Request Body and Parameter Validation with Spring Boot
Last Updated :
03 Jan, 2024
For the Spring-Based RESTful Application, it is important to handle the incoming API requests and meet the expected format criteria. To handle this situation, we used @RequestBody, @RequestParam, and @Valid Annotation in the Spring Boot Application to validate the required format of the incoming requests. The Validation of Input increases the Security of the Spring Boot Application.
Spring Boot Request Body and Parameter Validation
The Request Body validation involves ensuring that the data sent in the request body confirms the expected structure. On the other hand, Parameter validation focuses on validating Parameters passed in the URL of the API. In Spring Boot @Valid and @RequestBody Annotations are used for Request body validation and the @RequestParam or @PathVariable is used for parameter validation.
Prerequisites
Requirements
- Spring Tool Suite for creating Spring Boot Application
- MongoDB is used for data storage.
- Gradle (here we have used) is the type of project Category.
Development Process
- Create a Spring Boot Project
- While creating Spring Boot Project Select Required Dependencies
- After That in the main project package create one POJO class which is User, After That Create a UserRepo class, and after that create One User Rest controller class.
- Now Develop each class with the required logic.
- After That run this project as Spring Boot App
Database Connection
For Connection with MongoDB keep these properties in your application properties file which is located in the resources folder in the project folder.
spring.data.mongodb.host=localhost
spring.data.mongodb.port=27017
spring.data.mongodb.database=geeks
Dependencies
implementation 'org.springframework.boot:spring-boot-starter-data-mongodb'
implementation 'org.springframework.boot:spring-boot-starter-validation'
implementation 'org.springframework.boot:spring-boot-starter-web'
compileOnly 'org.projectlombok:lombok'
developmentOnly 'org.springframework.boot:spring-boot-devtools'
annotationProcessor 'org.projectlombok:lombok'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
These Dependencies are available in build.gradle file.
Now we will discuss each and every point mention in the above. And also, we have provided the Project Folder Structure also for better understanding the concept.
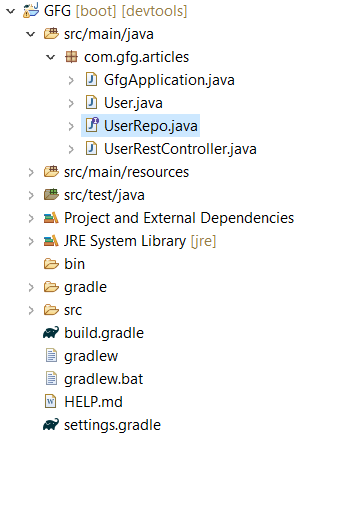
After Successful creation of Spring Boot Project, in main package we have created one User.java POJO for performing the operations on the Data. In this User POJO we take three attributes of user those ID, UserName, Age, for handling the Setters and Getters methods and default and Parameterized Constructor we have used lombok dependency which is available in Spring Boot.
User.java ( POJO Class)
Java
package com.gfg.articles;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
import jakarta.validation.constraints.NotEmpty;
import jakarta.validation.constraints.NotNull;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@AllArgsConstructor
@NoArgsConstructor
@Document (collection = "user" )
public class User {
@Id
private Integer id;
@NotEmpty (message = "user name is required" )
private String userName;
@NotNull (message = "user age is required" )
private Integer age;
}
|
In the Above code for username and age we have used @NotEmpty and @NotNull Annotation for data validation. While Testing the API. If you don’t provide any data in that fields, then we get errors.Means those attributes values are mandatory. After that create on repository class for handling database operation like insert data, retrieve data and other operation we can @Repository in the Repository class.
UserRepo.class ( Repository )
For Repository Creation we use @Repository Annotation in Spring Boot. We have used MongoDB that’s why this UserRepo Interface extends to MongoRepository and in this class we have passed two arguments name POJO class and it’s ID datatype. You can Observe in the Below code.
Java
package com.gfg.articles;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepo extends MongoRepository<User, Integer>{
}
|
In the above code we have created UserRepo Interface for perform operation on data by using MongoDB.
Now we will create one Rest Controller class to handle the incoming REST API requests by using @RestController Annotation in Spring Boot. In that class we will create one saveUser API for save the user details in the database. For this API, we have used POST Mapping as an endpoint.
