NPM bcrypt
Last Updated :
10 May, 2024
bcrypt is a popular npm package used for password hashing. It utilizes the bcrypt hashing algorithm, which is designed to be slow and computationally intensive, making it resistant to brute-force attacks even with the increasing computational power of modern hardware.
What is bcrypt?
bcrypt is a password-hashing function designed by Niels Provos and David Mazières and presented at USENIX in 1999 to securely hash passwords. It is based on the Blowfish cipher and incorporates a salt to protect against rainbow table attacks and other common password-cracking techniques. Bcrypt is widely used in the industry and is considered one of the most secure methods for hashing passwords.
The bcrypt function accepts a password string (up to 72 bytes), a numeric cost, and a salt value of 16 bytes (128 bits). Usually, the salt is an arbitrary number. These inputs are used by the bcrypt function to calculate a 24-byte (192-bit) hash. The final output of the bcrypt function is a string of the form:
$2<a/b/x/y>$[cost]$[22 character salt][31 character hash]
Example:
$2a$12$R9h/cIPz0gi.URNNX3kh2OPST9/PgBkqquzi.Ss7KIUgO2t0jWMUW
\__/\/ \____________________/\_____________________________/
Alg Cost Salt Hash
Where:
$2a$: The hash algorithm identifier (bcrypt)
12: Input cost (212 i.e. 4096 rounds)
R9h/cIPz0gi.URNNX3kh2O: A base-64 encoding of the input salt
PST9/PgBkqquzi.Ss7KIUgO2t0jWMUW: A base-64 encoding of the first 23 bytes of the computed 24 byte hash
Installation
You can install this package with npm using following command:
npm install bcrypt
or
npm i bcrypt
Basic Usage:
Once installed, you can include bcrypt in your code using the require function:
const bcrypt = require('bcrypt');
Hashing a Password
To hash a password using bcrypt, you’ll typically use the ” bcrypt.hash() “ function.
JavaScript
const bcrypt = require('bcrypt');
const password = 'gfgDemoPassword';
const saltRounds = 10;
bcrypt.hash(password, saltRounds, function (err, hash) {
if (err) {
console.error(err);
return;
}
console.log(hash);
});
In this example, saltRounds is the number of salt rounds to use. The higher the number of salt rounds, the more computationally intensive the hashing process becomes ( inc. security).
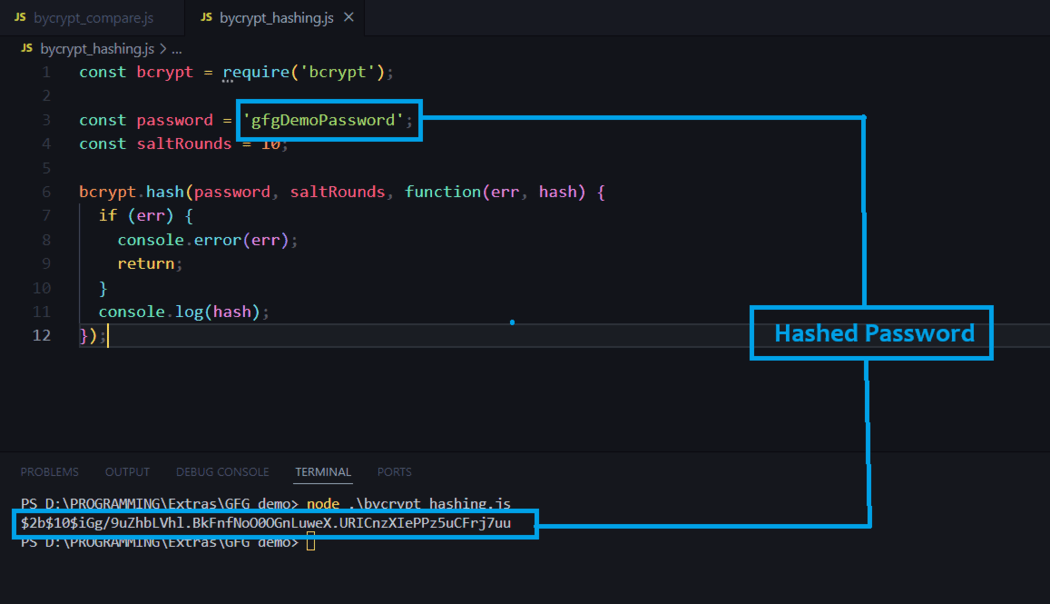
Comparing a Password
To compare a plain-text password with a hashed password, you can use the bcrypt.compare() function.
JavaScript
const bcrypt = require("bcrypt");
const hashedPassword =
"$2b$10$iGg/9uZhbLVhl.BkFnfNoO0OGnLuweX.URICnzXIePPz5uCFrj7uu";
const plainPassword = "gfg1122";
bcrypt.compare(plainPassword, hashedPassword, function (err, result) {
if (err) {
console.error(err);
return;
}
if (result) {
console.log("Password is correct!");
} else {
console.log("Password is incorrect!");
}
});
In this example, plainPassword is the plain-text password that you want to compare, and hashedPassword is the hashed password retrieved from your database. The bcrypt.compare() function compares the plain-text password with the hashed password and returns a boolean value indicating whether the passwords matches or not.
