Python – Starlette
Last Updated :
11 Dec, 2023
This article will delve into the Python web framework, Starlette, exploring its advantages and providing illustrative examples. Through these examples, we aim to enhance understanding and comprehension of Starlette’s capabilities and features.
What is Starlette?
Starlette has emerged as a notable addition to the Python web framework landscape, rapidly gaining favor for its simplicity, performance, and seamless integration with asynchronous programming. Created by Tom Christie, known for notable Python libraries such as ‘requests’ and ‘httpie,’ Starlette offers a robust solution for web development.
Handling essential aspects like routing, middleware, and request/response flow, Starlette operates within the ASGI framework. What sets Starlette apart is its flexibility in allowing developers to make independent choices regarding ORM (Object-Relational Mapping) and database tools. This unique feature grants developers the freedom to select the most suitable options for their specific project requirements.
Advantages of Starlette
In this section, we’ll explore some advantages of Python-Starlette:
- ASGI Compatibility: Starlette is constructed on the ASGI standard, a foundational interface for asynchronous web servers and applications in Python. Leveraging ASGI, Starlette efficiently manages asynchronous programming, enabling it to handle a large number of concurrent connections without the need for additional threads or processes.
- Lightweight and Fast: As a minimalist framework, Starlette boasts a concise codebase and a clear separation of concerns. This design promotes ease of understanding and extensibility. Moreover, its emphasis on performance makes Starlette an excellent choice for developing high-speed applications.
- Support for Middleware: Starlette incorporates a middleware system that facilitates the augmentation of functionality in the request/response handling process. This feature proves beneficial for tasks such as authentication, CORS (Cross-Origin Resource Sharing), and more. Middleware can modify the request before it reaches the application or alter the response before it is sent back to the client.
- WebSockets Support: Starlette includes built-in support for handling WebSockets, empowering developers to create real-time, interactive applications. This capability is particularly valuable for applications requiring bidirectional communication between the client and server.
- Testing and Debugging: Starlette provides a dedicated testing client, simplifying the process of writing unit tests for web applications. Additionally, it comes equipped with built-in support for debugging, streamlining the identification and resolution of issues during the development phase.
Required Installation
Install Necessary Libaries
pip install starlette
pip install uvicorn
Run Server : Run the following command to start the server:
uvicorn new:app --reload
Example 1: Basic Starlette Application
Once installed, you can create a new Starlette application by creating a Python file and importing the necessary modules:
Python3
from starlette.applications import Starlette
from starlette.routing import Route
from starlette.responses import PlainTextResponse
async def homepage(request):
return PlainTextResponse( "Hello, World!" )
app = Starlette(routes = [
Route( "/" , homepage)
])
|
Output
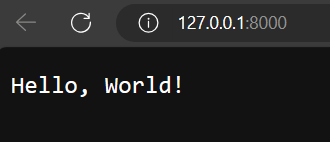
Output
Examples 2: Middleware Implementation
Below code creates a Starlette web application with CORS middleware enabled to allow all origins. It defines a route for the homepage (“/”) that, when accessed, responds with a plain text message showcasing the implementation of middleware.
Python3
from starlette.applications import Starlette
from starlette.responses import PlainTextResponse
from starlette.middleware.cors import CORSMiddleware
app = Starlette()
app.add_middleware(CORSMiddleware, allow_origins = [ "*" ])
@app .route( "/" )
async def homepage(request):
return PlainTextResponse( "Hello, It's an example of middleware implementation!" )
|
Output :
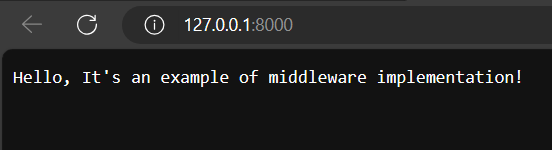
Middleware Implementation
Example 3: Testing & Debugging
Below code demonstrates a simple Starlette web application with a single route (“/”) that responds with a plain text message. The application is tested using the TestClient
class, ensuring the homepage route returns a successful response (status code 200) with the expected text. If executed as a script, the application runs on localhost (127.0.0.1) and port 8000 using the UVicorn server. This example is useful for understanding the basics of creating, testing, and running a Starlette web application for testing and debugging purposes.
Python
from starlette.applications import Starlette
from starlette.responses import PlainTextResponse
from starlette.testclient import TestClient
app = Starlette()
@app .route( "/" )
async def homepage(request):
return PlainTextResponse( "Hello, This is an example of Testing & Debugging!" )
def test_homepage():
client = TestClient(app)
response = client.get( "/" )
assert response.status_code = = 200
assert response.text = = "Hello, This is an example of Testing & Debugging!"
if __name__ = = "__main__" :
import uvicorn
uvicorn.run(app, host = "127.0.0.1" , port = 8000 )
|
Output
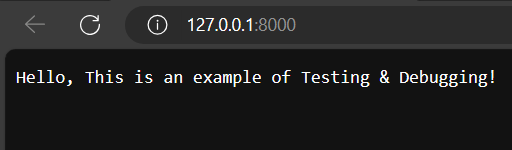
Testing & Debugging
Example 4: Websocket Support
Below code showcases a basic Starlette web application with WebSocket support. It defines a root route (“/”) handled by an asynchronous function called homepage
, responding with a plain text message. The script includes a test function (test_homepage
) using TestClient
to simulate a request and validate the expected response. When executed as a script, the application runs on localhost (127.0.0.1) and port 8000 using the UVicorn server.
Python3
from starlette.applications import Starlette
from starlette.responses import PlainTextResponse
from starlette.testclient import TestClient
app = Starlette()
@app .route( "/" )
async def homepage(request):
return PlainTextResponse( "Hello, This is an example of Websocket Support!" )
def test_homepage():
response = TestClient(app).get( "/" )
assert response.status_code = = 200
assert response.text = = "Hello, This is an example of Websocket Support!"
if __name__ = = "__main__" :
import uvicorn
uvicorn.run(app, host = "127.0.0.1" , port = 8000 )
|
Output
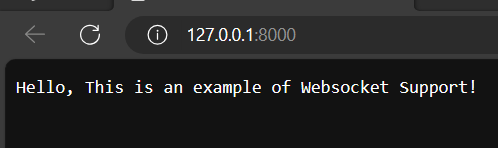
output
Conclusion
In summary, Starlette is a lightweight and versatile Python web framework designed for building fast and asynchronous web applications. It offers simplicity, flexibility, and features like WebSocket support and middleware, making it suitable for a wide range of projects, from simple APIs to high-performance applications. Its compatibility with ASGI enhances its integration capabilities with other asynchronous servers. Overall, Starlette provides developers with the tools to create modern and responsive web services in Python.
Share your thoughts in the comments
Please Login to comment...