Perl | Multidimensional Arrays
Last Updated :
14 Sep, 2021
Multidimensional arrays in Perl are the arrays with more than one dimension. Technically there is no such thing as a multidimensional array in Perl but arrays are used to act as they have more than one dimension. Multi dimensional arrays are represented in the form of rows and columns, also knows as matrix. Each element of an array can be a reference to another array but in syntax, they will appear like a 2-dimensional array.
A multidimensional array can only hold scalar values, they can not hold arrays or hashes.
Initialization and Declaration of a Multidimensional Array
Given below is the example that makes clear the initialization and declaration of a Multidimensional array.
In this example we will simply initialize the array by @array_name = ([…], […], […]);.
Perl
#!/usr/bin/perl
use strict;
use warnings;
my @items = ( [ 'book' , 'pen' , 'pencil' ],
[ 'Bat' , 'Ball' , 'Stumps' ],
[ 'snake' , 'rat' , 'rabbit' ] );
print $items [0][0], "\n" ;
print $items [1][1], "\n" ;
print $items [2][2], "\n" ;
|
Output:
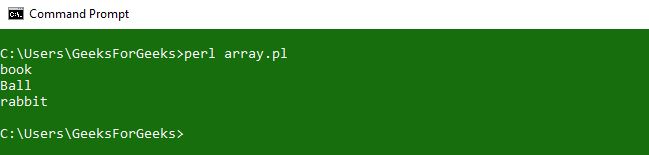
Creating a Matrix
Matrix is a collection of rows and columns of arrays that appears to be in more than one dimension.
Let’s see an example to make it more clear of creating a matrix in Perl.
Example 1:
In this example, we first declared three arrays with values and then merged them into a final resulted array to form a 3*3 matrix. In order to control the variable $m and $n, two for loops are used.
Perl
#!/usr/bin/perl
use strict;
use warnings;
my @arrayA = qw(1 0 0) ;
my @arrayB = qw(0 1 0) ;
my @arrayC = qw(0 0 1) ;
my @result = (\ @arrayA , \ @arrayB , \ @arrayC );
print "Resultant 3*3 Matrix:\n" ;
for ( my $m = 0; $m <= $#result ; $m ++)
{
for ( my $n = 0; $n <= $#result ; $n ++)
{
print "$result[$m][$n] " ;
}
print "\n" ;
}
|
Output:
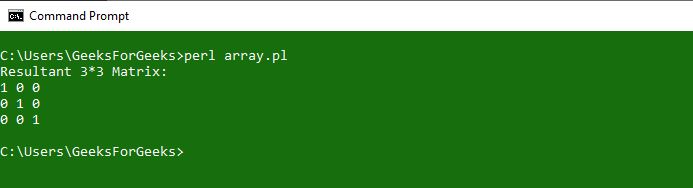
Example 2:
In this example, we will take the input from the keyboard and then add the two matrices and printing the result using the resultant matrix.
Perl
#!usr/bin/perl
use strict;
use warnings;
my ( @MatrixA , @MatrixB , @Resultant ) = ((), (), ());
print "Please Provide the order of MatrixA :\n" ;
print "\tMatrixA rows:" ;
chomp ( my $rowA = <>);
print "\tMatrixA columns:" ;
