Spring MVC Optional @PathVariable in Request URI
Last Updated :
08 Dec, 2023
Spring MVC becomes even more easy with the use of the @PathVariable
annotation and by the use of Optional classes within the Request URI to make robust Spring Applications.
In this article, we will look into the necessity of the two components, delving into their prerequisites and understanding why Optionals are essential in handling scenarios where data might be absent with practical implementation and the benefits they offer while handling potential null pointer exceptions.
- Optionals with path variables contribute to cleaner, error-resilient code in Spring MVC.
Prerequisites
Why do we need to use Optional classes with @PathVariable Annotation?
Suppose the user is accessing a value in the database/collection that does not exist and the primary key of the data is passed in the template variable and assigned to a variable, then we can use Optionals to check if there exists a value at that place or not.
- Using Optionals helps us handle the null pointer exceptions and also makes the code readable.
To better understanding, let us look at a code example explained below:
Java
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.Arrays;
import java.util.List;
@RestController
@RequestMapping ( "/index" )
public class MyControllerPathVariable {
private static List<String> fruits= null ;
@GetMapping ( "/{fruit}" )
public static String publicMessage(
@PathVariable (required = true ) int fruit
){
return fruits.get(fruit);
}
}
|
- In the above code, suppose that our database is the list of fruits that is currently set as null.
- However when we as the user try to visit the URL at http://localhost:8080/index/5,
- we get an error message of 404 – Not found.
- This is because, we as the users of a website are unaware that the database of fruits is currently empty.
The backend code logs the following ugly error on the console:-
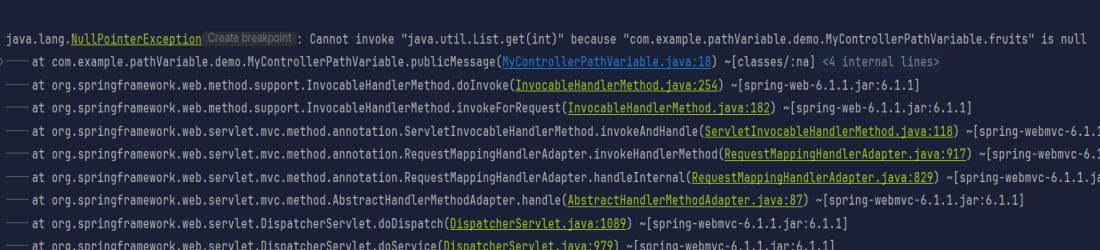
Null Pointer Exception
To avoid this situation, we can make the use of Optionals in our code that can help us to handle the exceptions on the backend.
To make use of Optionals, make the following changes are required to your code:
Java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
import java.util.Optional;
@RestController
@RequestMapping ( "/index" )
public class MyControllerPathVariable {
private static Optional<List<String>> fruits= Optional.ofNullable( null );
@GetMapping ( "/{fruit}" )
public static String publicMessage(
@PathVariable (required = true ) int fruit
){
if (fruits.isPresent()) {
return fruits.get().get(fruit);
}
else {
return "No fruits found." ;
}
}
}
|
- In the above code, suppose that our database is the list of fruits that is currently set as null using Optionals.
- when we as the user try to visit the URL at http://localhost:8080/index/5,
- we get an error message of 404 – Not found.
- This is because, we as the users of a website are unaware that the database of fruits is currently empty.
Now when we execute the code and try to access the fruits list in our browser, we get the following message:
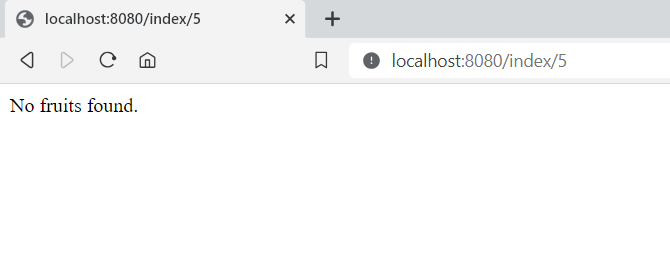
Using Optionals
And the backend too, does not run into any unintended errors:-
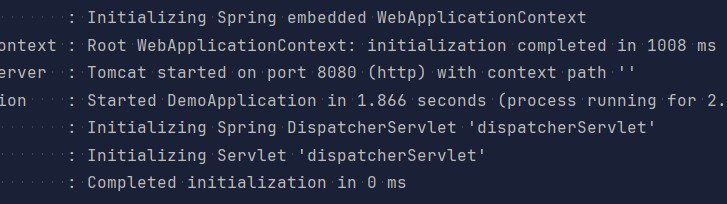
No unwanted exceptions
Conclusion
Thus using Optionals, we can write clean code. However, please note that the use of Optionals must be limited to cases where there could be many nulls.
Note: Unnecessary use of Optionals for small code samples will lead to unintended memory overheads and can slow down the running of the code.
Thus, we have seen why do we need Optionals with path variables, how to use them and the limitations of their use.
Share your thoughts in the comments
Please Login to comment...