Spring Boot is an extension of the Spring framework which is built on top of the Servlet. The Spring framework is largely used to build loosely coupled, robust, and standalone Java-based enterprise applications.
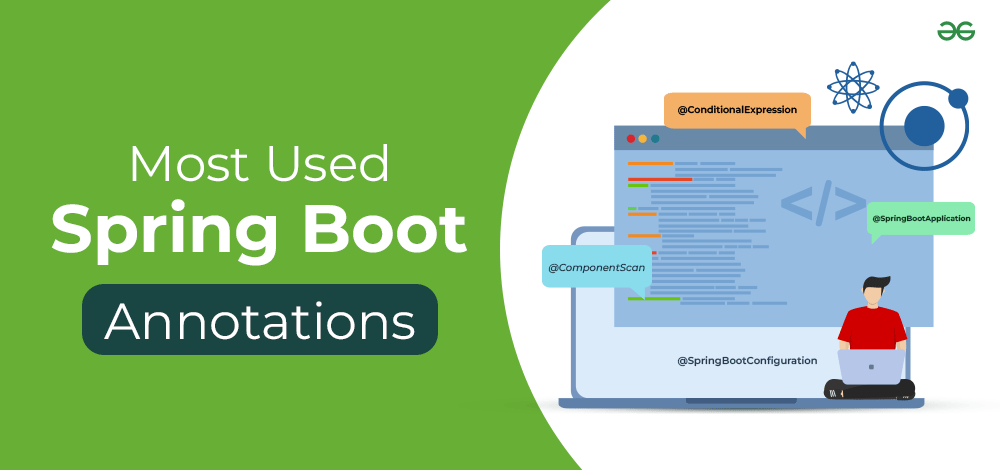
Spring Boot offers a handful of versatile functionalities such as auto-configuration, opinionated defaults, embedded server, vast annotation support, etc.,
What are Spring Boot Annotations?
Spring Boot annotations are the most common functionality that’s effectively utilized to build and develop a software system. These annotations come occupied with a number of features such as – dependency injection, component scanning, controller support, defining REST Endpoints etc.
With this article, we’ll be discussing the Top-10 most commonly used Spring Boot Annotations. Let’s move forward!
Top 10 Most Used Spring Boot Annotations
Now that we are familiar with the basics, we will move forward to our main topic and understand them in detail.
1. @SpringBootApplication
This annotation is used to bootstrap a Spring Boot Application and launch it through the Java main method. It initalizes the application context from the classpath and launches the application. It enables the developer to avoid hectic configuration-related work by providing auto-configuration, component scanning etc. It has the following features :
- Component Scanning for registering the Java Beans with Spring Context.
- Enables auto-configuration for configuring the data source, external dependencies etc.
- Define the Java Beans inside the current class.
This annotation further comprises the following 3 Annotations that allow us to leverage the above-mentioned functionalities.
- @EnableAutoConfiguration: This provides a prominent functionality for configuring the spring boot application by some opinionated defaults that are configured using the dependencies provided in the classpath.
- @ComponentScan: Performs a full context scan of the package & its sub-packages in our directory structure to register classes with Spring Context.
- @Configuration: Annotate a class to indicate that it contains bean definition and that this is a configuration class that has configurable beans defined in it.
Example of @SpringBootApplication
Java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication. class , args);
}
}
|
2. @Configuration
The @Configuration is the annotation of Spring Core that when annotated on top of a class signifies that this class contains definition for various Java Beans annotated with @Bean that will be managed by Spring IoC Container. This annotation also comes occupied with the semantics of @Component that conveys to the spring framework that this class is to be detected in Component Scanning.
Example of @Configuration
Java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyConfig {
@Bean
public MyBean myBean1() {
return new MyBean();
}
}
|
3. @Bean
This is the simplest annotation that’s annotated on top of a method signaling to the Spring Framework that this method contains a definition of Java Bean and will return a Java Bean that has to manage by the Spring IoC Container.