chomp ( my $columnA = <>);
print "Please Provide the order of MatrixB :\n" ;
print "\tMatrixB rows:" ;
chomp ( my $rowB = <>);
print "\tMatrixB columns:" ;
chomp ( my $columnB = <>);
if ( $rowA == $rowB and $columnA == $columnB )
{
print "Enter $rowA * $columnA elements in MatrixA:\n" ;
foreach my $m (0.. $rowA - 1)
{
foreach my $n (0.. $columnA - 1)
{
chomp ( $MatrixA [ $m ][ $n ] = <>);
}
}
print "Enter $rowB * $columnB elements in MatrixB:\n" ;
foreach my $m (0.. $rowB - 1)
{
foreach my $n (0.. $columnB - 1)
{
chomp ( $MatrixB [ $m ][ $n ] = <>);
}
}
foreach my $m (0.. $rowB - 1)
{
foreach my $n (0.. $columnB - 1)
{
$Resultant [ $m ][ $n ] = $MatrixA [ $m ][ $n ] +
$MatrixB [ $m ][ $n ];
}
}
print "MatrixA is :\n" ;
foreach my $m (0.. $rowB - 1)
{
foreach my $n (0.. $columnB - 1)
{
print "$MatrixA[$m][$n] " ;
}
print "\n" ;
}
print "MatrixB is :\n" ;
foreach my $m (0.. $rowB - 1)
{
foreach my $n (0.. $columnB - 1)
{
print "$MatrixB[$m][$n] " ;
}
print "\n" ;
}
print "SUM of MatrixA and MatrixB is :\n" ;
foreach my $m (0.. $rowB - 1)
{
foreach my $n (0.. $columnB - 1)
{
print "$Resultant[$m][$n] " ;
}
print "\n" ;
}
}
else
{
print "Matrices order does not MATCH, Addition is not Possible" ;
}
|
Output:
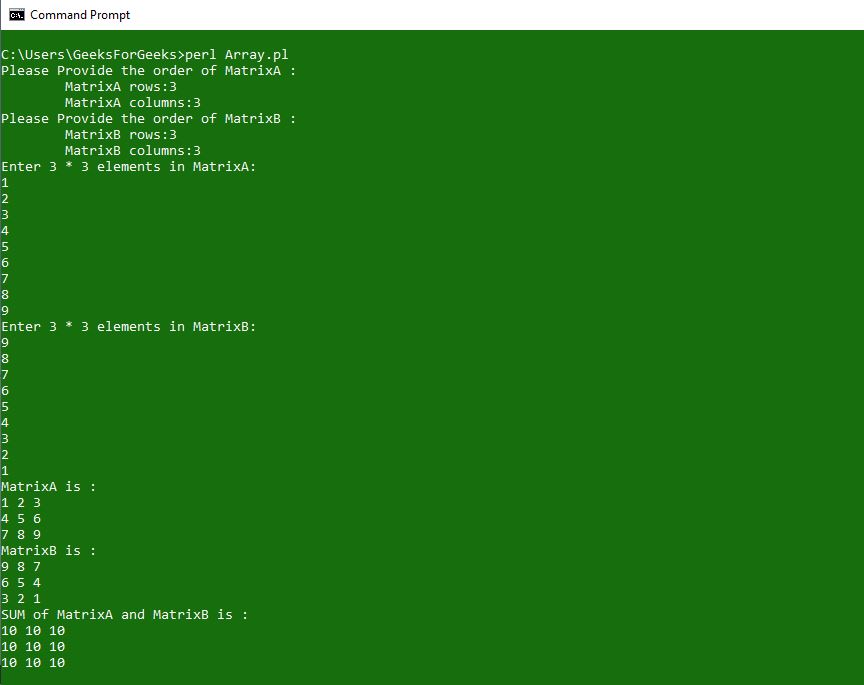
Addition of Matrices can only be performed if the matrices are of the same order.
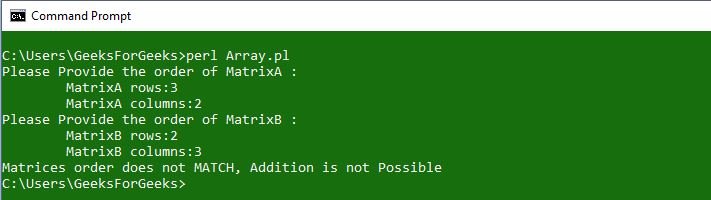
Array of Arrays
Array of Arrays are the data structures having an array having list of array references. Elements inside an array are the array references. These references can be printed individually or the whole array can be printed as well, as per the requirement.
Syntax:
@ARRAY_NAME = ([value1], [value2], [value3], ..., [valueN]);
Example 1:
Perl
#!/usr/bin/perl
use strict;
use warnings;
my @GfG = ( [ "Geek" , "For" , "Geeks" ],
[ "Is" , "Best" , "For" ],
[ "Those" , "In" , "Need" ] );
print "Accessing Array elements:\n" ;
print @{ $GfG [0]}[1], "\n" ;
print @{ $GfG [1]}[0], "\n" ;
print @{ $GfG [2]}[2], "\n" ;
print "\nAccessing whole arrays: \n" ;
print @{ $GfG [0]}, "\n" ;
print @{ $GfG [1]}, "\n" ;
print @{ $GfG [2]}, "\n" ;
print "\n" ;
|
Output:
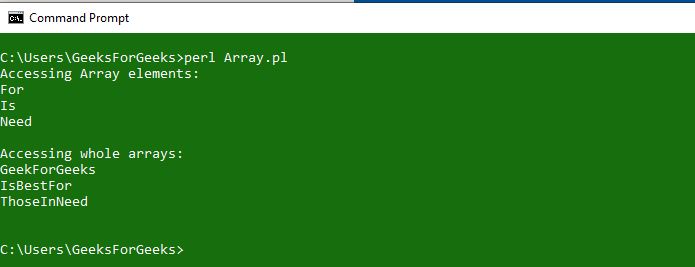
Example 2:
In this example, we generated an array of array by using a single array.
