Order of Constructor/ Destructor Call in C++
Last Updated :
14 Sep, 2023
Prerequisite: Constructors
Whenever we create an object of a class, the default constructor of that class is invoked automatically to initialize the members of the class.
If we inherit a class from another class and create an object of the derived class, it is clear that the default constructor of the derived class will be invoked but before that the default constructor of all of the base classes will be invoke, i.e the order of invocation is that the base class’s default constructor will be invoked first and then the derived class’s default constructor will be invoked.
Why the base class’s constructor is called on creating an object of derived class?
To understand this you will have to recall your knowledge on inheritance. What happens when a class is inherited from other? The data members and member functions of base class comes automatically in derived class based on the access specifier but the definition of these members exists in base class only. So when we create an object of derived class, all of the members of derived class must be initialized but the inherited members in derived class can only be initialized by the base class’s constructor as the definition of these members exists in base class only. This is why the constructor of base class is called first to initialize all the inherited members.
C++
#include <iostream>
using namespace std;
class Parent
{
public :
Parent()
{
cout << "Inside base class" << endl;
}
};
class Child : public Parent
{
public :
Child()
{
cout << "Inside sub class" << endl;
}
};
int main() {
Child obj;
return 0;
}
|
Output:
Inside base class
Inside sub class
Order of constructor call for Multiple Inheritance
For multiple inheritance order of constructor call is, the base class’s constructors are called in the order of inheritance and then the derived class’s constructor.
C++
#include <iostream>
using namespace std;
class Parent1
{
public :
Parent1()
{
cout << "Inside first base class" << endl;
}
};
class Parent2
{
public :
Parent2()
{
cout << "Inside second base class" << endl;
}
};
class Child : public Parent1, public Parent2
{
public :
Child()
{
cout << "Inside child class" << endl;
}
};
int main() {
Child obj1;
return 0;
}
|
Output:
Inside first base class
Inside second base class
Inside child class
Order of constructor and Destructor call for a given order of Inheritance
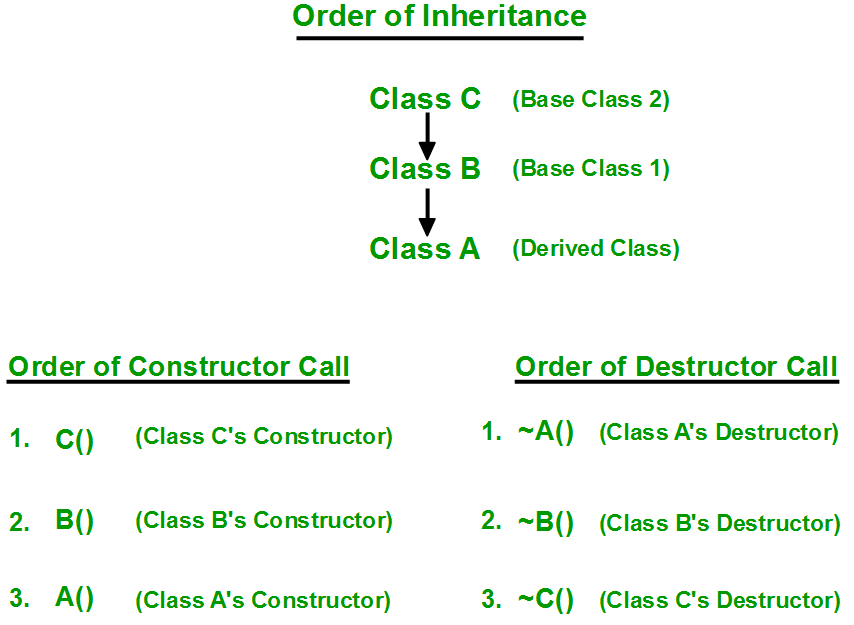
How to call the parameterized constructor of base class in derived class constructor?
To call the parameterized constructor of base class when derived class’s parameterized constructor is called, you have to explicitly specify the base class’s parameterized constructor in derived class as shown in below program:
C++
#include <iostream>
using namespace std;
class Parent {
int x;
public :
Parent( int i)
{
x = i;
cout << "Inside base class's parameterized "
"constructor"
<< endl;
}
};
class Child : public Parent {
public :
Child( int x): Parent(x)
{
cout << "Inside sub class's parameterized "
"constructor"
<< endl;
}
};
int main()
{
Child obj1(10);
return 0;
}
|
Output:
Inside base class's parameterized constructor
Inside sub class's parameterized constructor
Important Points:
- Whenever the derived class’s default constructor is called, the base class’s default constructor is called automatically.
- To call the parameterized constructor of base class inside the parameterized constructor of sub class, we have to mention it explicitly.
- The parameterized constructor of base class cannot be called in default constructor of sub class, it should be called in the parameterized constructor of sub class.
Destructors in C++ are called in the opposite order of that of Constructors.
Share your thoughts in the comments
Please Login to comment...