In the cloud computing revolution, AWS Lambda stands out as a key solution from Amazon Web Services (AWS), allowing developers to easily implement code without the burden of monitoring the check server. In this ecosystem, Lambda Handler becomes important. This handler acts as the gateway to the Lambda function, orchestrating the flow and defining the function’s behavior when it occurs. In this article, we will learn about Python Lambda handler in detail.
What Is AWS Lambda?
AWS Lambda is a serverless compute technology service provided by Amazon Web Services to execute a certain code whenever a certain event occurs. It is an auto-scaling technology which auto-scales its resources dynamically according to the incoming traffic. AWS Lambda supports multiple languages, Python, Node.js, Java, Ruby and .NET.
Lambda functions also support multiple versions of python, such as Python 3.12, Python 3.11, Python 3.10 etc.
What Is Lambda Handler In Python?
The Lambda Handler in Lambda Functions refer to the entry point. Just like the “main” method is an entry point in Java and C++ files, Lambda function’s file contains a method called lambda_handler() which serves as the entry point whenever the lambda function is invoked. Lambda Handler is the method that defines the behavior of your lambda function.
Lambda handler can be named anything, but the default name is always ‘lambda_handler()‘. The lambda handler is always stored in the ‘lambda_function.py’ file. It also contains two arguments, ‘event’ and ‘context’.
Implementation Of Lambda Handler In Python Through AWS Console: A Step-By-Step Guide
Step 1: Log In into your AWS Account
Step 2: Click on Services and search ‘Lambda’ and click on the first option you get.
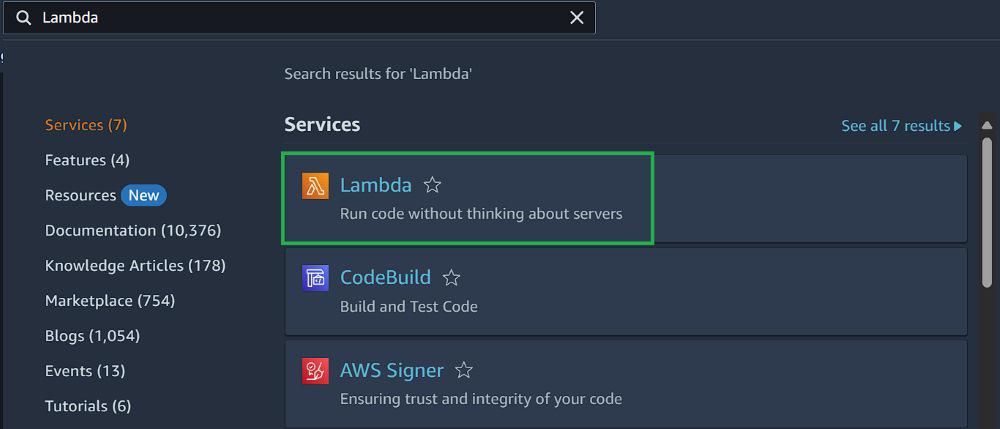
Step 3: Now, click on the ‘Create function’ button to create a Lambda Function.
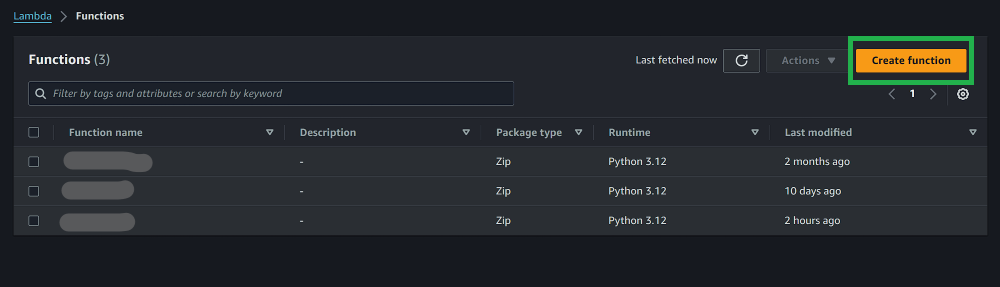
Step 4: After clicking on create function, you will be redirected to a website, where you can create your function. Write down the name of the function and click on the drop-down menu to select your function’s runtime. In the menu you will get a lot of runtime options to select, but we will choose python 3.12 as the runtime.
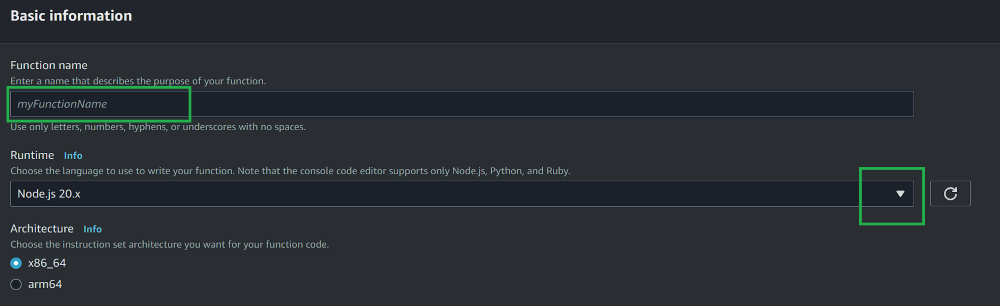
Step 5: Click on the ‘Create function’ after setting all things up.