UserRestController.java
Java
package com.gfg.articles;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import jakarta.validation.Valid;
import jakarta.validation.constraints.Positive;
import lombok.RequiredArgsConstructor;
@RestController
@RequestMapping ( "/api" )
@RequiredArgsConstructor
public class UserRestController {
@Autowired
private UserRepo userRepo;
@PostMapping ( "/user" )
public ResponseEntity<String> saveUser( @Valid @RequestBody User user)
{
userRepo.save(user);
return ResponseEntity.ok( "User details saved!" );
}
@GetMapping ( "/finduser/{id}" )
public ResponseEntity<String> findUserById( @PathVariable @Positive Integer id)
{
User foundUser = userRepo.findById(id).orElse( null );
if (foundUser != null ) {
return ResponseEntity.ok( "User exists!" );
} else {
return ResponseEntity.notFound().build();
}
}
}
|
In Above code, we have used @Valid, @RequestBody Annotation for validating data in the body. as well as we have used @PathVariable Annotation in findById API in the code for search user by using user id @Positive Annotation is used for Check the given User ID is positive or not.
If Given User ID is Negative value then it gives error message. And If user id not found it displays 404 error.
Note: For API Testing I use Postman Tool.
Output:
With Right Data
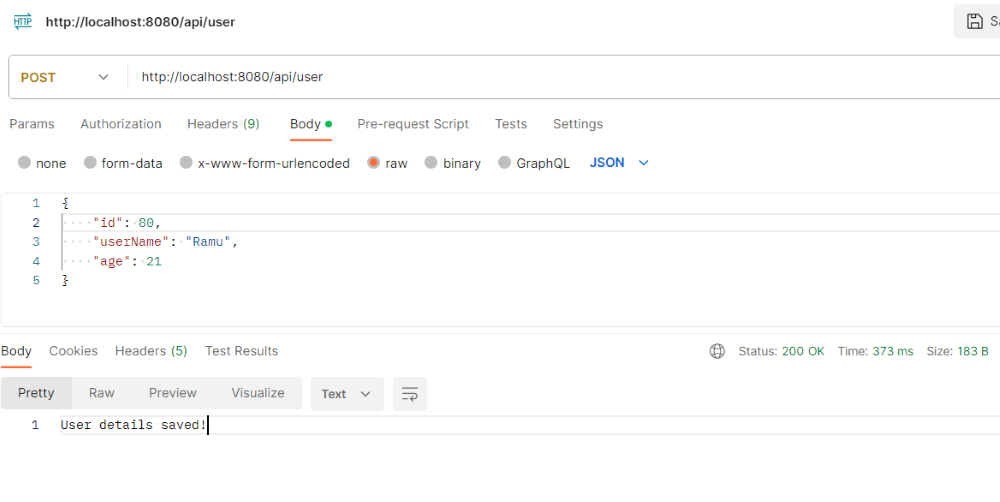
With missing data, we get Validation error message:
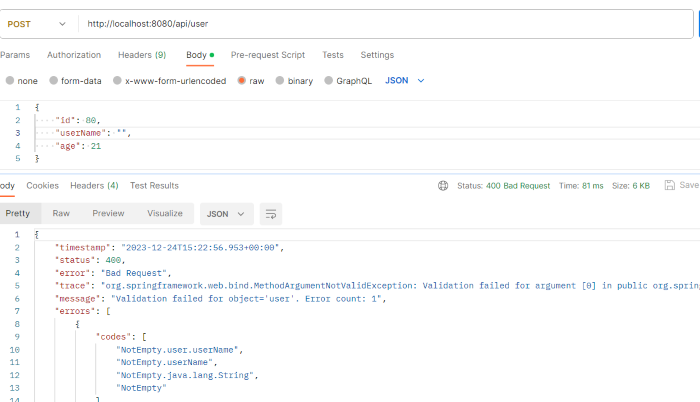
Conclusion
In Spring Boot Application, Request Body and Parameter Validation are used for validating the structure of data in the body as well as Validation the Parameter passed with in the URL of the API. These Validations plays an important role in Data Security. And The API’s Are not get Any response for Wrong format of the API URL.
Share your thoughts in the comments
Please Login to comment...