Less.js (Leaner Style Sheets) is an extension to normal CSS which basically enhances the abilities of the normal CSS code and gives it more functionalities and programmable features.
Less.js provides us with many List Functions which are basically functions that we can perform on a list of elements like finding the length of the list, extracting an element, etc. And in this post, we are going to deep dive into the topic of List functions.
List Functions in Less.js: Less.js comes with 4 functions that come under the category of List Functions and can be performed on a list. The List Functions are as follows:
1. length: Take a list (comma/space separated values) as an argument and returns the number of elements present in the list.
Syntax:
length(list)
Parameter:
- list: A list of values that is comma/space-separated or a variable storing a list. For example: 1px solid black, , @myList etc.
2. extract: Used to extract an element using its index value from a list. This function takes 2 arguments which are a list and the index to fetch. (index value starts from 1 to the length of the list).
Syntax:
extract(list, index)
Parameters:
- list: A list of values that is comma/space-separated or a variable storing a list. For example: 1px solid black, , @myList etc.
- index: The position of the element which you want to extract. The index value lies between 1 and the length of the list. For example: 1, 3, 2 etc.
3. range: This function is used to generate a list containing a range of values This function takes 3 arguments that are start value (if not provided then equal to 1), end value, and step value (if not provided then equal to 1). The output of each value in the range will be of the same unit as that of the end value.
Syntax:
range(start_value, stop_value, step_value)
Parameters:
- start_value (optional): The starting value of the list. For example: 1, 10px etc.
- end_value: The ending value of the list. For example 10, 50px, etc.
- step_value: The value by which the start value must be incremented. For example: 1, 10 etc.
Note: The unit of end_value will be considered as the unit of all the elements of the output list.
4. each: This function is basically used to bind a particular ruleset with each member of a list (This function is used kind of like a for loop). It takes 2 arguments which are a list (comma/space separated values) and an anonymous ruleset/mixin. The function gives us 3 variables to be used inside the 2nd argument that is @value (which stores the value of the element), @index (which stores the index position of that element), and @key (which in the case of a normal list is equal to @index and in the case of a ruleset store the value of key).
Syntax:
each(list, rules)
Parameters:
- list: a list (comma/space separated values) value. Also, you can use the rulesets themselves as structured lists. For Example: @myList = 1px solid black , @mySet = { one: 1, two: 2 } etc
- rules: Basically an anonymous ruleset or a mixin.
Example syntax of using each function:
each(@myList, {
.list-@{value}{
@{key}-@{index}: @value;
}
})
See that the @key and @index variables are written as @{key} and @{index}. And also the same thing has been used in the “.list-@{value}” for @value as well.
Basically, you need to wrap up the variables inside curly braces whenever they are not used as values like in the case of “@{key}-@{index}: @value”, @value is being used as a value for the property “@{key}-@{index}”.
You can also change the name of the variables “@key”, “@index” and “@value” using the below syntax:
each( @myList, .( @myvalue, @mykey, @myindex ){
@{mykey}-@{myindex}: @myvalue;
} )
The order of the variables must remain the same. If you, for example, only write (@myvalue) then @key and @index variables will not be defined.
Note: In place of .(@myvalue, @mykey, @myindex) , you can also write #(@myvalue, @mykey, @myindex). Basically, you can use a “#” in place of “.” as well.
Examples of List Functions in Less.js:
Example 1: In this example, we have made 4 lists that are @sections, @colors, @sizes (which are made using the range function), and @families.
We have calculated the number of sections using the length function on the list @sections and then made the width of the “.section” class equal to “1000px/number of sections” so that every section has the same width.
Using the range function we have defined the @sizes list. The sizes becomes 15px, 20px, 25px and 30px as the range function has step value of 5px and hence it increments the start value by 5px until the end value comes.
Then, using the extract function we have provided values to various properties inside the body, “.section”, h1, and h3 selectors.
