Self-Referential Classes in C++
Last Updated :
14 Mar, 2022
A class is a building block in C++ that leads to Object-Oriented programming. It is a user-defined type that holds its own data members and member functions. These can be accessed by creating an instance of the type class.
Self-referential classes are a special type of classes created specifically for a Linked List and tree-based implementation in C++. To create a self-referential class, declare a data member as a pointer to an object of the same class.
Syntax:
class Self
{
private:
int a;
Self *srefer;
}
Below is the C++ program to implement self-referential classes.
C++
#include<iostream>
using namespace std;
class Self
{
public :
int x;
Self *srefer;
Self( int a):srefer(nullptr),x(a){}
};
void print(Self *b)
{
if (b == nullptr)
return ;
do
{
cout << b->x << endl;
} while ((b = b->srefer));
}
int main()
{
Self x(5), y(7), z(9);
x.srefer = &y;
y.srefer = &z;
print(&x);
return 0;
}
|
Key Points:
- Many frequently used dynamic data structures like stacks, queues, linked lists, etc.. use self-referential members.
- Classes can contain one or more members which are pointers to other objects of the same class.
- this pointer holds an address of the next object in a data structure.
The pictorial representation of a linked list having a pointer to the next object of the same class is shown below-
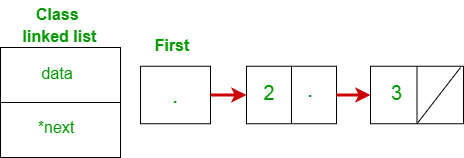
Example: Below is the C++ program to implement a linked list using a class. The class must contain a pointer member which points to the next node in the list.
C++
#include<iostream>
using namespace std;
class list
{
private :
int data;
list *next;
public :
list()
{
data = 0;
next = NULL;
}
list( int dat)
{
data = dat;
next = NULL;
}
~list();
int get()
{
return data;
}
void insert(list *node);
friend void display(list *);
};
void list::insert(list *node)
{
list *last = this ;
while (last->next)
last = last->next;
last->next = node;
}
void display(list *first)
{
list *traverse;
cout << "Elements of List are:" ;
cout << endl;
for (traverse = first; traverse;
traverse = traverse->next)
cout << traverse->data << " " ;
cout << endl;
}
int main()
{
list *first = NULL;
list *node;
for ( int i = 1; i < 5; i++)
{
node = new list(i);
if (first == NULL)
first = node;
else
first->insert(node);
}
display(first);
}
|
Output
Elements of List are:
1 2 3 4
Share your thoughts in the comments
Please Login to comment...