Loading Multiple Scripts Dynamically in Sequence using JavaScript
Last Updated :
13 Jul, 2022
In this article, we will see how to insert multiple JavaScript files dynamically in the intended execution order by creating <script> elements for each of them and inserting them into DOM. For this, the concept of Promise & async() function will be utilized to accomplish this task,
Promise Object: A Promise can be fulfilled with a value or rejected with an error(reason). It represents the completion (or failure) of an asynchronous operation and its value. When either of the options happens, then or catch method is called.
Async Function: It enables asynchronous promise-based behavior. Also, the use of try/catch blocks is enabled using the async function. In simple words, Asynchronous calls the next statement gets executed without even waiting for the previous one’s execution.
Syntax:
let scriptList = [
/*'scriptName1',
'scriptName2', //Define script names to load
...........*/
];
scriptList.forEach(function(a){ //loop to iterate over each script file.
let script = document.createElement('script'); //creating <script> element
script.src = a;
script.async = false;
document.body.appendChild(script);
});
Approach: The JavaScript files which need to be loaded dynamically will be stored in an array named multipleScript.Then, we will iterate over each JS file and create <script> elements for each of them. The order of execution will not be saved if we don’t set the value of async as false. Otherwise, the browser automatically set this attribute to true. To detect whether all scripts have finished loading, onload event is used that occurs when an object has been loaded. We will use the concept of promise to know whether all scripts are loaded or not.
Example: In this example, we need to load 3 Javascript files dynamically in the order given by the user. According to the user, any one of the three javascript files can be used without connecting the JS file with an HTML file. If all scripts are loaded successfully, the message of success will be logged in the console else an error message will be logged.
HTML
< html >
< head >
< title >
Loading Multiple Scripts Dynamically in Javascript
</ title >
</ head >
< body >
< h1 id = "head" >Welcome to GeeksforGeeks</ h1 >
< script >
let create = (info) => {
return new Promise(function(resolve, reject) {
let gfgData = document.createElement('script');
gfgData.src = info;
gfgData.async = false;
gfgData.onload = () => {
resolve(info);
};
gfgData.onerror = () => {
reject(info);
};
document.body.appendChild(gfgData);
});
};
let gfgScript = ['firstscript.js',
'secondscript.js',
'thirdscript.js'];
let promiseData = [];
gfgScript.forEach(function(info) {
promiseData.push(create(info));
});
Promise.all(promiseData).then(function() {
console.log('The required scripts are loaded successfully!');
}).catch(function(gfgData) {
console.log(gfgData + ' failed to load!');
});
</ script >
</ body >
</ html >
|
firstscript.js:
let head = document.getElementById("head");
head.style.color = 'green';
Output:
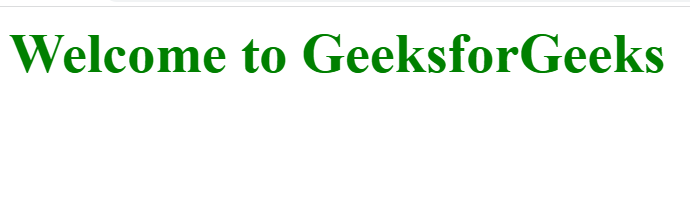
Loaded firstscript.js successfully
secondscript.js:
let head = document.getElementById('head');
head.innerText = "Thank You!";
Output:
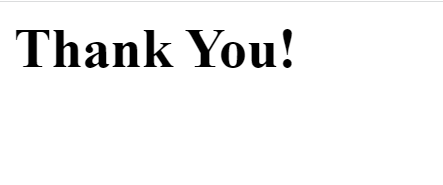
Loaded secondscript.js successfully
thirdscript.js:
let head = document.getElementById("head");
head.style.fontStyle="italic";
Output:

Loaded thirdscript.js successfully
Explanation: Promise is resolved when the script is loaded and rejected when loading fails. All the promises of scripts are stored in an array and passed as parameters to Promise.all(). It returns a Promise when all input Promises have been resolved. If any of the input Promises is rejected it is rejected completely.
The following message will be logged in the console if all 3 JS files are loaded sequentially.
If the names of the JavaScript file are declared wrongly or the file doesn’t exist on the local machine, the following errors will be logged in the console. Instead of declaring ‘firstcsript.js‘, we have written ‘fisrtscript.js‘.Thus, it failed to load.
- If the JS file is not available on the local machine:
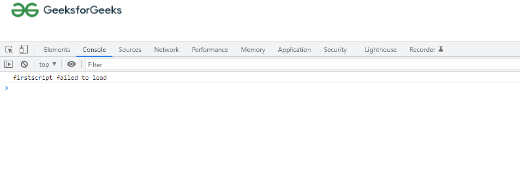
Failed as the file doesn’t exist
- JS file name is wrongly defined:
Share your thoughts in the comments
Please Login to comment...