Spring MVC – Download File Controller
Last Updated :
05 Jan, 2024
The Spring MVC is an approach for designing and developing the web application in the MVC pattern. In this article, we will learn how to develop logic for downloading a file from the server or file location in the Spring Boot with Spring Controller. For the application, we have used one HTML page, Spring Boot with MVC Pattern. We will explain from scratch how to develop this logic in Spring Boot with Spring MVC pattern.
Prerequisites
To understand this application, we need basic knowledge of the below technologies:
- Spring Boot
- Thymeleaf
- Spring MVC
- Bootstrap Framework
Here we have used Spring Boot for developing the main logic for file download and Bootstrap Framework is used for creating a good web interface for downloading the file.
Project Steps
- Step 1: Create a Spring Stater Project using your favorite IDE (Reference)
- Step 2: In the main package, create one Java class for the Download File Controller
- Step 3: After that create one HTML file in Templates which is located in the Resource folder of the same folder.
- Step 4: After developing the logic, run this project as a Spring boot App.
- Step 5: After that open your browser then type this http://localhost:8080/
- Step 6: After that, you have one HTML interface on that page one button is there click on it.
Then file will be downloaded into your system.
Project Folder Structure
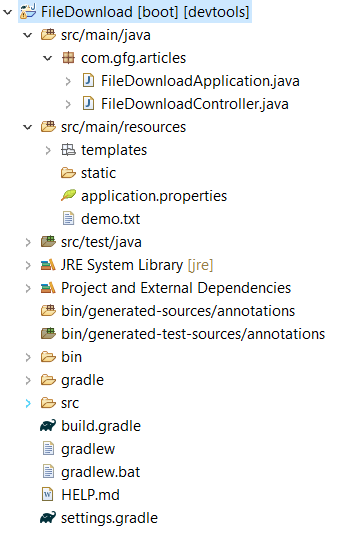
Now we will discuss the main logic for downloading the file with Spring Boot with Controller layer. After that we will see the testing with images in the below.
Download File Controller:
Java
package com.gfg.articles;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.Resource;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import jakarta.servlet.http.HttpServletResponse;
@Controller
public class FileDownloadController {
@GetMapping ( "/download" )
public void downloadFile(HttpServletResponse response) {
try {
Resource resource = new ClassPathResource( "demo.txt" );
response.setContentType( "text/plain" );
response.setHeader( "Content-Disposition" , "attachment; filename=" + resource.getFilename());
InputStream inputStream = resource.getInputStream();
OutputStream outputStream = response.getOutputStream();
byte [] buffer = new byte [ 1024 ];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != - 1 ) {
outputStream.write(buffer, 0 , bytesRead);
}
inputStream.close();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
|
In the above code, first we have created one GET mapping for download the file, after that we provided the path of the downloaded file from the location or file server. Once we got file location then we will set content type as text. We have written this entire logic within try catch block. If file is not existed, then it throws an exception otherwise File is downloaded in your local System. Here we have used HttpServletResponse for getting response from the API Hit point.
HTML Content:
HTML
<!DOCTYPE html>
< head >
< meta charset = "UTF-8" >
< title >File Download Example</ title >
</ head >
< body class = "container mt-5" >
< h2 class = "mb-4" >File Download Example</ h2 >
< a th:href = "@{/download}" class = "btn btn-success" >Download Sample File</ a >
</ body >
</ html >
|
Here we have created one HTML file. With that File we have created one anchor tag, by using Thymeleaf we hit the GET mapping API point. Then back-end logic is worked which is write in controller layer. Then file will be downloaded. Below we have provided the output images for better understanding.
Output:
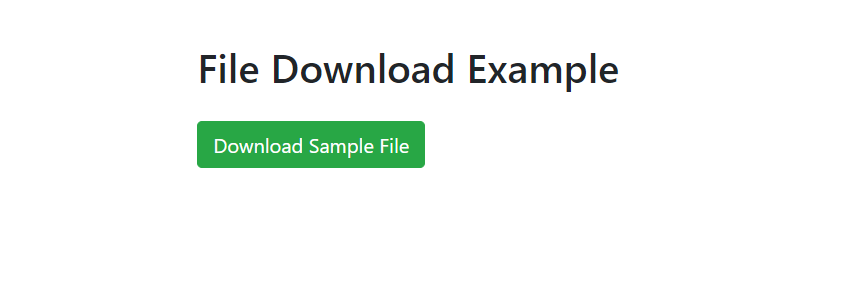
When you click on the Download Sample File Button. If the file exists in the given location, then the file will be downloaded, otherwise It will show exception.
Conclusion
File Download concept is widely used in every software application for gathering information from users. In this article, we have provided the basic example for how a file will be downloaded while click on given link or button. We developed this logic in controller layer. For understanding this example, you need basic knowledge on spring boot with Spring MVC pattern. In the Above example, we have mentioned the file location for as Resource you observe in the Controller java file.
Share your thoughts in the comments
Please Login to comment...