Less.js Color Blending Functions
Last Updated :
16 Oct, 2022
In this article, we are going to see Color Blending Functions in Less.js.
Less (Leaner Style Sheets) is an extension to normal CSS code which basically enhances the abilities of normal CSS and provides it superpowers.
Color blending functions are provided by Less.js to basically perform blending operations on colors and get the output.
There are 9 Color Blending functions provided by Less.js which are as follows:
1. multiply: This function is used to multiply two colors and return the output. Basically, the corresponding RGB channels of both colors are multiplied and then divided by 255. The output color is a darker color.
Syntax:
multiply(color1, color2)
Parameters:
- color1: A color object. For example: brown, RGB(13, 13, 13) etc
- color2: A color object. For example: brown, RGB(13, 13, 13) etc
2. screen: This function is basically used to do the opposite of the multiply function and hence, the resultant output color is a brighter color.
Syntax:
screen(color1, color2)
Parameters:
- color1: A color object. For example: brown, RGB(13, 13, 13) etc
- color2: A color object. For example: brown, RGB(13, 13, 13) etc
3. overlay: This function is used to basically combine the effects of both the multiply and screen functions. This function makes the light channels lighter and the dark channels darker. The result is determined by the first color argument.
Syntax:
overlay(color1, color2)
Parameters:
- color1: A base color object which determines whether the output color would be lighter or darker.
- color2: A color object to overlay.
4. softlight: This function is basically the same as the overlay function, the difference being that it avoids the resultant on pure black being pure black and on pure white being pure white.
Syntax:
softlight(color1, color2)
Parameters:
- color1: A color object to soft light another. For example: brown, RGB(13, 13, 13) etc.
- color2: A color object to soft and light. For example: brown, RGB(13, 13, 13) etc.
5. hardlight: This function is the same as the overlay function, the difference being that color2 becomes the base color and color1 becomes the color to be overlayed.
Syntax:
hardlight(color1, color2)
Parameters:
- color1: A color object to overlay.
- color2: A base color object which determines whether the output color would be lighter or darker.
6. difference: This function is used to subtract color2 from color1 on a channel-by-channel basis. The negative values are inverted. Subtracting black color results in no difference and white color results in inversion of the color.
Syntax:
difference(color1, color2)
Parameters:
- color1: A color object which acts as the minuend.
- color2: A color object which acts as the subtrahend.
7. exclusion: This function is similar to the different functions, the difference being that the output is having a lower contrast.
Syntax:
exclusion(color1, color2)
Parameters:
- color1: A color object which acts as the minuend.
- color2: A color object which acts as the subtrahend.
8. average: This function is used to find the average of two colors by averaging the corresponding RGB channels and returning the output color.
Syntax:
average(color1, color2)
Parameters:
- color1: A color object. For example: brown, RGB(13, 13, 13) etc.
- color2: A color object. For example: brown, RGB(13, 13, 13) etc.
9. negation: This function does the opposite of the difference function and return a brighter color as the output.
Syntax:
negation(color1, color2)
Parameters:
- color1: A color object which acts as the minuend.
- color2: A color object which acts as the subtrahend.
Example 1: In this example, we have defined two colors namely @primary and @secondary. Now using these two colors we perform different various color blending functions and apply them to the HTML.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >GeeksforGeeks |
Color Blending Functions in Less.js</ title >
< link rel = "stylesheet" href = "/styles.css" >
</ head >
< body style = "font-family: sans-serif;" >
< h1 style = "color: green;" >GeeksforGeeks</ h1 >
< h3 >< b >Color Blending Functions in Less.js</ b ></ h3 >
< p class = "multiply" >Multiply</ p >
< p class = "overlay" >Overlay</ p >
< p class = "difference" >Difference</ p >
< p class = "average" >Average</ p >
< p class = "negation" >negation</ p >
</ body >
</ html >
|
styles.less: The LESS code is as follows.
CSS
// styles.less
@primary: springgreen;
@secondary: brown;
.multiply {
color : multiply(@primary, @secondary);
}
.overlay {
color : overlay(@primary, @secondary);
}
.difference {
color : difference(@primary, @secondary);
}
.average {
color : average(@primary, @secondary);
}
.negation {
color : negation(@primary, @secondary);
}
|
Now, to compile the above LESS code to CSS code, run the following command:
lessc styles.less styles.css
The compiled CSS file comes to be:
styles.css:
CSS
.multiply {
color : #002a15 ;
}
.overlay {
color : #00ff2a ;
}
.difference {
color : #a5d555 ;
}
.average {
color : #539455 ;
}
.negation {
color : #a5d5a9 ;
}
|
Output:
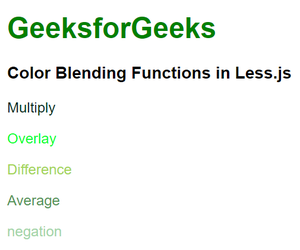
the output of color blending functions in less.js example 1
Example 2: In this example, we have two colors namely @color1 which is formed using the screen function on pink and brown colors, and @color2 which is formed using the exclusion function on pink and brown colors. Then we have made 2 classes namely “hardlight” and “softlight” which basically perform hardlight() and softlight() functions respectively on the two colors formed and apply them to the HTML.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >GeeksforGeeks |
Color Blending Functions in Less.js</ title >
< link rel = "stylesheet" href = "/styles.css" >
</ head >
< body style = "font-family: sans-serif;" >
< h1 style = "color: green;" >GeeksforGeeks</ h1 >
< h3 >< b >Color Blending Functions in Less.js</ b ></ h3 >
< p class = "hardlight" >I am hardlight</ p >
< p class = "softlight" >I am softlight</ p >
</ body >
</ html >
|
styles.less: The LESS code is as follows.
CSS
// styles.less
@color 1: screen (pink, brown);
@color 2: exclusion(pink, brown);
.hardlight {
background-color : hardlight(@color 1 , @color 2 );
}
.softlight {
background-color : softlight(@color 1 , @color 2 );
}
|
Now, to compile the above LESS code to CSS code, run the following command:
lessc styles.less styles.css
The compiled CSS file comes to be:
styles.css:
CSS
.hardlight {
background-color : #b4dce5 ;
}
.softlight {
background-color : #ffd3dc ;
}
|
Output:
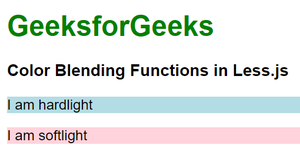
output of color blending functions in less.js example 2
Reference: https://lesscss.org/functions/#color-blending
Share your thoughts in the comments
Please Login to comment...