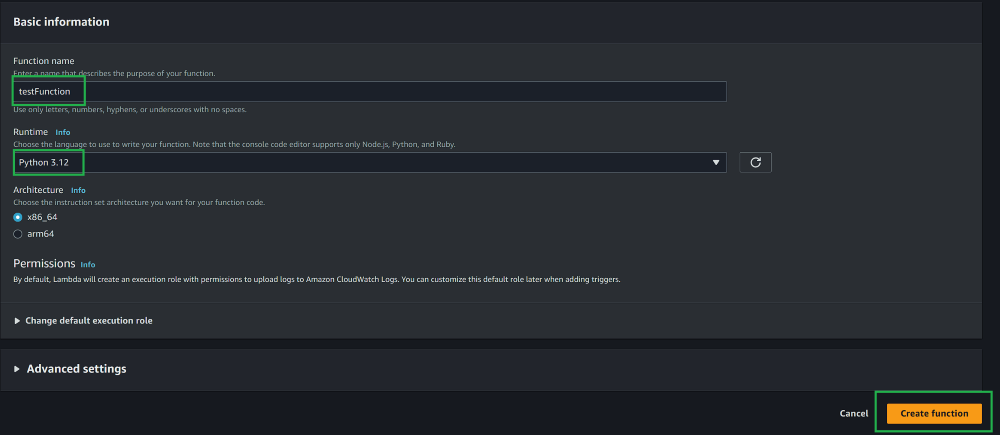
- After this you will be redirected into your function console where you can configure your lambda function and add your code. In this console you will also notice an editor with lambda_handler.py file. This is your function’s main code.
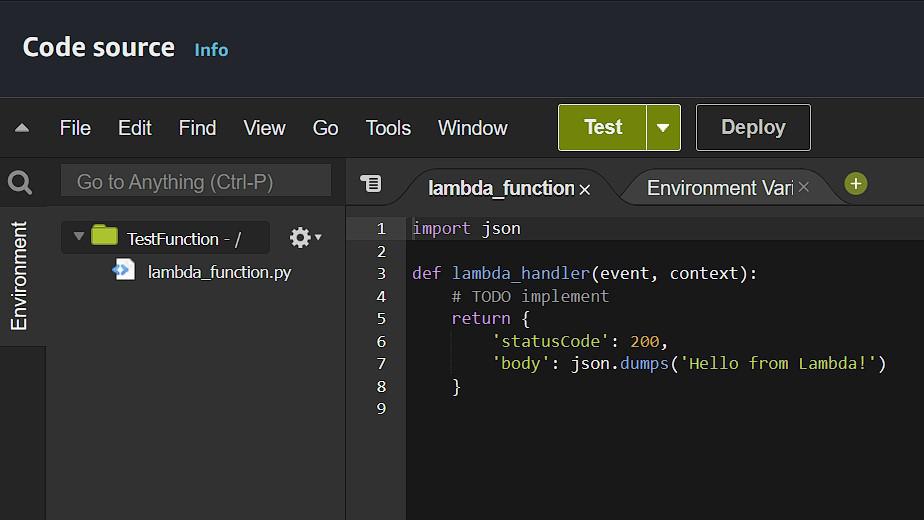
- Here is the default format of the lambda_function.py file.
Python
import json
def lambda_handler(event, context):
# TODO implement
return {
'statusCode': 200,
'body': json.dumps('Hello from Lambda!')
}
- We can see that the entry point ‘lambda_handler(event, context)’ describes the behavior of the function which only returns a JSON string with ‘StatusCode’ and a ‘body’ key.
Terms Related To AWS Lambda Handler In python
def lambda_handler()
- This is the handler function’s default name. It serves as the entry point in the Lambda function file. You can customize the name of your Lambda Handler function but you need to tell AWS Lambda that I have changed my function name. It can be done like this.
- Scroll down to the Runtime Setting tab inside the Lambda console and click on edit.

- A new window will appear from which you can change your Lambda Handler function’s name.
