Servlet – Hits Counter
Last Updated :
30 Jan, 2022
Sometimes, you’ll want to know the overall number of visitors to a specific page on your website. Because the life cycle of a servlet is governed by the container in which it runs, counting these hits with a servlet is fairly straightforward. The following steps are based on the implementation of a basic Servlet life cycle page hit counter:
- In the init() function, create a global variable.
- Every time the doGet() or doPost() methods are used, the global variable is increased.
- You may utilize a database table to save the value of a global variable in the destroy() method if necessary. When the servlet is next initialized, this value may be read from the init() function. This step is optional.
- If you want to count just unique page hits within a session then you may use the isNew() function to verify if the same page already has been hit within that session. This is an optional step.
- The value of the global counter may be shown to represent the overall number of hits on your website. This is also an optional step.
We’ll presume that the Web container can’t be restarted in this case. The counter will be reset if you restart or if the Servlet is deleted.
Example
This example demonstrates how to create a simple page hit counter.
Java
import java.io.*;
import java.sql.Date;
import java.util.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class GfgServletExample extends HttpServlet {
private int hitCount;
public void init()
{
hitCount = 0 ;
}
public void doGet(HttpServletRequest request,
HttpServletResponse response)
throws ServletException, IOException
{
response.setContentType( "text/html" );
hitCount++;
PrintWriter out = response.getWriter();
String title = "Count of total number of hits" ;
String docType
= "<!doctype html public \"-//w3c//dtd html 4.0 "
+ "transitional//en\">\n" ;
out.println(
docType + "<html>\n"
+ "<head><title>" + title + "</title></head>\n"
+ "<body bgcolor = \"#f0f0f0\">\n"
+ "<h1 align = \"center\">" + title + "</h1>\n"
+ "<h2 align = \"center\">" + hitCount
+ "</h2>\n"
+ "</body>"
+ "</html>" );
}
public void destroy()
{
}
}
|
Now compile the Servlet above and add the following item to the web.xml file:
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< web-app >
< display-name >HitCounter</ display-name >
< servlet >
< servlet-name >GfgServletExample</ servlet-name >
< servlet-class >GfgServletExample</ servlet-class >
</ servlet >
< servlet-mapping >
< servlet-name >GfgServletExample</ servlet-name >
< url-pattern >/GfgServletExample</ url-pattern >
</ servlet-mapping >
</ web-app >
|
Now by visiting http: to call the Servlet localhost:8080/HitCounter/GfgServletExample. This will refresh the page every time, to increase the value of counter 1, the result is as follows:
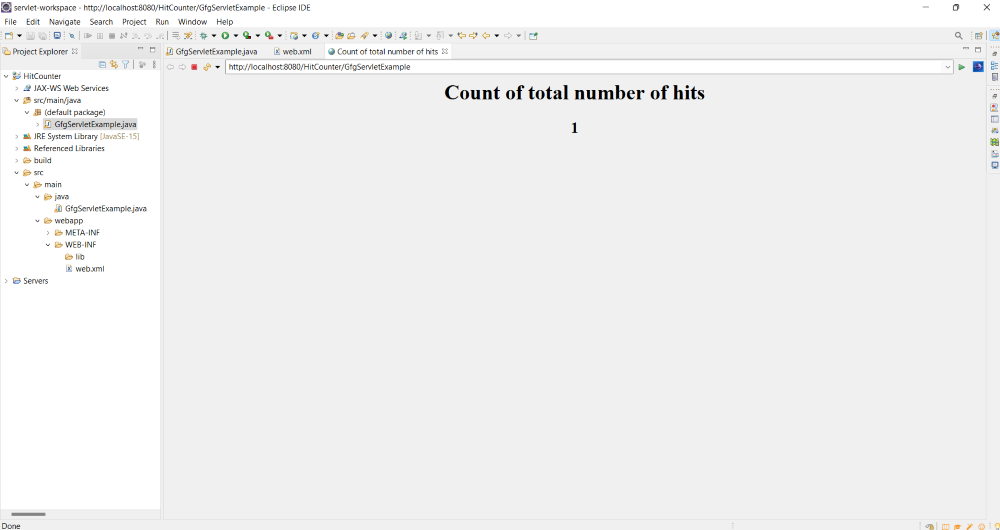
Website Hit Counter
You may be interested in knowing the overall number of hits on your entire website on many occasions. In Servlet, this is also quite straightforward, and we can do it by using filters. As a result, the methods to create a basic website hit counter based on the Filter Life Cycle are outlined.
- In the init() method of a filter, set a global variable.
- Every time the doFilter method is run, the global variable is increased.
- You may utilize a database table to save the value of a global variable in the filter’s destroy() function if necessary. When the filter is initialized the following time, this value may be read from the init() function. This is an optional step.
We are assuming that the web container will not be restarted at this point. The hit counter will be reset if it is restarted or if the servlet is destroyed.
Java
import java.io.*;
import java.util.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class GfgServletExample implements Filter {
private int hitCount;
public void init(FilterConfig config)
throws ServletException
{
hitCount = 0 ;
}
public void doFilter(ServletRequest request,
ServletResponse response,
FilterChain chain)
throws java.io.IOException, ServletException
{
hitCount++;
System.out.println( "Visits count is :" + hitCount);
chain.doFilter(request, response);
}
public void destroy()
{
}
}
|
Now compile the Servlet above and add the following item to the web.xml file:
XML
...
< filter >
< filter-name >GfgServletExample</ filter-name >
< filter-class >GfgServletExample</ filter-class >
</ filter >
< filter-mapping >
< filter-name >GfgServletExample</ filter-name >
< url-pattern >/GfgServletExample</ url-pattern >
</ filter-mapping >
...
|
Now by visiting http: to call the Servlet localhost:8080/HitCounter/GfgServletExample. This will refresh the page every time, to increase the value of counter 1, the result is as follows:
Visits count is : 1
Visits count is : 2
Visits count is : 3
Visits count is : 4
Visits count is : 5
...........
Share your thoughts in the comments
Please Login to comment...