Example of @Bean
Java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyConfig {
@Bean
public User theUser() {
return new User();
}
@Bean
public Admin theAdmin() {
return new Admin();
}
}
|
Code Explanation
In the above example, we’ve created a simple ‘MyConfig‘ class. Note that every class that contains bean definition has to be annotated with @Configuration.
- @Bean is marked on top of the both methods that return a ‘User‘ and ‘Admin‘ Java Beans.
- We can define multiple beans in this class.
4. @Autowired
The sole purpose of this annotation is to facilitate the core feature of Spring Framework – ‘Dependency Injection‘. Dependency Injection is the procedure of attaching one object with another inorder to share data & access each other’s functionality. Let’s first look at simple example to understand its benefits.
Manual Dependency Injection
Let’s say, we have a car and an engine. In order for the car to run we must inject the engine as its dependency. Assuming we have 2 classes – ‘Car’ and ‘Engine’. Here’s how we would do it in pure java :-
Java
public class MyApp {
public static void main(String[] args) {
Engine engine = new Engine();
Car theCar = new Car(engine);
}
}
|
Using @Autowired
Now, look at how the same can be achieved in Spring Boot using @Autowired. (There are multiple ways – using field injection, constructor injection or setter injections.
Java
import org.springframework.beans.factory.annotation.Autowired;
public class Car {
private Engine theEngine;
@Autowired
public Car(Engine theEngine) {
this .engine = theEngine;
}
}
|
Code Explanation
- Annotating the top of the constructor, field or even a setter method signals the spring framework that this field or a constructor is a dependency injection.
- In our example, the engine is the non-dependent class getting injected into car class.
4. @Qualifier
This annotation allows the programmer to be more specific in requesting the beans from the Spring Container. Elaborating this further, when there is one generalised class defined in our spring application and that generalised class has further 3 types or specializations then when we request that generalised class then our Spring container will get confused which class to provide in the response. So it will prompt us that we should be more ‘specific’.
Let’s understand this by following example :
Assuming we have following 2 things defined :
- Interface ‘Teacher’
- Classes that implent Teacher – ‘MathTeacher‘, ‘PhysicsTeacher’, ‘ChemistryTeacher’. (All of these classes will be annotated with @Component – more on this later)
When you ask the Spring framework for a dependency to inject using @Autowired, it will throw you an Exception : NoUniqueBeanDefinitionException, indication that more than one bean is available for autowiring if you are not specific using @Qualifier.
Example of @Qualifier
Java
package com.adwitiya.springcorequalifier.REST;
import com.adwitiya.springcorequalifier.common.Teacher;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class DemoController {
private Teacher myTeacher;
@Autowired
DemoController( @Qualifier ( "mathTeacher" ) Teacher theTeacher) {
this .myTeacher = theTeacher;
}
}
|
Code Explanation
- Above code is a simple Rest Controller which has specified Mappings for performing some operations.
- @Autowired is used to inject the dependency teacher type into the Teacher object.
- We’re using constructor injection to specify which bean we’re requesting of type ‘Teacher‘. In this case, its – “MathTeacher”. Notice that we’re using initial letter in lower case because this is the default in Spring Boot unless explicitly the bean name has changed using @Bean(“BeanName”) Annotation.
5. @Component
This annotation is used to indicate to the spring framework that this class is to be managed by the Spring Container and should be treated as the Bean and Spring Container will create its instance and allow it to be used across various parts of our application using dependency injection.
Taking reference from our previous example (@Qualifier example), we can say that we have a Java Bean – ‘MathTeacher‘ that will be instantiated and managed by the spring framework for dependency injection, when we annotate it with @Component.
Example of @Component
Java
@Component
class MathTeacher implement Teacher {
@Override
public getSchedule(){
return "Study maths for 2 hours" ;
}
}
|
Code Explanation
- We’ve simply defined a MathTeacher Class that’s implementing the Teacher interface.
- For simplicity we’re not including any fields, but there’s a single method annotated with @Override (indicating the same) that can be called by other objects having the MathTeacher as the depency injected.
- @Component here is crucial to registering this bean with spring context, so that it can be automatically injected into other components of our application.
