Less.js String Functions
Last Updated :
28 Sep, 2022
Less.js (Leaner Style Sheets) is an extension to normal CSS which basically enhances the abilities of the normal CSS and gives it programmable powers like variables, functions, loops, etc.
String functions are one such type of function provided by Less.js to perform operations on a string like replacing a substring, URL encoding, formatting a string, etc.
There are 4 functions provided by Less.js which come under the category of String Functions, and they are as follows:
1. escape: This function is used to apply URL encoding to the special characters like “#”, “=”, “(“, etc found in the given string. Takes only one argument which is the input string and returns the encoded string as output.
The characters which are not encoded by this function are : ,, /, ?, @, &, +, ‘, ~, ! and $ .
Syntax:
escape(string)
Parameter:
- string: The string to apply URL encoding on.
Note: If the given argument is not a string, then the output returned is undefined if the argument is color value else the value as it is. This behavior must not be relied upon and may change in the future.
2. e: This function is used to basically remove quotes from a string and return its content without quotes as it is. Take only one argument that is the input string and returns the escaped string (without quotes).
Syntax:
e(string)
Parameter:
- string: The string to escape.
3. % format: This function is used to format a string. Basically “%(string, arguments …)“. The first argument is the input string with placeholder values (places that need to be replaced). Then the following arguments will be equal to the number of times placeholders have been used in the given string.
Syntax:
%(string, arguments...)
Parameters:
- string: A string with placeholder values.
- arguments: Tells what to fill in place of those placeholder values. The number of arguments is equal to the number of placeholder values present in the string.
Placeholders:
- a, A, d, D: These placeholders can be used to replace any kind of argument like color, number, string, expression, etc. In case the corresponding argument is a string then the whole string with quotes included is replaced in the place of a placeholder.
- s, S: These placeholders can be used to replace any kind of argument like color, number, string, expression, etc. In case the corresponding argument is a string then the content of the string (that is string without quotes) is replaced in the place of a placeholder.
Placeholder values start from “%” symbol and are followed by the characters : “a”, “A”, “d”, “D”, “s” and “S”.
If there is a “%” symbol in the string which is not considered for the purpose of being a placeholder then you can use “%%” instead of a single “%”.
Uppercase placeholders are used when you want that the string present in the “arguments” first needs to be escaped which is escaping the special characters into their UTF-8 special codes. The function escapes all characters except for the characters: ()’~! .
4. replace: This function is used to replace a sub-string from the given string. This function can accept 4 arguments out of which 3 are necessary and the 4th one is optional. The first three necessary arguments are string, pattern to replace, replacement string and the 4th optional argument is regular expression flags.
Syntax:
replace(string, pattern, replacement, flags)
Parameters:
- string: A string value whose text has to be replaced.
- pattern: A string/regular expression to search for within the string.
- replacement: The sub-string or text which needs to be replaced in place of the given pattern found within the string.
- flags (optional): The flags used in the regular expression. For example: “g”, “i”, etc.
Example 1: In this code example, we have 2 variables namely “@primaryColor” and “@fontFamily”, but they are in string format and we want their value to be used without quotes hence we use the “e” function to remove the quotes.
Then, we used the “%” function to add 2 strings in the selector “h3::after” to make its content property equal to the value of adding the two strings that are normal “Hello=World+GeeksForGeeks” an the escaped version of the string “Hello=World+GeeksForGeeks” and then display it in the webpage.
index.html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >GeeksForGeeks | String Functions in Less.js</ title >
< link rel = "stylesheet" href = "/styles.css" >
</ head >
< body >
< h1 >GeeksForGeeks</ h1 >
< h3 >String Functions in Less.js</ h3 >
</ body >
</ html >
|
styles.less
@primaryColor: "springgreen" ;
@fontFamily: "sans-serif" ;
body {
font-family : e(@fontFamily);
}
h 1 {
color : e(@primaryColor);
}
h 3: :after {
content : %(
"Hello=World+GeeksForGeeks : %s" ,
escape( "Hello=World#GeeksForGeeks" )
);
color : white ;
background-color : black ;
}
|
Now, to compile the above LESS code into normal CSS code, run the following command:
lessc styles.less styles.css
The compiled CSS file comes out to be:
styles.css
body {
font-family : sans-serif ;
}
h 1 {
color : springgreen;
}
h 3: :after {
content :
"Hello=World+GeeksForGeeks : Hello%3DWorld%23GeeksForGeeks" ;
color : white ;
background-color : black ;
}
|
Output:
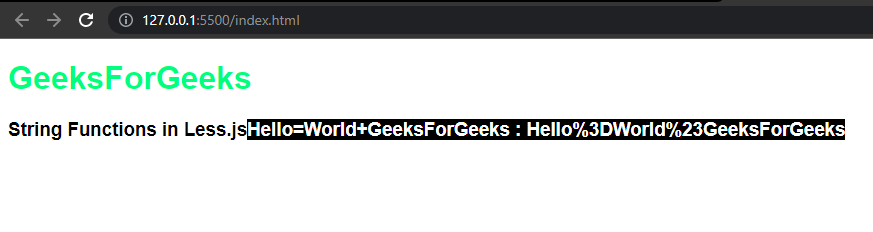
output of string function in Less.js example 1
Example 2: In this example, we have a format of string and we have the name. Using the replace function we replace the “name” from the format and replace it with the given name.
Also, we have a format of specifying a border in which we replace the placeholder value that is “%a”, “%s” and “%s” with the values “2”, “solid” and “black”.
index.html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >GeeksForGeeks | String Functions in Less.js</ title >
< link rel = "stylesheet" href = "/styles.css" >
</ head >
< body >
< h1 >GeeksForGeeks</ h1 >
< h3 >String Functions in Less.js</ h3 >
< p ></ p >
</ body >
</ html >
|
styles.less
@name: "GeeksForGeeks" ;
@format: "name | String Functions " ;
@myBorder: %( "%apx %s %s" , 2 , "solid" , "black" );
h 1 {
width : max-content;
border : e(@myBorder);
}
p::after {
content : replace(@ format , "name" , @name);
}
|
Now, to compile the above LESS code into normal CSS, run the following command:
lessc styles.less styles.css
The output CSS file comes out to be:
styles.css
h 1 {
width : max-content;
border : 2px solid black ;
}
p::after {
content : "GeeksForGeeks | String Functions " ;
}
|
Output:
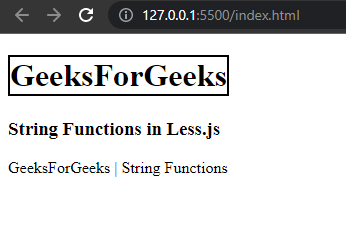
the output of string function in Less.js example 2
Reference: https://lesscss.org/functions/#string-functions
Share your thoughts in the comments
Please Login to comment...