plugin >
< artifactId >maven-compiler-plugin</ artifactId >
< version >3.8.0</ version >
</ plugin >
< plugin >
< artifactId >maven-surefire-plugin</ artifactId >
< version >2.22.1</ version >
</ plugin >
< plugin >
< artifactId >maven-war-plugin</ artifactId >
< version >3.2.2</ version >
</ plugin >
< plugin >
< artifactId >maven-install-plugin</ artifactId >
< version >2.5.2</ version >
</ plugin >
< plugin >
< artifactId >maven-deploy-plugin</ artifactId >
< version >2.8.2</ version >
</ plugin >
</ plugins >
</ pluginManagement >
</ build >
</ project >
|
Step 5: Configuring Dispatcher Servlet
Please refer to this article What is Dispatcher Servlet in Spring? and read more about Dispatcher Servlet which is a very very important concept to understand. Now we are going to configure Dispatcher Servlet with our Spring MVC application.
Go to the src > main > java and create a class WebAppInitilizer. Below is the code for the WebAppInitilizer.java file.
File: WebAppInitilizer.java
Java
package com.gfg.config;
import org.springframework.web.servlet.support.AbstractAnnotationConfigDispatcherServletInitializer;
public class WebAppInitilizer extends
AbstractAnnotationConfigDispatcherServletInitializer {
@Override
protected Class<?>[] getRootConfigClasses() {
return null ;
}
@Override
protected Class<?>[] getServletConfigClasses() {
Class[] configFiles = {MyAppConfig. class };
return configFiles;
}
@Override
protected String[] getServletMappings() {
String[] mappings = { "/" };
return mappings;
}
}
|
Create another class in the same location (src > main > java) and name it MyAppConfig. Below is the code for the MyAppConfig.java file.
File: MyAppConfig.java
Java
package com.gfg.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
@Configuration
@EnableWebMvc
@ComponentScan ( "com" )
public class MyAppConfig {
}
|
Reference article: Spring – Configure Dispatcher Servlet in Three Different Ways
Step 6: Create Your Spring MVC Controller
Go to the src > main > java and create a class GfgController. Below is the code for the GfgController.java file.
File: GfgController.java
Java
package com.gfg.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class GfgController {
@GetMapping ( "/gfg" )
public String helloGfg() {
return "hello-gfg" ;
}
}
|
Go to the src > main > java and create a class LoginController. Below is the code for the LoginController.java file.
File: LoginController.java
Java
package com.gfg.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class LoginController {
@GetMapping ( "/customLogin" )
public String customLogin() {
return "custom-login" ;
}
}
|
Reference article: Create and Run Your First Spring MVC Controller in Eclipse/Spring Tool Suite
Step 7: Create Your Spring MVC View
Go to the src > main > webapp > WEB-INF > right-click > New > Folder and name the folder as views. Then views > right-click > New > JSP File and name your first view. Here we have named it as hello-gfg.jsp file. Below is the code for the hello-gfg.jsp file. We have created a simple web page inside that file.
File: hello-gfg.jsp
HTML
<!DOCTYPE html>
< html >
< body bgcolor = "green" >
< h1 >Hello GeeksforGeeks!</ h1 >
</ body >
</ html >
|
Also, create another view named custom-login.jsp file. Below is the code for the custom-login.jsp file. We have created a simple login form inside that file.
File: custom-login.jsp
HTML
<!DOCTYPE html>
< html >
< title >GFG Login Page</ title >
< body bgcolor = "green" >
< h1 >Custom Login Page</ h1 >
< form:form >
Username : < input type = "text" name = "username" >
< br />
Password : < input type = "password" name = "password" >
< br />
< input type = "submit" value = "Login" >
</ form:form >
</ body >
</ html >
|
Reference articles:
Step 8: Setting Up ViewResolver in Spring MVC
Go to the src > main > java > MyAppConfig and set your ViewResolver like this
File: MyAppConfig.java
Java
package com.gfg.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
@Configuration
@EnableWebMvc
@ComponentScan ( "com" )
public class MyAppConfig {
@Bean
InternalResourceViewResolver viewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver.setPrefix( "/WEB-INF/views/" );
viewResolver.setSuffix( ".jsp" );
return viewResolver;
}
}
|
Reference article: ViewResolver in Spring MVC
Step 9: Setting Up Spring Security Filter Chain
Go to the src > main > java and create a class MySecurityAppConfig and annotate the class with @EnableWebSecurity annotation. This class will help to create the spring security filter chain. Below is the code for the MySecurityAppConfig.java file.
File: MySecurityAppConfig.java
Java
package com.gfg.config;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
@EnableWebSecurity
public class MySecurityAppConfig extends WebSecurityConfigurerAdapter {
}
|
Step 10: Create Spring Security Initilizer
Go to the src > main > java and create a class SecurityInitializer. This class will help to register the spring security filter chain with our application. Below is the code for the SecurityInitializer.java file.
File: SecurityInitializer.java
Java
package com.gfg.config;
import org.springframework.security.web.context.AbstractSecurityWebApplicationInitializer;
public class SecurityInitializer extends AbstractSecurityWebApplicationInitializer {
}
|
Now we are done with setting up our Spring Security Filter Chain.
Step 11: JDBC Authentication Implementation
Modify the MyAppConfig file. Here we are going to create the DataSource Bean. That means we are going to write the code for MySQL Database connection.