6. @Controller
This is a class-based annotation, basically it marks the class as the ‘Controller‘. A Controller is a crucial component in Spring Framework that knows how to handle the incoming requests and provide a customized response for every request. This annotation is used with the spring MVC along with @RequestMapping which we’ll see later in this article.
Example of @Controller
Java
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class TestController {
@RequestMapping ( "/api" )
public String sayHello()
{
return "Hello Geek!" ;
}
}
|
Code Explanation
- In the above code, we’ve basically created a TestController class, marked it as a controller to handle the requests
- There’s only one method defined for simplicity that can be accessed through the endpoint – ‘https://localhost:8080/api’
7. @RestController
This annotation is used to create RESTful web-services, and is a specialized version of @Controller which we discussed earlier.
This is also a class level annotation that deals with various REST APIs – GET, PUT, POST, DELETE etc. A class is considered to be defining various REST endpoints if it is annotated with @RestController.
It comprises of two sub-annotations – @Controller and @ResponseBody.
@ResponseBody: The default behaviour of a spring application is to assume that, every rest endpoint method will return some view page like HTML or JSP which should be resolved by some view resolver. But, this annotation overrides this default behaviour to – indicating that all the methods in this controller will return some content rather than some view-page.
Example of @RestController
Java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping ( "/api" )
public class TestController {
@GetMapping ( "/hello" )
public String greet() {
return "Hello Geek!" ;
}
}
|
Code Explanation
- We’ve defined a simple test Rest controller that contains a REST endpoint – /api/hello.
- When you run it on localhost:8080 (default port) you’ll get the output as – ‘Hello Geek!‘.
- Note: The method name can be anything here, what matters is the endpoint defined must be know to access a certain resource.
8. @RequestMapping
This annotation can used on a class level as well as method level for defining the path of URL which is our endpoints. It is the core annotation to define the path which will return some response containing the resource requested by the client. Although it is preferred only at class level because methods are supposed to perform a one single task and that should be annotated with something more meaning full, so we use @GetMapping, @PostMapping etc for such purposes.
We can take reference from previous example for reference. Controller is annotated with @RequestMapping and method is annotated with @GetMapping to indicate that this method is only returning a resource.
9. @PathVariable
This is a method level annotation which allows us to be more specific based on context and fetch a more specific resource. For example, we might need the data of student with id = 5. Then we can specify the same in the URL while trying to fetch the resource.
@PathVariable is used to pass & extract parameters from the endpoint to the method parameters and then perform operations on it.
Example of @PathVariable
Java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ProductController {
@GetMapping ( "/api/product/{id}" )
public Product getProduct( @PathVariable Long id) {
return productService.getProductById(id);
}
}
|
Code Explanation
- Assuming we have a Product class as POJO already defined and its service layer as well (productService – object).
- When client hits the endpoint- /api/product/{id} the {id} will contain a positive integer to specify the product with a specific id. It would look something like – /api/product/5. This indicates that the client is requesting the product with id = 5. This digit can vary depending on the client’s request.
10. @Repository
This annotation is a class level annotation that signifies that this class is a data repository or Data Accessor Object (DAO) that interacts with the database and perform CRUD Operations.
It is also considered a specialization of @Component that means a class annotated with @Repository is also implicitly annotated with @Component and will be managed by the Spring IoC Container.
Example of @Repository
Java
import org.springframework.stereotype.Repository;
@Repository
public class UserRepository {
}
|
Conclusion
In conclusion, Spring Boot offers us with a powerful toolkit comprised with a handful of annotations, which assist in programming an application in numerous ways. These annotations have become a building block for writing back-end code using Spring Boot that helps us in build robust, stand-alone and portable application by leveraging the full power of spring framework.
- These annotations can be further classified into various types: Configuration annotation, Component Annotation, Dependency Injection related Annotations etc.
- Annotations helps in structuring and improving our code readability as well as promoting best practices.
- We’ve discussed top 10 most commonly used Spring Boot annotations that you will come across whether you’re a beginner or you’re writing code for a large-scale web application.
Share your thoughts in the comments
Please Login to comment...