index.html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >GeeksForGeeks | List Functions in Less.js</ title >
< link rel = "stylesheet" href = "/styles.css" >
</ head >
< body >
< div class = "section" >
< h1 >GeeksForGeeks</ h1 >
< h3 >List Functions in Less.js</ h3 >
</ div >
</ body >
</ html >
|
styles.less
@sections: "section1" , "section2" , "section3" ;
@noOfSections: length(@sections);
@colors: green , springgreen, black , red ;
@sizes: range( 15px , 30px , 5px );
@families: serif , sans-serif , monospace , cursive ;
body {
font-family : extract(@families, 2 );
}
.section {
width : ( 1000px / @noOfSections);
background-color : extract(@colors, 3 );
}
h 1 {
color : extract(@colors, 2 );
font-size : extract(@sizes, length(@sizes));
}
h 3 {
color : extract(@colors, 4 );
font-size : extract(@sizes, 2 );
}
|
Now, to compile the above LESS code to CSS code, run the following command:
lessc styles.less styles.css
The compiled CSS file comes to be:
styles.css
body {
font-family : sans-serif ;
}
.section {
width : 333.33333333px ;
background-color : black ;
}
h 1 {
color : springgreen;
font-size : 30px ;
}
h 3 {
color : red ;
font-size : 20px ;
}
|
Output:
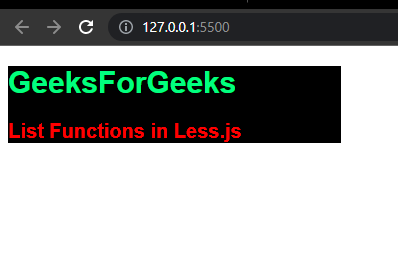
The output of List Functions in Less.js Example 1
Example 2: In this example, we have defined font sizes using range function from 10px to 30px with a step value of 5px.
Then using “each” function, we have defined 5 classes ranging from “font-1” class with font size of “10px” to “font-5” class with font size of “30px”.
Then we have added the class “font-1” to body tag to make the default font size equal to 10px, “font-2” class to h3 tag to make it’s font size equal to “15px” and “font-5” class to h1 tag to make it’s font size equal to “30px”.
index.html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >GeeksForGeeks | List Functions in Less.js</ title >
< link rel = "stylesheet" href = "/styles.css" >
</ head >
< body class = "font-1" >
< h1 class = "font-5" >GeeksForGeeks</ h1 >
< h3 class = "font-2" >List Functions in Less.js</ h3 >
< p >Lorem ipsum, dolor sit amet consectetur
adipisicing elit. Libero, quo!</ p >
</ body >
</ html >
|
style.less
@sizes: range( 10px , 30px , 5 );
each(@sizes, {
.font -@{ index }{
font - size : @value;
}
})
|
To compile the above LESS code to normal CSS code, run the following command:
lessc styles.less styles.css
The compiled CSS file comes out to be:
styles.css
.font -1 {
font-size : 10px ;
}
.font -2 {
font-size : 15px ;
}
.font -3 {
font-size : 20px ;
}
.font -4 {
font-size : 25px ;
}
.font -5 {
font-size : 30px ;
}
|
Output:
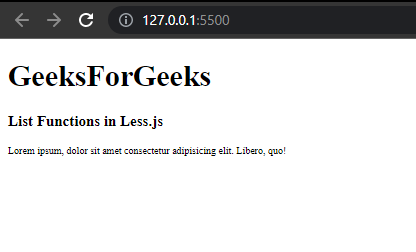
The output of List Function in Less.js Example 2
Example 3: In this example, we have defined the text color classes “text-primary”, “text-secondary”, “text-dark” and “text-light” along with the background color classes “bg-primary”, “bg-secondary”, “bg-dark” and “bg-light” using the “@colors” ruleset.
Also, we have given our custom names to the variables “@value”, “@key” and “@index”.
index.html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >GeeksForGeeks | List Functions in Less.js</ title >
< link rel = "stylesheet" href = "/styles.css" >
</ head >
< body class = "bg-dark text-light" >
< h1 class = "text-primary" >GeeksForGeeks</ h1 >
< h3 class = "text-secondary" >List Functions in Less.js</ h3 >
< p class = "text-dark bg-light" >
Lorem ipsum, dolor sit amet consectetur
adipisicing elit. Libero, quo!</ p >
</ body >
</ html >
|
style.less
@colors: {
primary: springgreen;
secondary: yellow;
dark: black ;
light: white ;
}
each(@colors, .(@v, @k, @i) {
.bg -@{ k }{
background - color : @v;
}
.text -@{ k }{
color : @v;
}
})
|
To compile the above LESS code to normal CSS code, run the following command:
lessc styles.less styles.css
The compiled CSS file comes out to be:
style.css
.bg-primary {
background-color : springgreen;
}
.text-primary {
color : springgreen;
}
.bg-secondary {
background-color : yellow;
}
.text-secondary {
color : yellow;
}
.bg-dark {
background-color : black ;
}
.text-dark {
color : black ;
}
.bg-light {
background-color : white ;
}
.text-light {
color : white ;
}
|
Output:
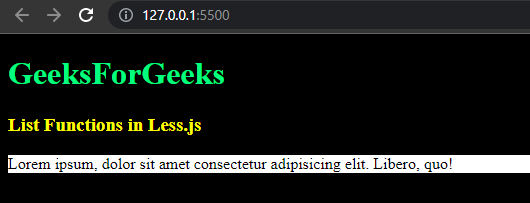
The output of List Function in Less.js Example 3
Reference: https://lesscss.org/functions/#list-functions
Share your thoughts in the comments
Please Login to comment...