Scroll to the top of the page using JavaScript/jQuery
Last Updated :
07 Dec, 2023
A scrollable page can be scrolled to the top using 2 approaches:Â
The scrollTo() method of the window Interface can be used to scroll to a specified location on the page. It accepts 2 parameters the x and y coordinate of the page to scroll to. Passing both the parameters as 0 will scroll the page to the topmost and leftmost points.Â
Syntax:
window.scrollTo(x-coordinate, y-coordinate)
Example: In this example, we have used window.scrollTo() method.
html
<!DOCTYPE html>
< html >
< head >
< title >
Scroll to the top of the
page using JavaScript/jQuery?
</ title >
< style >
.scroll {
height: 1000px;
background-color: lightgreen;
}
</ style >
</ head >
< body >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< b >Scroll to the top of the page
using JavaScript/jQuery?</ b >
< p >Click on the button below to
scroll to the top of the page.</ p >
< p class = "scroll" >GeeksforGeeks is a
computer science portal. This is a
large scrollable area.</ p >
< button onclick = "scrollToTop()" >
Click to scroll to top
</ button >
< script >
function scrollToTop() {
window.scrollTo(0, 0);
}
</ script >
</ body >
</ html >
|
Output:
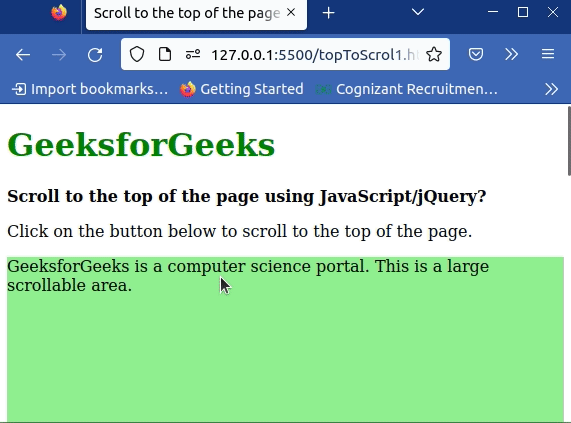
In jQuery, the scrollTo() method is used to set or return the vertical scrollbar position for a selected element. This behavior can be used to scroll to the top of the page by applying this method on the window property. Setting the position parameter to 0 scrolls the page to the top.Â
Syntax:
$(window).scrollTop(position);
Example:Â In this example, e have used scrollTop() method.
html
<!DOCTYPE html>
< html >
< head >
< title >
Scroll to the top of the
page using JavaScript/jQuery?
</ title >
< style >
.scroll {
height: 1000px;
background-color: lightgreen;
}
</ style >
</ head >
< body >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< b >
Scroll to the top of the page
using JavaScript/jQuery?
</ b >
< p >
Click on the button below to
scroll to the top of the page.
</ p >
< p class = "scroll" >
GeeksforGeeks is a computer
science portal.
This is a large scrollable area.
</ p >
< button onclick = "scrollToTop()" >
Click to scroll to top
</ button >
</ script >
< script >
function scrollToTop() {
$(window).scrollTop(0);
}
</ script >
</ body >
</ html >
|
Output:
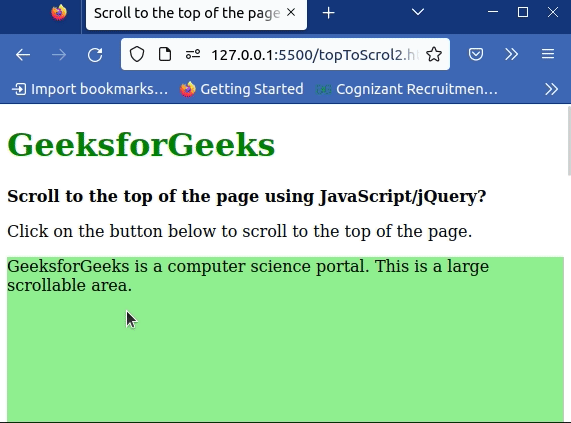
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples.
jQuery is an open-source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous for it’s philosophy of “Write less, do more”. You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...