PL/SQL Exception Handling Division by Zero
Last Updated :
19 Mar, 2024
In database management and programming, handling exceptions is vital for reliability. A common problem is the ‘divide by zero’ error, especially in PL/SQL. It’s crucial to handle this to avoid runtime issues and data corruption.
Imagine you’re working on your computer program, and everything seems fine, but suddenly, you hit a big problem: you’re trying to divide a number by zero! It’s like trying to split a cookie into zero pieces – it just doesn’t make sense. This error, called “division by zero,” can mess up your program and cause it to crash.
Understanding the ‘Divide by Zero’ Exception
The ‘divide by zero’ exception occurs when attempting to divide a number by zero, which is mathematically undefined. In PL/SQL, such an operation can lead to runtime errors, causing disruptions in application execution and potentially compromising data integrity. To mitigate this risk, PL/SQL provides mechanisms for handling exceptions, including the ‘divide by zero‘ scenario.
Handling the Exception
Identify Division Operations: Identify any division operations in your code where a denominator value could potentially be zero.
- Use Conditional Checks: Implement conditional checks to ensure that the denominator is not zero before performing division. This involves verifying the value of the denominator variable to avoid division by zero.
- Implement Error Handling: If a division operation is attempted with a zero denominator, handle the error gracefully using exception handling mechanisms provided by your programming language or platform. In PL/SQL, the EXCEPTION block is used to catch the “divide by zero” exception.
- Graceful Exception Handling: Within the exception block, specify the actions to be taken when the error occurs. This may include displaying an error message to the user, logging the error for debugging purposes, or executing alternative logic to handle the exceptional condition.
- Preventative Measures: In addition to handling errors reactively, consider implementing preventative measures to reduce the likelihood of encountering the “divide by zero” error altogether. This may involve validating input values, implementing fallback mechanisms, or restructuring code to avoid division operations with potential zero denominators.
- Testing and Validation: Thoroughly test your code to ensure that the error handling mechanisms function as intended. Verify that division operations with non-zero denominators produce the expected results, while division operations with zero denominators are handled gracefully without causing crashes or unexpected behavior.
By following these steps, you can effectively handle the “divide by zero” error in your code, ensuring robustness, reliability, and user satisfaction.
Catching the Exception
To catch the ‘divide by zero‘ exception in PL/SQL, developers can utilize the EXCEPTION block within their code. By encapsulating the division operation within a try-catch construct, the program can gracefully handle the exception without terminating abruptly. Below is a basic example demonstrating how to catch the ‘divide by zero’ exception:
PL/SQL Code:
DECLARE
numerator NUMBER := 10;
denominator NUMBER := 0;
result NUMBER;
BEGIN
-- Attempting division
BEGIN
result := numerator / denominator;
EXCEPTION
WHEN ZERO_DIVIDE THEN
DBMS_OUTPUT.PUT_LINE('Error: Division by zero');
END;
END;
Statement processed.
Error: Division by zero
Output:
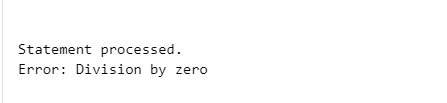
Catching “Divide By zero” error
Explanation: The division operation numerator/denominator is enclosed within a BEGIN-END block. If a ‘divide by zero‘ error occurs during execution, the EXCEPTION block intercepts the error and executes the specified handling logic, printing an error message to the console.
Preventing Divide by Zero Errors
While catching the ‘divide by zero’ exception is essential for error management, proactive prevention is equally important. Developers can employ conditional checks to verify the divisor before executing division operations. For instance:
PL/SQL code:
DECLARE
numerator NUMBER := 10;
denominator NUMBER := 0;
result NUMBER;
BEGIN
-- Check if denominator is not zero before division
IF denominator != 0 THEN
result := numerator / denominator;
DBMS_OUTPUT.PUT_LINE('Result: ' || result);
ELSE
-- Handle division by zero scenario
DBMS_OUTPUT.PUT_LINE('Error: Division by zero');
END IF;
END;
Statement processed.
Error: Division by zero
if error does not occur: numerator NUMBER := 10; denominator NUMBER := 2;
Output:
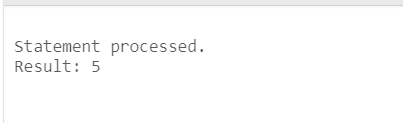
Output result
If error occurs: numerator NUMBER := 10; denominator NUMBER := 0;
Output:
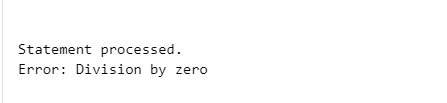
Output result
Explanation: By checking the divisor in advance, developers can avoid ‘divide by zero’ errors completely, improving the robustness and robustness of PL/SQL code.
Conclusion
In the ever-changing world of making software, being really good at dealing with mistakes isn’t just a bonus – it’s a must-have skill. The divide by zero problem is like a test for how tough our programs are. But if we learn all about it, get good at handling mistakes nicely, and take steps to stop it from happening, we make our programs much stronger. So, as you start learning about PL/SQL and how to work with databases, remember this: even when mistakes happen, if we’re ready for them and know what to do, we can keep moving forward and getting better. Each time we fix a mistake, we’re getting closer to being really awesome at programming, where mistakes don’t bother us much at all.
Share your thoughts in the comments
Please Login to comment...