session._get_current_object() in Flask
Last Updated :
16 Mar, 2023
The session._get_current_object() method returns a session object for the current request that is made. It stores the information on the client’s computer as it works on the client side.
The exact object returned by the session._get_current_object() method completely depends on the implementation of the session so in the flask the method returns an instance of the ‘secureCookieSession’ class which is a subclass of the ‘dict’ which is a python built-in type.
Let’s understand session._get_current_object() :
- In Python, a “session” generally denotes a method of preserving user-specific data across multiple HTTP requests. Sessions are frequently used in web applications to track user authentication status, the contents of shopping carts, and other user-specific information.
- In Python web frameworks like Flask and Django, session management is often handled through a combination of server-side storage (e.g., in-memory data structures or a database) and client-side storage (e.g., cookies).
- For instance, the flask. session object in Flask is used to maintain sessions. A dictionary-like interface, (which is a python inbuilt type) is provided by the session object for storing and retrieving user-specific information. When a user submits a request to the server, Flask instantly accesses the user’s session data and makes it available to the application code (using the session ID saved in a cookie or in the request URL).
Using session._get_current_object()
Example:
Python3
from flask import Flask, session
app = Flask(__name__)
app.secret_key = "secret_key"
@app .route( '/set_session' )
def set_session():
current_session = session._get_current_object()
current_session[ 'username' ] = 'GeekforGeeks'
current_session[ 'logged_in' ] = True
return 'Session variables set'
@app .route( '/get_session' )
def get_session():
current_session = session._get_current_object()
username = current_session.get( 'username' , 'Guest' )
logged_in = current_session.get( 'logged_in' , False )
return f 'Username: {username}, Logged in: {logged_in}'
if __name__ = = '__main__' :
app.run(debug = True )
|
Now all done to run flask project type in command:
python <file-name>.py
Now navigate to the /set_session route, the session variables username and logged_in will be set in the session dictionary and the output screen will be like:
Output:
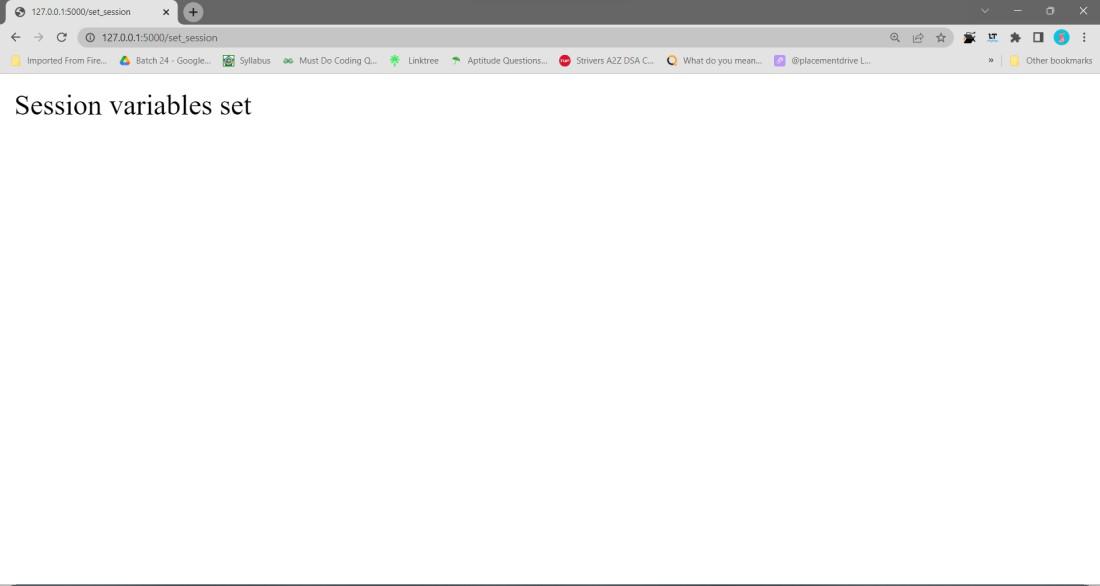
Setting the variables in session
- Assuming we make a GET request to /set_session first and then to /get_session, the output of the code will be:
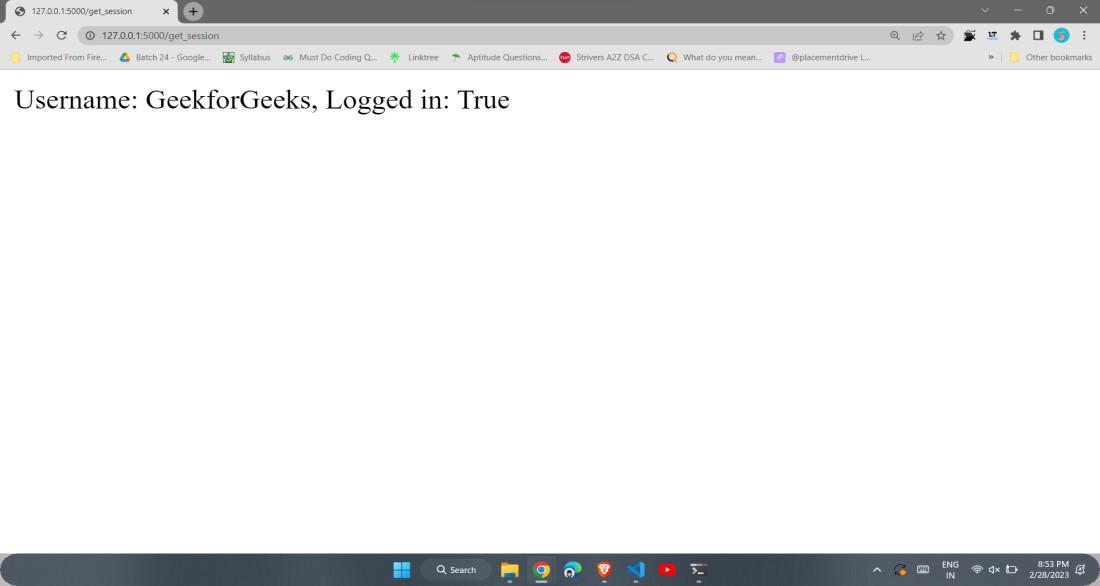
output of the code
- This is because the set_session route sets the username and logged_in variables in the session dictionary, and the get_session route retrieves those values and displays them in the response.
This output indicates that the session dictionary now contains two keys, ‘username’ and ‘logged_in’, with the value of ‘logged_in’ set to True.
In the output generated, we can see that session. get current object() doesn’t really return a dictionary-type result. It is an instance of the Python dict type, which was actually covered, as it’s a subclass of the SecureCookieSession class.
A SecureCookieSession object can behave like a dictionary in many ways, that way you can still access the data stored in the session object as if it were a dictionary by using the familiar dictionary syntax.
Share your thoughts in the comments
Please Login to comment...