Rotate Image by 90 Degree using JavaScript
Last Updated :
26 Mar, 2024
In this article, we are going to learn how we can rotate images by 90 degrees clockwise using JavaScript. In simple words, we are given a matrix, and we have to rotate it by 90 degrees clockwise. To understand the question clearly, below is the image illustration explaining how the matrix looks when it is rotated 90 degrees clockwise:
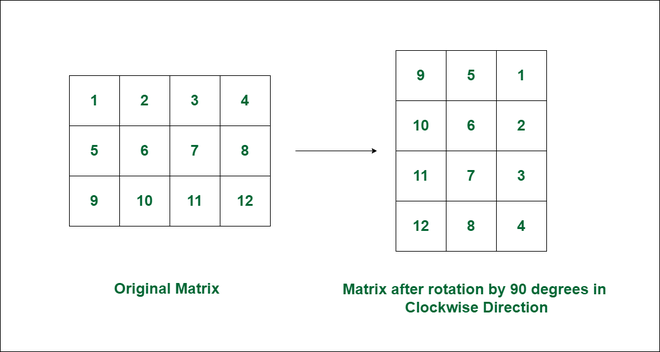
Brute force Approach
In this approach we are going to discuss how we can rotate image by 90 degree in clockwise direction by taking another matrix of same size. We will create another temporary matrix of same size of original matrix i.e. n*n and then we take the first row of original matrix and put it in the last column of the temporary matrix , take second row of original matrix and put it in the second last column of matrix , take third row of original matrix and put it in the third last column of matrix and so.
Example: To demonstrate rotation of image by 90 degree in clockwise direction by taking another matrix of same size by traversing the matrix using nested loop.
JavaScript
function rotate(matrix) {
let n = matrix.length;
let temp = new Array(n).fill().map(() => new Array(n).fill(0));
for (let i = 0; i < n; i++) {
for (let j = 0; j < n; j++) {
temp[j][n - i - 1] = matrix[i][j];
}
}
return temp;
}
function main() {
let arr = [
[1, 2, 3 ],
[4, 5, 6 ],
[7 , 8, 9]
];
let answer = rotate(arr);
console.log("Rotated image by 90 degree in clockwise direction ");
for (let i = 0; i < answer.length; i++) {
for (let j = 0; j < answer[0].length; j++) {
process.stdout.write(answer[i][j] + " ");
}
console.log();
}
}
main();
OutputRotated image by 90 degree in clockwise direction
7 4 1
8 5 2
9 6 3
Time Complexity: O(N*N) , we traverse original matrix.
Space Complexity: O(N*N) , created matrix of same size.
Using Optimal Approach (reduce Time Complexity)
In this approach we will reduce time complexity of problem from O(N*N) to O(1). If we observe the matrix , we can rotate it by 90 degree clockwise by two simple steps. First we will take transpose of matrix (change rows to columns and rows to columns) and then reverse each row of matrix. The matrix obtained is rotated matrix (by 90 degrees).
Example : To demonstrate rotation of image by 90 degree in clockwise direction by taking transpose of matrix and reversing its each row.
JavaScript
function rotateBy90Degree(matrix) {
const n = matrix.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < i; j++) {
[matrix[i][j],
matrix[j][i]] = [matrix[j][i],
matrix[i][j]];
}
}
for (let i = 0; i < n; i++) {
matrix[i].reverse();
}
}
function main() {
let arr = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
rotateBy90Degree(arr);
console.log("Rotated Image by 90 degree in clockwise direction ");
for (let i = 0; i < arr.length; i++) {
console.log(arr[i].join(" "));
}
}
main();
OutputRotated Image by 90 degree in clockwise direction
7 4 1
8 5 2
9 6 3
Time Complexity: O(N*N) , transposing the matrix + O(N*N) , reversing rows = O(N*N)
Space Complexity: O(1), constant space
Share your thoughts in the comments
Please Login to comment...