Python SQLite – Working with Date and DateTime
Last Updated :
20 Sep, 2021
SQLite does not support built-in DateTime storage a class, but SQLite allows us to work with timestamp types. We can store and retrieve Python date and datetime information stored in the SQLite database tables by converting them to Python date and datetime types and vice-versa.
While inserting the datetime value, the python sqlite3 module converts the datetime into the string format. And when retrieving datetime values from SQLite tables, the sqlite3 module converts them into a string object. But we don’t want string type. We want the datetime to get stored in DateTime type. For that, we need to use detect_types as it takes PARSE_DECLTYPES and PARSE_COLNAMES as arguments in the connect method of the sqlite3 module.
Syntax: connect(‘DATABASE NAME’,detect_types=sqlite3.PARSE_DECLTYPES | sqlite3.PARSE_COLNAMES)
sqlite3.PARSE_DECLTYPES: The sqlite3 module parses the declared type for each column it returns then uses the type converters dictionary to execute the converter function registered for that type there.
sqlite3.PARSE_COLNAMES: The SQLite interface parses the column name for each column it returns. It will use the converters dictionary and then use the converter function found there to return the value.
Inserting Date and DateTime data
First, we need to import the datetime module and to get the current time and date information now() function can be used. Then they store the datetime information in a variable, so it can be used to insert datetime in the SQLite table. To store the datetime information in the table, we need to use the column datatype as ‘TIMESTAMP’.
column_name TIMESTAMP
Below code creates a database ‘StudentAssignment.db’ and a table ‘ASSIGNMENT’. The code also inserts the data into the table with datetime information.
Python3
import datetime
import sqlite3
currentDateTime = datetime.datetime.now()
connection = sqlite3.connect( 'StudentAssignment.db' ,
detect_types = sqlite3.PARSE_DECLTYPES |
sqlite3.PARSE_COLNAMES)
cursor = connection.cursor()
createTable =
cursor.execute(createTable)
insertQuery =
cursor.execute(insertQuery, ( 1 , "Virat Kohli" ,
currentDateTime))
cursor.execute(insertQuery, ( 2 , "Rohit Pathak" ,
currentDateTime))
print ( "Data Inserted Successfully !" )
connection.commit()
cursor.close()
connection.close()
|
Output:
Data Inserted Successfully !
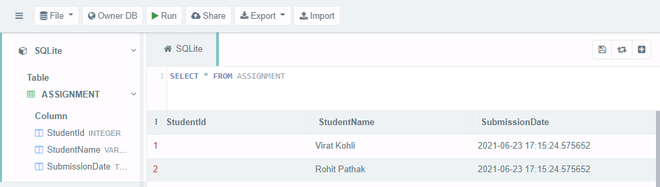
Retrieve Date and DateTime data
In order to retrieve the stored datetime information from database tables, we can simply use the select query and can access the individual rows data. Here for retrieval of data and to check the datatypes in which the data is stored, we need to use the detect_types as arguments in the connect method of the sqlite3 module.
The below code retrieves the stored record from the ‘ASSIGNMENT’ table. The record contains the ‘datetime’ type information.
Python3
import datetime
import sqlite3
connection = sqlite3.connect( 'StudentAssignment.db' ,
detect_types = sqlite3.PARSE_DECLTYPES |
sqlite3.PARSE_COLNAMES)
cursor = connection.cursor()
cursor.execute( "SELECT * from ASSIGNMENT where StudentId = 2" )
fetchedData = cursor.fetchall()
for row in fetchedData:
StudentID = row[ 0 ]
StudentName = row[ 1 ]
SubmissionDate = row[ 2 ]
print (StudentName, ", ID -" ,
StudentID, "Submitted Assignments" )
print ( "Date and Time : " ,
SubmissionDate)
print ( "Submission date type is" ,
type (SubmissionDate))
cursor.close()
connection.close()
|
Output:

As we can see in the output when we retrieve the submission data of students from ‘ASSIGNMENT’ table. We accessed each row of the table and printed them in a specific message. First-line contains the student name and the ID, on the second line we printed the datetime on which the student submitted the assignment, and on the third line we printed the type of the stored data, which is the type of datetime we stored in the table i.e., ‘datetime.datetime’.
Share your thoughts in the comments
Please Login to comment...