Python Requests Readtimeout Error
Last Updated :
26 Feb, 2024
Python’s requests
library is a powerful tool for making HTTP requests, but like any software, it is not without its quirks. One common issue users encounter is the ReadTimeout error, which occurs when the server takes too long to send a response. In this article, we’ll delve into the ReadTimeout error, explore its causes, and provide a step-by-step guide on how to fix it.
What is Requests Readtimeout Error?
The ReadTimeout error in Python’s requests library typically occurs when a request takes longer to complete than the specified timeout duration. This can happen for various reasons, such as slow network conditions, a server-side delay, or an incorrect timeout parameter.
Syntax:
ReadTimeout error: Server did not respond within the specified timeout
Why does Requests Readtimeout Error Occur?
The common reason for Requests ReadTimeout error arises when a server fails to provide data within the specified time frame, leaving the client waiting. Common culprits include slow server responses, network delays, or intricate server-side processing. Picture a scenario where a Python script utilizing the Requests library attempts to fetch data from a remote server. Despite the apparent simplicity of the task, the script abruptly halts, signaling a ReadTimeout error—a clear indication that the server did not respond within the expected timeframe.
Python3
import requests
try :
print (response.text)
except requests.exceptions.ReadTimeout:
print ( "ReadTimeout error: Server did not respond within the specified timeout." )
|
Output :
ReadTimeout error: Server did not respond within the specified timeout.
Approach to Solve Requests Readtimeout Error
The Python Requests ReadTimeout error must be resolved methodically, as follows:
To solve the ReadTimeout issue, analyze request payload size, network performance, and server response times. Adjust the timeout option in the Requests library to give the server more response time, finding the optimal balance. Optimize server efficiency through resource management, data caching, and query optimization to minimize ReadTimeout errors. Conduct a network analysis to identify and resolve delays in data transfer. Establish a retry mechanism in the software to automatically retry unsuccessful requests, fine-tuning retry intervals and attempt counts for resilience and resource optimization.
Code Solution
In this example, below Python code defines a function `fetch_data_with_retry` that uses the `requests` library with a retry mechanism for handling ReadTimeout errors. It sets up a session with a custom retry strategy, attempting a specified number of retries on certain HTTP error status codes. The example usage demonstrates fetching data from a URL, with the function handling errors.
Python3
import requests
from requests.adapters import HTTPAdapter
from urllib3.util.retry import Retry
def fetch_data_with_retry(url, timeout = 5 , retries = 3 ):
session = requests.Session()
retry_strategy = Retry(
total = retries,
backoff_factor = 0.5 ,
status_forcelist = [ 500 , 502 , 503 , 504 ],
)
adapter = HTTPAdapter(max_retries = retry_strategy)
try :
response = session.get(url, timeout = timeout)
response.raise_for_status()
return response.text
except requests.exceptions.ReadTimeout:
print ( "ReadTimeout error: Server did not respond within the specified timeout." )
except requests.exceptions.RequestException as e:
print (f "An error occurred: {e}" )
return None
data = fetch_data_with_retry(url)
if data:
print ( "Data fetched successfully:" , data)
else :
print ( "Failed to fetch data." )
|
Output:
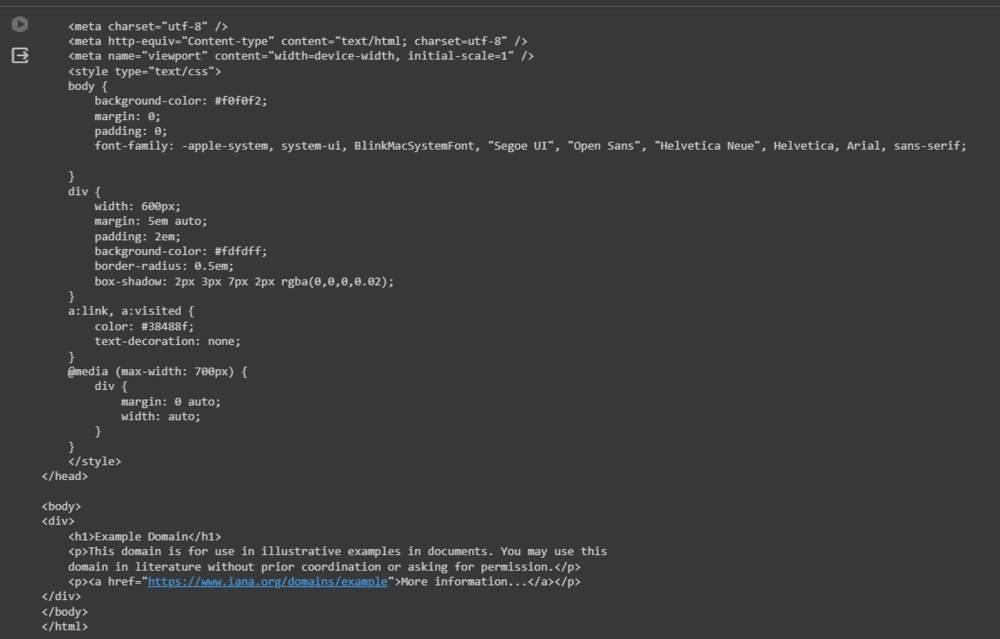
Conclusion
In conclusion, resolving the Python Requests ReadTimeout error involves adjusting timeout values, diagnosing underlying issues with payload size, network, and server performance, optimizing server-side operations, and implementing a retry mechanism. By carefully addressing these aspects, developers can enhance the reliability of their HTTP requests and ensure a smoother interaction with external servers in Python applications.
Share your thoughts in the comments
Please Login to comment...