Python Hex String to Integer List
Last Updated :
12 Mar, 2024
Hexadecimal strings are commonly encountered when working with low-level programming, networking, or cryptographic applications. In Python, converting a hex string to an integer array or list is a frequent task. In this article, we’ll explore some different methods to achieve this conversion.
Hex String To Integer Array
We change a Hex string into a list of numbers, where every 2 hex values make one number. Look at the picture below to see how this change happens. We group 4 bits from each hex to create an 8-bit number. These numbers can range from 0 to 255.
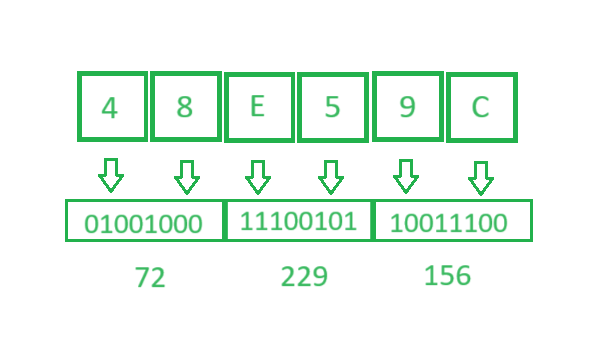
Python Hex String To Integer Array Or List
Below, are the ways to convert Python Hex String To Integer Array Or List.
Python Hex String To Integer Array Or List Using map()
In this example, below code transforms the hex string “1a2b3c4d” into an integer array using a lambda function and map(). It prints the original hex string and the resulting integer array, but there’s a typo in the print statement; it should be corrected to “integerArray.”
Python3
hexString = "1a2b3c4d"
intArray = list ( map ( lambda x: int (x, 16 ),
[hexString[i:i + 2 ] for i in range ( 0 , len (hexString), 2 )]))
print ( "Hex String: " ,hexString)
print ( "Integer array: " ,intArray)
|
Output
Hex String: 1a2b3c4d
Integer array: [26, 43, 60, 77]
Python Hex String To Integer Array Or List Using List Comprehension
In this example, below code converts the hex string ’48E59C7′ into an integer array, where each pair of hex values corresponds to an integer. It then prints both the original hex string and the resulting integer array.
Python3
hexString = '48E59C7'
intArray = [ int (hexString[i:i + 2 ], 16 ) for i in range ( 0 , len (hexString), 2 )]
print ( "Hex String: " ,hexString)
print ( "Integer array: " ,intArray)
|
Output
Hex String: 48E59C7
Integer array: [72, 229, 156, 7]
Python Hex String To Integer Array Or List Using bytes.from( )
In this example, below code transforms the hex string ’48E59C’ into an integer array using the `bytes.fromhex()` method. The resulting integer array is then printed, along with the original hex string.
Python3
hexString = '48E59C'
intArray = list (bytes.fromhex(hexString))
print ( "Hex String: " ,hexString)
print ( "Integer array: " ,intArray)
|
Output
Hex String: 48E59C
Integer array: [72, 229, 156]
Conclusion
In conclusion, converting a hex string to an integer array or list in Python can be accomplished through various methods. Whether using list comprehension, map(), bytearray.fromhex(), or struct.unpack(), each approach provides a distinct way to handle the conversion. The choice of method may depend on factors such as readability, performance, or specific project requirements.
Share your thoughts in the comments
Please Login to comment...