Multi-Dimensional Arrays in Objective-C
Last Updated :
16 Mar, 2023
Arrays are basically defined as linear data structures that are used to store similar types of data elements. Arrays can be single-dimensional or multi-dimensional. As the name suggests that multi-dimensional means it is a data structure that is used to store similar types of data like(integers, floats, characters, doubles, etc) elements in tabular form where each shell is identified by its row or column number. Or in other words multi-dimensional array in Objective – C can be termed as an array of arrays that stores homogeneous data in tabular form. Data in multidimensional arrays are stored in row-major order.
Syntax:
data_type array_name[size1] [size2] size[3]…….size[N];
Here,
- data_type: Basically it clarifies or defines the type of data to be stored in the array. It is basically defined as the type of data which we are going to store in the array it can be Integer, float, character, etc.
- array_name: Here the name of the array will be declared the naming conventions rules were followed to name the array.
- size1, size2,… ,sizeN: Sizes of the dimensions or we can define it as the size of arrays, or in the two-dimensional array it can be defined as the number of rows and columns in the matrix basically it will form a matrix.
Example of the three-dimensional array
Let’s see an example to see the declaration of a three-dimensional array with sizes 2, 6, and 4 with an array name that is array_name, and the data type is an integer.
int array_name[2][6][4];
so this is a three-dimensional array with sizes and array names and types.
Example of the two-dimensional array
It is basically defined as an array with two dimensions. let’s see it with an example:
int arr[4][4];
It is a two-dimensional square matrix with the name arr and types integer. Or we can say a two-dimensional array is the simplest form of a multidimensional array. Let’s understand it clearly with the help of the diagram:
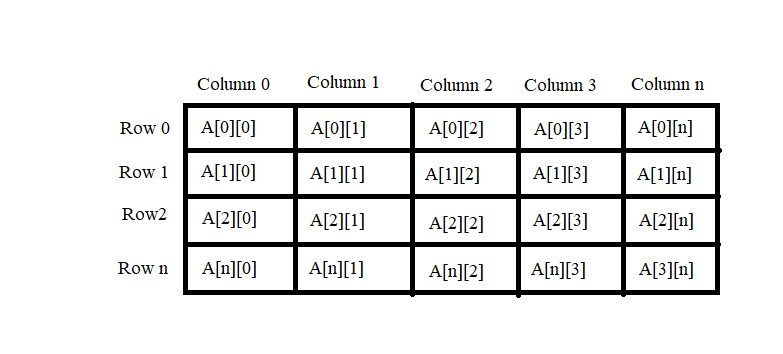
Two Dimensional Array
The simplest form of declaring a two-dimensional array of size a, b:
Syntax:
data_typr array_name[a][b];
Elements in two-dimensional arrays are commonly referred to by arr[i][j] where i is the row number and ‘j’ is the column number.
Initializing Multi-Dimensional Arrays
Let’s understand it by taking the example of a two-dimensional array because the initialization is the same for all sizes Let’s see it.
int arr [2][2] = { { 20 , 40} , {12 , 24} };
So using brackets [] we can initialize values in each row of the array.
Accessing Elements of Two-Dimensional Arrays
Now we will see how elements in Two-Dimensional arrays are accessed using the row indexes and column indexes when they are initialized. So to access the array we use subscript. Subscript helps us to access the row index and column index of an array as shown in the below example.
int arr [0][1];
Here the element present in the 0th row and 1st column will be accessed and printed as the output. Let’s understand all the concepts of Initialization and accessing the elements with the help of examples:
Example 1:
ObjectiveC
#import <Foundation/Foundation.h>
int main ()
{
int arr[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int i, j;
for (i = 0; i < 3; i++ )
{
for (j = 0; j < 3; j++ )
{
NSLog ( @"arr[%d][%d] = %d\n" , i, j, arr[i][j]);
}
}
return 0;
}
|
Output:
2022-12-16 05:18:25.156 main[29483] arr[0][0] = 1
2022-12-16 05:18:25.157 main[29483] arr[0][1] = 2
2022-12-16 05:18:25.157 main[29483] arr[0][2] = 3
2022-12-16 05:18:25.157 main[29483] arr[1][0] = 4
2022-12-16 05:18:25.157 main[29483] arr[1][1] = 5
2022-12-16 05:18:25.157 main[29483] arr[1][2] = 6
2022-12-16 05:18:25.157 main[29483] arr[2][0] = 7
2022-12-16 05:18:25.157 main[29483] arr[2][1] = 8
2022-12-16 05:18:25.157 main[29483] arr[2][2] = 9
As the name suggests that there is any number of dimensions but we basically deal with one, two, or hardly three or four dimensions let’s take an example of a four dimensions array also to initialize elements and access elements.
Example 2:
ObjectiveC
#import <Foundation/Foundation.h>
int main ()
{
int arr[4][4] = {{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12},
{13, 14, 15, 16}};
int i, j;
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
NSLog ( @"arr[%d][%d] = %d\n" , i, j, arr[i][j]);
}
}
return 0;
}
|
Let’s see the output of the 4*4 matrix:
Output:
2022-12-16 05:27:50.994 main[44172] arr[0][0] = 1
2022-12-16 05:27:50.995 main[44172] arr[0][1] = 2
2022-12-16 05:27:50.995 main[44172] arr[0][2] = 3
2022-12-16 05:27:50.995 main[44172] arr[0][3] = 4
2022-12-16 05:27:50.995 main[44172] arr[1][0] = 5
2022-12-16 05:27:50.995 main[44172] arr[1][1] = 6
2022-12-16 05:27:50.995 main[44172] arr[1][2] = 7
2022-12-16 05:27:50.995 main[44172] arr[1][3] = 8
2022-12-16 05:27:50.995 main[44172] arr[2][0] = 9
2022-12-16 05:27:50.995 main[44172] arr[2][1] = 10
2022-12-16 05:27:50.995 main[44172] arr[2][2] = 11
2022-12-16 05:27:50.995 main[44172] arr[2][3] = 12
2022-12-16 05:27:50.995 main[44172] arr[3][0] = 13
2022-12-16 05:27:50.995 main[44172] arr[3][1] = 14
2022-12-16 05:27:50.995 main[44172] arr[3][2] = 15
2022-12-16 05:27:50.995 main[44172] arr[3][3] = 16
However, with an increase in the dimensions of the array, the complexity also increases as the number of dimensions increases. The most used multidimensional array is the Two-Dimensional Array or hardly three-dimensions array because complexity increases and it becomes quite challenging to handle them.
Share your thoughts in the comments
Please Login to comment...