Session Management using express-session Module in Node.js
Last Updated :
28 Apr, 2020
Session management can be done in node.js by using the express-session module. It helps in saving the data in the key-value form. In this module, the session data is not saved in the cookie itself, just the session ID.
Installation of express-session module:
- You can visit the link Install express-session module. You can install this package by using this command.
npm install express-session
- After installing express-session you can check your express-session version in command prompt using the command.
npm version express-session
- After that, you can create a folder and add a file for example index.js, To run this file you need to run the following command.
node index.js
Filename: index.js
const express = require( "express" )
const session = require( 'express-session' )
const app = express()
var PORT = process.env.port || 3000
app.use(session({
secret: 'Your_Secret_Key' ,
resave: true ,
saveUninitialized: true
}))
app.get( "/" , function (req, res){
req.session.name = 'GeeksforGeeks'
return res.send( "Session Set" )
})
app.get( "/session" , function (req, res){
var name = req.session.name
return res.send(name)
})
app.listen(PORT, function (error){
if (error) throw error
console.log( "Server created Successfully on PORT :" , PORT)
})
|
Steps to run the program:
- The project structure will look like this:
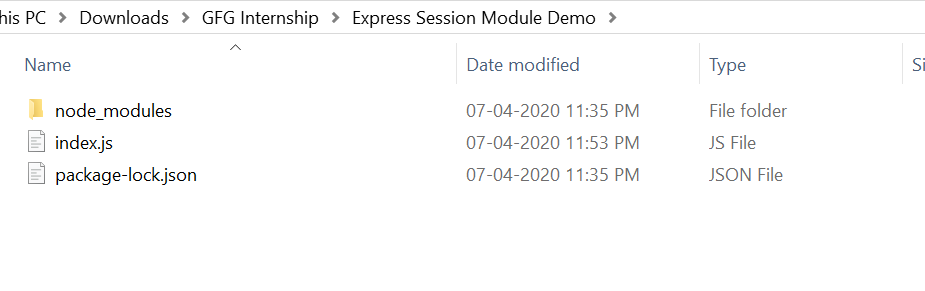
- Make sure you have install express and express-session module using following commands:
npm install express
npm install express-session
- Run index.js file using below command:
node index.js
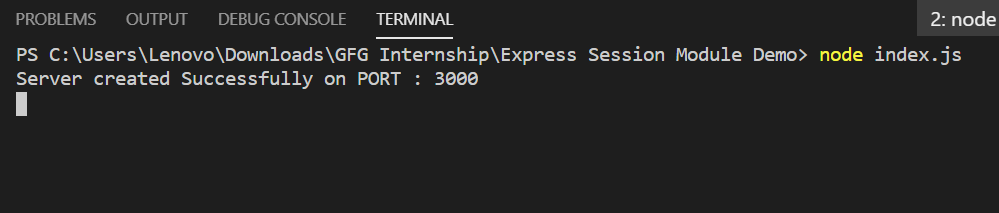
- Now to set your session, just open browser and type this URL:
http://localhost:3000/
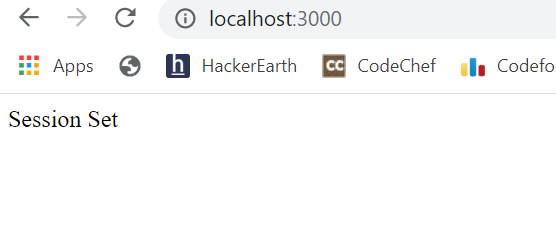
- Till now, you have set session and to see session value, type this URL:
http://localhost:3000/session
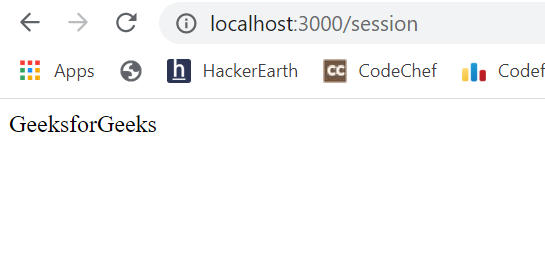
So this is how you can do session management in node.js using the express-session module.
Share your thoughts in the comments
Please Login to comment...