OpenMP | Hello World program
Last Updated :
01 Nov, 2023
Prerequisite: OpenMP | Introduction with Installation Guide
In C/C++/Fortran, parallel programming can be achieved using OpenMP. In this article, we will learn how to create a parallel Hello World Program using OpenMP.
STEPS TO CREATE A PARALLEL PROGRAM
- Include the header file: We have to include the OpenMP header for our program along with the standard header files.
//OpenMP header
#include <omp.h>
-
- Specify the parallel region: In OpenMP, we need to mention the region which we are going to make it as parallel using the keyword pragma omp parallel. The pragma omp parallel is used to fork additional threads to carry out the work enclosed in the parallel. The original thread will be denoted as the master thread with thread ID 0. Code for creating a parallel region would be,
#pragma omp parallel
{
//Parallel region code
}
- So, here we include
#pragma omp parallel
{
printf("Hello World... from thread = %d\n",
omp_get_thread_num());
}
-
- Set the number of threads:
we can set the number of threads to execute the program using the external variable.
export OMP_NUM_THREADS=5
- Diagram of parallel region
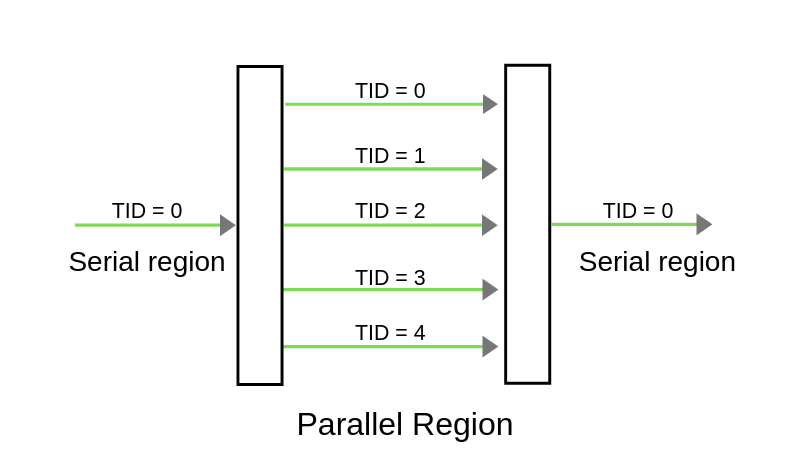
- As per the above figure, Once the compiler encounters the parallel regions code, the master thread(thread which has thread id 0) will fork into the specified number of threads. Here it will get forked into 5 threads because we will initialise the number of threads to be executed as 5, using the command export OMP_NUM_THREADS=5. Entire code within the parallel region will be executed by all threads concurrently. Once the parallel region ended, all threads will get merged into the master thread.
- Compile and Run:
Compile:
gcc -o hello -fopenmp hello.c
- Execute:
./hello
-
Below is the complete program with the output of the above approach:
Program: Since we specified the number of threads to be executed as 5, 5 threads will execute the same print statement at the same point of time. Here we can’t assure the order of execution of threads, i.e Order of statement execution in the parallel region won’t be the same for all executions. In the below picture, while executing the program for first-time thread 1 gets completed first whereas, in the second run, thread 0 completed first. omp_get_thread_num() will return the thread number associated with the thread.
OpenMP Hello World program
#include <omp.h>
#include <stdio.h>
#include <stdlib.h>
int main( int argc, char * argv[])
{
#pragma omp parallel
{
printf ( "Hello World... from thread = %d\n" ,
omp_get_thread_num());
}
}
|
Output:
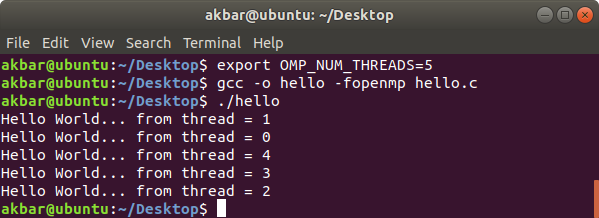
-
- When run for multiple time: Order of execution of threads changes every time.
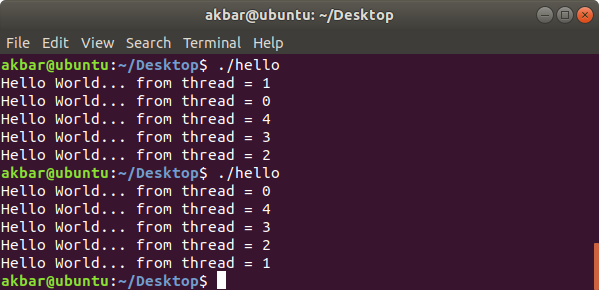
Share your thoughts in the comments
Please Login to comment...