Python | URL shortener using tinyurl API
Last Updated :
09 Jan, 2023
There are multiple APIs (e.g-bitly API, etc.) available for URL shortening service but in this article, we will be using Tinyurl API to shorten URLs. Though tinyURL has not officially released its API but we are going to use it unofficially. Here, we can put as input any number of URLs at a time and get the shortened URLs at a time. For Example, if in command line we write
$ python filename URL1 URL2 URL3
then output will be:
['shortened url1', 'shortened url2', 'shortened url3']
Note:
The urllib2 library was deprecated in favor of the requests library due to the latter’s more advanced features and capabilities. In particular, the requests library natively supports HTTPS, has a more intuitive and flexible API, and includes functionality such as automatic retries and connection pooling, which are not available in urllib2. These features make the requests library more suitable for modern web development and more efficient for developers to use.
Step #1:
- First we have to import 7 libraries.
- We could have used just one library to work, but in order to make good url shortener we have to use 7 libraries.
The code snippet is as follows:
Python3
from __future__ import with_statement
import contextlib
try :
from urllib.parse import urlencode
except ImportError:
from urllib import urlencode
try :
from urllib.request import urlopen
except ImportError:
from urllib2 import urlopen
import sys
|
Step 2: Here encoding of url and appending it to API is done and then we open request_url using urlopen. Then we convert the response to UTF-8, since urlopen() returns a stream of bytes rather than a string. The code snippet is as follows:
Python3
def make_tiny(url):
with contextlib.closing(urlopen(request_url)) as response:
return response.read().decode( 'utf-8 ' )
|
Step 3: In the last step we are calling main() and we are getting user input by using sys.argv We have not limited ourself to only one url as input, instead, we are saying that give us as many urls as you want, we will make them tiny. map() is a simple way of looping over a list and passing it to a function one by one. The code snippet is as follows:
Python3
def main():
for tinyurl in map (make_tiny, sys.argv[ 1 :]):
print (tinyurl)
if __name__ = = '__main__' :
main()
|
Finally, the complete code is below:
Python3
from __future__ import with_statement
import contextlib
try :
from urllib.parse import urlencode
except ImportError:
from urllib import urlencode
try :
from urllib.request import urlopen
except ImportError:
from urllib2 import urlopen
import sys
def make_tiny(url):
with contextlib.closing(urlopen(request_url)) as response:
return response.read().decode( 'utf-8 ' )
def main():
for tinyurl in map (make_tiny, sys.argv[ 1 :]):
print (tinyurl)
if __name__ = = '__main__' :
main()
|
Output: 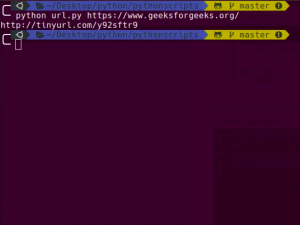
Share your thoughts in the comments
Please Login to comment...