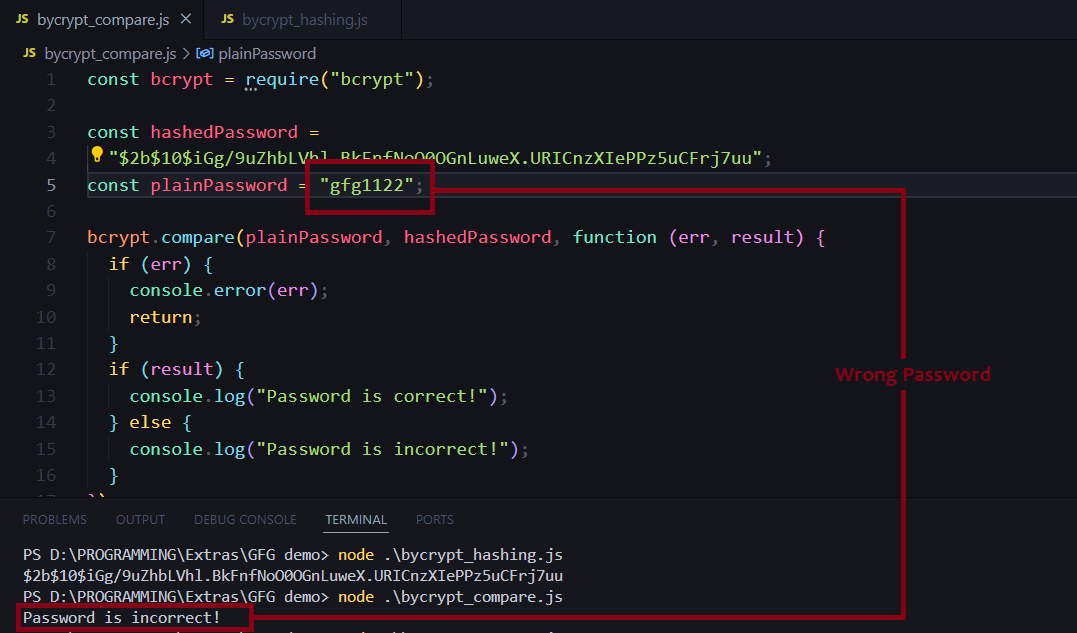
When entered wrong password
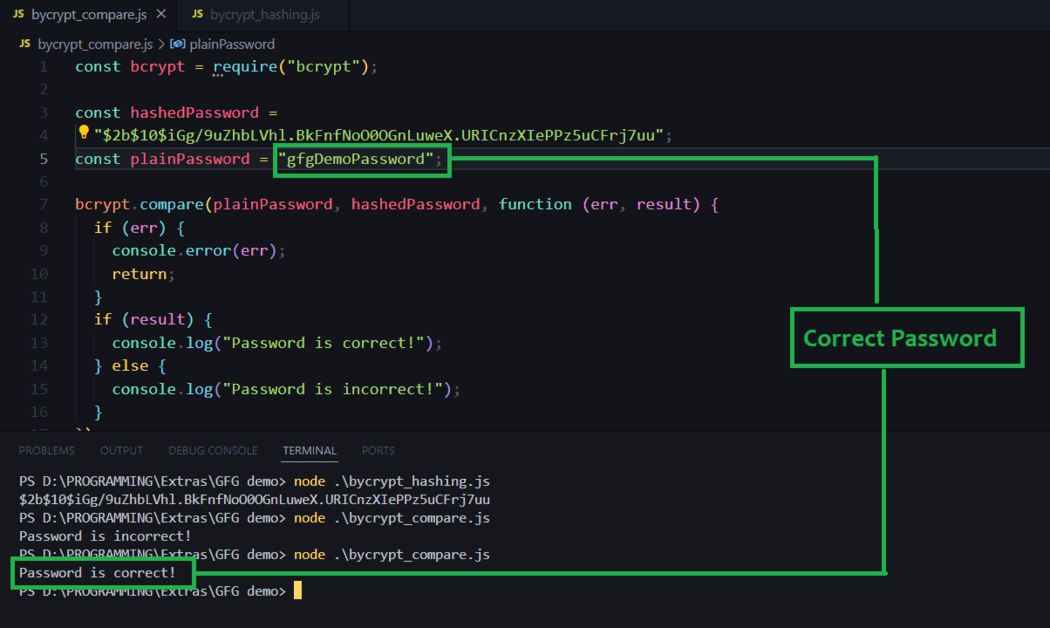
When entered correct password
Why do we use bcrypt for password hashing?
The reasons why bcrypt is the preferred choice for password hashing are following:
- Slow runtime:The slow working of the Bcrypt algorithm makes it difficult for hackers to break password hashes because it takes time to generate hashes and decode them. Security software or a user can detect unusual activity and stop hackers from accessing sensitive data because it takes longer for a threat actor to act.
- Usage of salt: Rainbow table-resistant password hashes can be produced by adding a random piece of data and hashing it with the password. Password salting ensures the highest security requirements for password storage.
- Adapts to changes:Bcrypt is a flexible tool that can change to accommodate optimized hardware and software. The hashing password’s speed of calculation determines its level of security. As computers get more powerful, hackers can hash passwords more quickly. Bcrypt, on the other hand, employs a variable number of password iterations, which can greatly raise computational costs. Therefore, as computers get faster, bcrypt slows down the hashing process, halting threat actors in the same way that slower, outdated methods would.
Share your thoughts in the comments
Please Login to comment...