One Dimensional Arrays in C
Last Updated :
03 May, 2024
In C, an array is a collection of elements of the same type stored in contiguous memory locations. This organization allows efficient access to elements using their index. Arrays can also be of different types depending upon the direction/dimension they can store the elements. It can be 1D, 2D, 3D, and more. We generally use only one-dimensional, two-dimensional, and three-dimensional arrays.
In this article, we will learn all about one-dimensional (1D) arrays in C, and see how to use them in our C program.
One-Dimensional Arrays in C
A one-dimensional array can be viewed as a linear sequence of elements. We can only increase or decrease its size in a single direction.
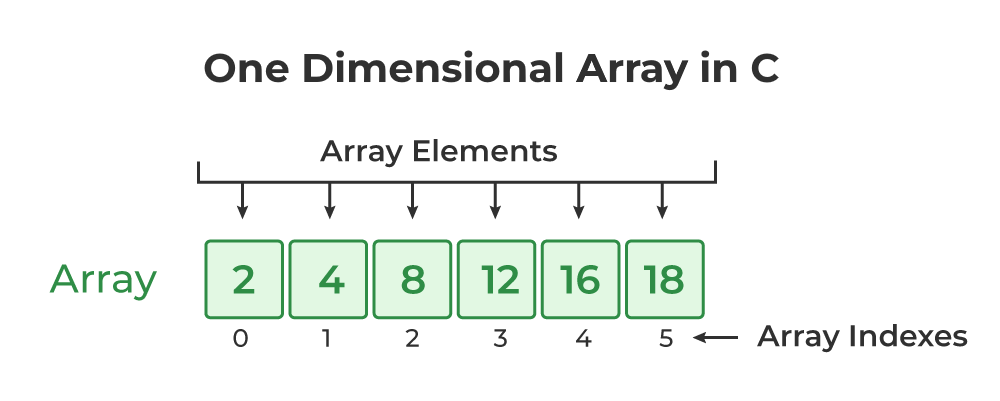
Only a single row exists in the one-dimensional array and every element within the array is accessible by the index. In C, array indexing starts zero-indexing i.e. the first element is at index 0, the second at index 1, and so on up to n-1 for an array of size n.
Syntax of One-Dimensional Array in C
The following code snippets shows the syntax of how to declare an one dimensional array and how to initialize it in C.
1D Array Declaration Syntax
In declaration, we specify then name and the size of the 1d array.
elements_type array_name[array_size];
In this step, the compiler reserved the given amount of memory for the array but this step does not define the value of the elements. They may contain some random values. So we initialize the array to give its elements some initial valu
1D Array Initialization Syntax
In declaration, the compiler reserved the given amount of memory for the array but does not define the value of the element. To assign values, we have to initialize an array.
elements_type array_name[array_size] = {value1, value2, ... };
This type of The values will be assigned sequentially, means that first element will contain value1, second value2 and so on.
Note: The number of elements initialized should be equal to the size of the array. If more elements are given in the initializer list, the compiler will show a warning and they will not be considered.
This initialization only works when performed with declaration.
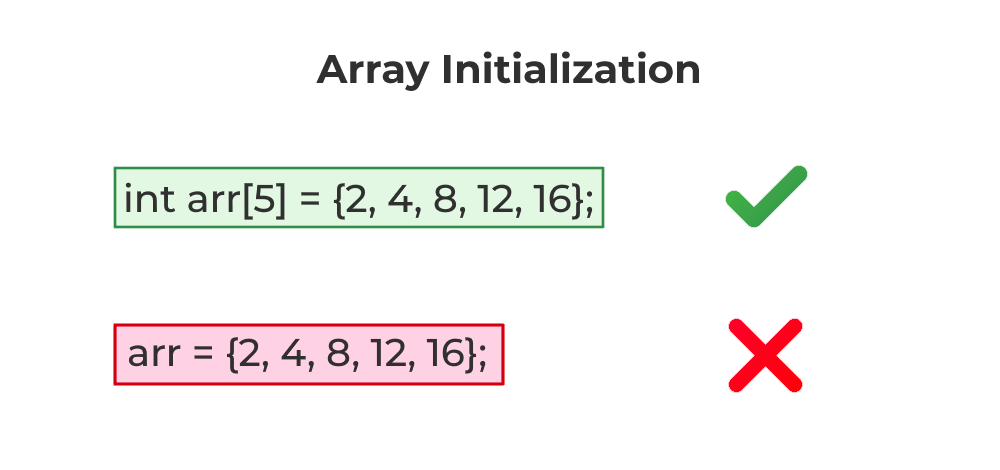
1D Array Element Accessing/Updating Syntax
After the declaration, we can use the index of the element along with the array name to access it.
array_name [index]; // accessing the element
Then, we can also assign the new value to the element using assignment operator.
array_name [index] = new_value; // updating element
Note: Make sure the index lies within the array or else it might lead to segmentation fault.
To access all the elements of the array at once, we can use the loops as shown below.
Example of One Dimensional Array in C
The following example demonstrate how to create a 1d array in a c program.
C
// C program to illustrate how to create an array,
// initialize it, update and access elements
#include <stdio.h>
int main()
{
// declaring and initializing array
int arr[5] = { 1, 2, 4, 8, 16 };
// printing it
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// updating elements
arr[3] = 9721;
// printing again
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Output1 2 4 8 16
1 2 4 9721 16
Memory Representation of One Dimensional Array in C
Memory for an array is allocated in a contiguous manner i.e. all the elements are stored adjacent to its previous and next eleemtn in the memory. It means that, if the address of the first element and the type of the array is known, the address of any subsequent element can be calculated arithmetically.
The address of the ith element will be calculated as given the base address (base) and element size (size):
Address of ith element = base + (i x size)
This structured memory layout makes accessing array elements efficient.
Note: The name of the array is the pointer to its first element. And also, we don’t need to multipy the size of the data type. The compiler already do it for you.
Example: To Demonstrate the 1D Array Memory Layout
C
// C++ program to illustrate the memory layout of one
// dimensional array
#include <stdio.h>
int main()
{
// declaring array
int arr[5] = { 11, 22, 33, 44, 55 };
// pointer to the first element of the array;
int* base = &arr[0];
// size of each element
int size = sizeof(arr[0]);
// accessing element at index 2
printf("arr[2]: %d\n*(base + 2): %d\n", arr[2],
*(base + 2));
printf("Address of arr[2]: %p\n", &arr[2]);
printf("Address of base + 2: %p", base + 2);
return 0;
}
Outputarr[2]: 33
*(base + 2): 33
Address of arr[2]: 0x7fff1841c3c8
Address of base + 2: 0x7fff1841c3c8
As we can see, the arr[2] and (base + 2) are essentially the same.
Array of Characters (Strings)
There is one popular use of array that is the 1D array of characters that is used to represent the textual data. It is more commonly known as strings. The strings are essentially an array of character that is terminated by a NULL character (‘\0’).
Syntax
char name[] = "this is an example string"
Here, we don’t need to define the size of the array. It will automatically deduce it from the assigned string.
Example
C
// C++ Program to illlustrate the use of string
#include <stdio.h>
int main()
{
// defining array
char str[] = "This is GeeksforGeeks";
printf("%s", str);
return 0;
}
OutputThis is GeeksforGeeks
To know more about strings, refer to this article – Strings in C
Share your thoughts in the comments
Please Login to comment...