Perl
#!/usr/bin/perl
use strict;
use warnings;
use Data::Dumper;
my @GfG = ( [ "SAM" , "SHABANAM" , "SOHAM" ],
[ "DHONI" , "GONI" , "AVNI" ],
[ "VIRAT" , "STUART" , "ROHIT" ] );
my @test ;
foreach ( @GfG )
{
if ( $_ =~ /M/ )
{
push ( @{ $test [0]}, $_ );
}
elsif ( $_ =~ /I/ )
{
push ( @{ $test [1]}, $_ );
}
else
{
push ( @{ $test [2]}, $_ );
}
}
print Dumper(\ @test ), "\n" ;
|
Output:
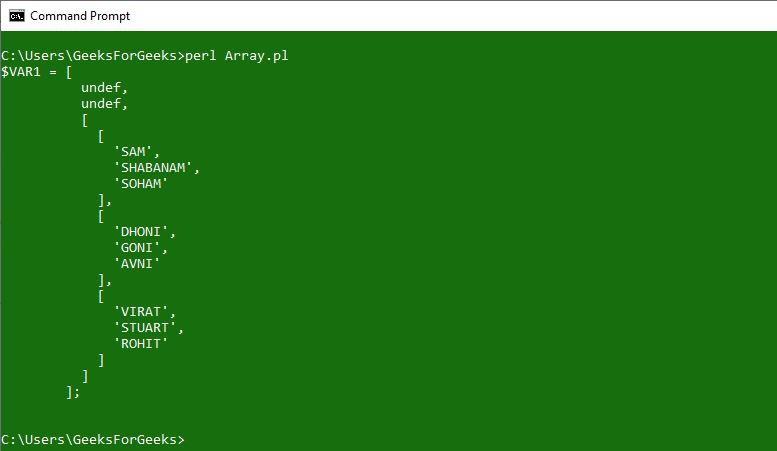
Array of Hashes
Array of hashes is the data structure where the array has a list of hashes. Hash references are the objects of an array. In order to access the key and values, we need to de-reference them.
Array of hashes is a great data structure if we want to loop through hashes numerically.
Syntax:
@ARRAY_NAME = ({KEY1 => VALUE1}, {KEY2 => VALUE2});
Example 1:
Perl
#!/usr/bin/perl
use strict;
use warnings;
my @hashtest = ({ First => "Geek" ,
Middle => "For" ,
Last => "Geeks" },
{ Street => "Royal Kapsons" ,
City => "Noida-Uttar Pradesh" },
{ About => "Computer Science portal" ,
Content => "Technical" });
print $hashtest [0], "\n" ;
print keys %{ $hashtest [2]}, "\n" ;
print $hashtest [0]->{ "First" }, "\n" ;
print $hashtest [0]->{ "Middle" }, "\n" ;
print $hashtest [0]->{ "Last" }, "\n" ;
|
Output:
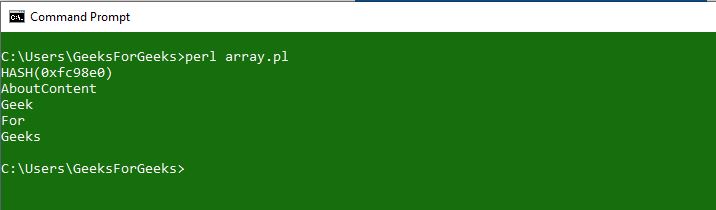
Example 2:
Generating an array of hashes from a simple array.
Perl
#!/usr/bin/perl
use strict;
use warnings;
use Data::Dumper;
my @array = ( "Item - Java Course" ,
"Cost - 5000" ,
"Ratings - 5 star" );
my @array_1 ;
foreach ( @array )
{
my @array = split ( " - " , $_ );
$array_1 [0]->{ $array [0]} = $array [1];
}
print Dumper (\ @array_1 );
|
Output:
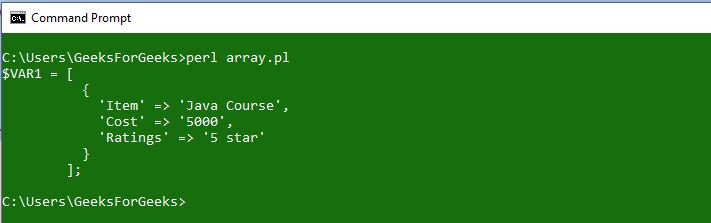
Share your thoughts in the comments
Please Login to comment...