// Connect to MySQL Database
@Bean
DataSource dataSource() {
DriverManagerDataSource driverManagerDataSource = new DriverManagerDataSource();
driverManagerDataSource.setUrl("jdbc:mysql://localhost:3306/gfgspringsecuritydemo");
driverManagerDataSource.setUsername("root");
driverManagerDataSource.setPassword("143@Arpilu");
driverManagerDataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
return driverManagerDataSource;
}
File: MyAppConfig.java
Java
package com.gfg.config;
import javax.sql.DataSource;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
@Configuration
@EnableWebMvc
@ComponentScan ( "com" )
public class MyAppConfig {
@Bean
InternalResourceViewResolver viewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver.setPrefix( "/WEB-INF/views/" );
viewResolver.setSuffix( ".jsp" );
return viewResolver;
}
@Bean
DataSource dataSource() {
DriverManagerDataSource driverManagerDataSource = new DriverManagerDataSource();
driverManagerDataSource.setUsername( "root" );
driverManagerDataSource.setPassword( "143@Arpilu" );
driverManagerDataSource.setDriverClassName( "com.mysql.cj.jdbc.Driver" );
return driverManagerDataSource;
}
}
|
Modify the MySecurityAppConfig file. Here we are going to implement the JDBC Authentication by overriding the configure() method.
File: MySecurityAppConfig.java
Java
package com.gfg.config;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.password.NoOpPasswordEncoder;
@SuppressWarnings ( "deprecation" )
@EnableWebSecurity
public class MySecurityAppConfig extends WebSecurityConfigurerAdapter {
@Autowired
private DataSource datasource;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.jdbcAuthentication().dataSource(datasource).passwordEncoder(NoOpPasswordEncoder.getInstance());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests()
.antMatchers( "/gfg" ).authenticated()
.and()
.formLogin().loginPage( "/customLogin" )
.and()
.httpBasic();
}
}
|
Now, let’s run the application and test it out.
Step 12: Run Your Spring MVC Application
To run our Spring MVC Application right-click on your project > Run As > Run on Server. After that use the following URL to run your controller.
http://localhost:8080/springsecurity/gfg
And it will ask for authentication to use the endpoint and a pop-up screen will be shown like this. But this time it’s our custom login page.
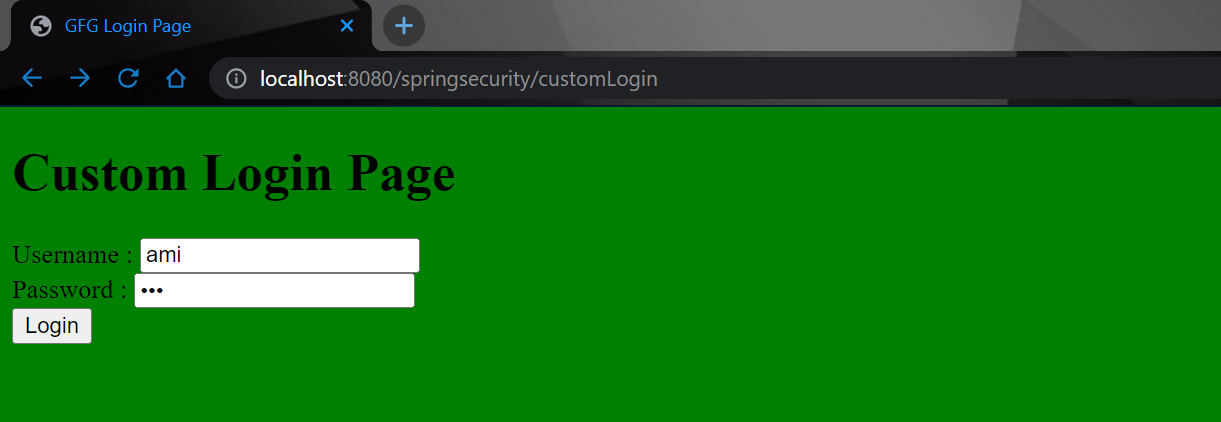
Now sign in with your database credentials
- Username: ami
- Password: 123
And now you can access your endpoint.
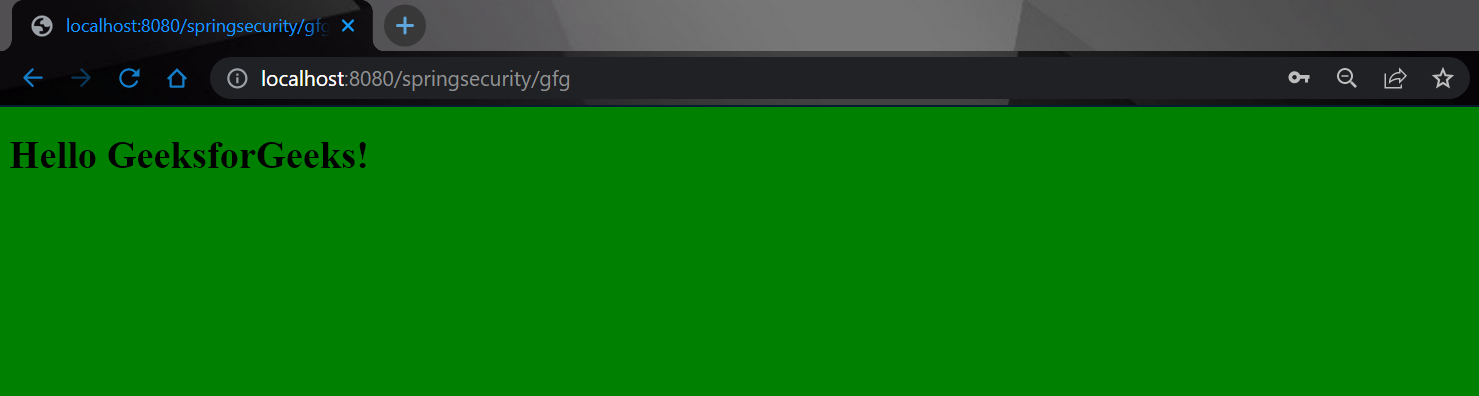
Share your thoughts in the comments
Please Login to comment...