Create an Infinite Scroll Page using HTML CSS & JavaScript
Last Updated :
19 Oct, 2023
In this article, we will create an infinite scroll page using HTML, CSS, and JavaScript. Infinite scrolling allows you to load and display content as the user scrolls down the page, providing a seamless browsing experience. We’ll fetch and append new content dynamically as the user reaches the end of the current content.
Preview Image:
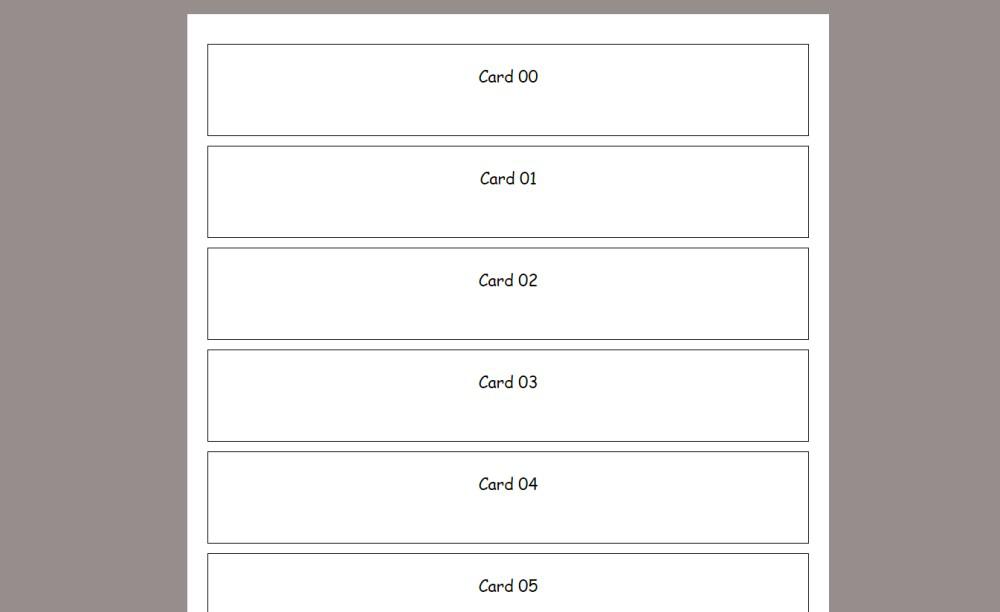
Output Preview
Approach:
We’ll build a basic web page with a container to display content and a loader indicator. JavaScript will handle fetching and appending new content when the user scrolls to the bottom of the page.
- When you open the HTML file in your web browser, you’ll immediately notice the initial content loading on the page. As you gracefully scroll down, the magic happens: new items seamlessly appear, dynamically loaded, and elegantly displayed. During this process, a loading indicator gracefully shows up, politely indicating that fresh data is being fetched.
- In essence, this code crafts an infinite scroll page where new content materializes as you gracefully navigate downward. The charm lies in its adaptability – you can effortlessly tailor both the content and style to harmonize with your project’s unique requirements.
- This practical example serves as a foundational introduction to crafting an infinite scroll page using HTML, CSS, and JavaScript. From this solid foundation, you have the creative liberty to refine it further, perhaps integrating real data from an API or refining the visual aesthetics to harmonize seamlessly with your project’s vision.
Project Structure:
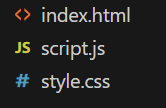
Project Structure
Example: Below is the basic implementation of the above project.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport"
content-after-loading=" width = device -width,
initial-scale = 1 .0" />
< link rel = "stylesheet" href = "style.css" />
< title >
Create an Infinite Scroll Page
using HTML CSS & JavaScript
</ title >
</ head >
< body >
< div id = "content-before-loading" ></ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
body {
font-family : cursive ;
margin : 0 ;
padding : 0 ;
background-color : #968d8d ;
}
.sub- block {
border : 1px solid #030303 ;
padding : 20px ;
margin : 10px 0 ;
height : 50px ;
text-align : center ;
}
#content-before-loading {
text-align : center ;
display : none ;
font-size : 16px ;
margin-top : 20px ;
max-width : 600px ;
margin : 20px auto ;
padding : 20px ;
background-color : #fff ;
}
|
Javascript
const before_loading =
document.getElementById( "content-before-loading" );
before_loading.style.display = "block" ;
let c = 0;
function getInformation() {
setTimeout(() => {
for (let i = 0; i < 10; i++) {
const new_div = document.createElement( "div" );
new_div.className = "sub-block" ;
new_div.innerHTML = `Card ${c}${i}`;
before_loading.appendChild(new_div);
}
c++;
}, 1000);
}
window.addEventListener( "scroll" , () => {
if (
document.documentElement.scrollTop +
document.documentElement.clientHeight >=
document.documentElement.scrollHeight
) {
getInformation();
}
});
getInformation();
|
Output:
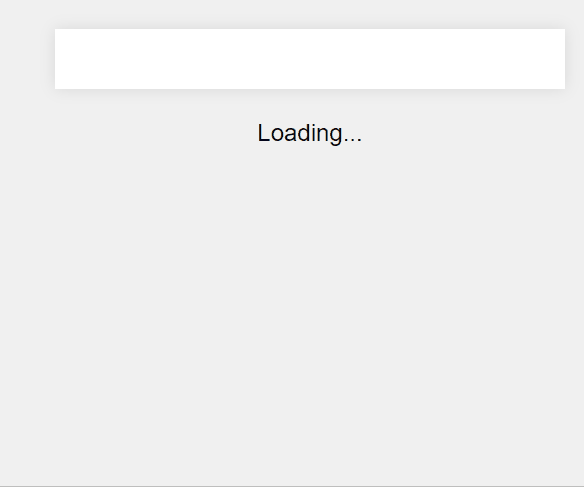
Output
Share your thoughts in the comments
Please Login to comment...