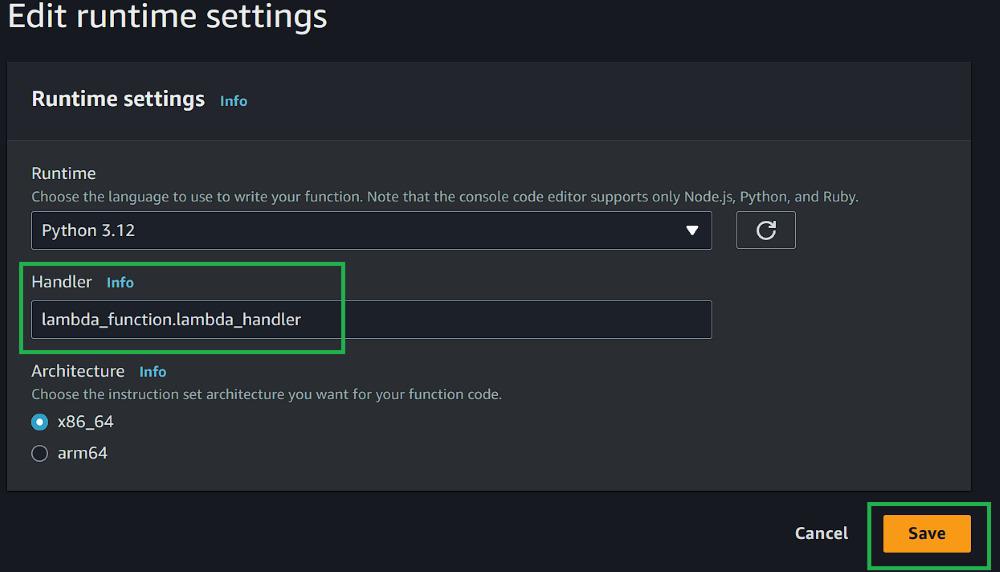
Note: Here lambda_function.lambda_handler is given. The ‘lambda_function’ specifies the file name ‘lambda_function.py’ and the function name is after the ‘dot’ operator. So, just change the name after the ‘dot’ operator to customize the name of the Lambda Handler function.
Event Object:
- AWS Lambda handler function contains two arguments, event and context as seen in the above default code of lambda_function.py file.
- An event is generally a JSON Formatted String that contains the data that was passed by the external sources when invoking a Lambda function. It is usually of the ‘dict’ type in python but it can also be other types, such as ‘list’, ‘int’, ‘float’, etc. The Lambda Handler function generally uses this object to get the data which it can process to generate output. An event object looks like this.
{
"key1": "value1",
"key2": "value2",
"key3": "value3"
}
- From this event, Lambda Handler can easily extract values from the keys to process the data.
Context Object: The context object is passed into the lambda handler method and usually contains methods and properties that provide information about function, invocation and runtime environment. Here are some examples of context methods and properties.
Context methods: get_remaining_time_in_millis() : returns the time in milliseconds left before the lambda function times out.
Context properties:
Context Properties
| Use
|
---|
function_name
| The name of lambda function.
|
---|
function_version
| The version of the function which is being executed.
|
---|
invoked_function_arn
| The Amazon Resource Name (arn) that’s used to invoke the function.
|
---|
memory_limit_in_mb
| The amount of memory that is allocated to the lambda function.
|
---|
aws_request_id
| The identifier of the invocation request.
|
---|
log_group_name
| The log group for the function
|
---|
log_stream_name
| The log stream for the function instance
|
---|
Flow of Lambda Handler
The following ones explains and details about the flow of lambda handler in sections wise:
- Extracting Data From Event : The first and foremost step for lambda handler is to extract the data from the event object from the lambda function. Considering the event example given previously, we can extract the values using these statements:
Python
value_1 = event['key1']
value_2 = event['key2']
value_3 = event['key3']
This will store all the three values in value_1, value_2 and value_3 respectively.
The Main function body: After extracting the input from the event object, the main body of function executes. This defines the behavior of our lambda function. The main body of lambda functions can also call other user-defined functions inside the file. The main body works on the extracted input and processes the data and formulates the result as wanted.
Continuing the previous example, suppose we want out lambda function to concatenate all three values obtained from event object. We will also see the demonstration of some context methods and properties inside the function body. So, our main body will be something like this:
# Calculating the concatenate value for the input strings from event object
result = value_1 + value_2 + value_3
# Demonstration of the contex methods and properties
remaining_time = context.get_remaining_time_in_millis()
fun_name = context.function_name
fun_version = context.function_version
memory = context.memory_limit_in_mb
Return Statement: Finally, we will return the value of the result obtained from the lambda function. This data will be sent back to the source which called the lambda function. The return type is also usually a JSON String though not compulsory. Return statements are written like this:
return {
"StatusCode": 200,
"Concat_value": result,
"Remaining time": remaining_time,
"Function Name": fun_name,
"Function Version": fun_version,
"Memory Used": memory
}
- With this the whole lambda handler is complete.
import json
def lambda_handler(event, context):
value_1 = event['key1']
value_2 = event['key2']
value_3 = event['key3']
# Concatenating values
result = value_1 + value_2 + value_3
# demonstrating context methods and properties
remaining_time = context.get_remaining_time_in_millis()
fun_name = context.function_name
fun_version = context.function_version
memory = context.memory_limit_in_mb
return {
"StatusCode": 200,
"Concat_value": result,
"Remaining time": remaining_time,
"Function Name": fun_name,
"Function Version": fun_version,
"Memory allocated": memory
}
Output:
- On deploying the lamda function, it generate the response as shown in the figure. If the function deployed successful then it print status code as 200 or else based on the error it generates respective status code.
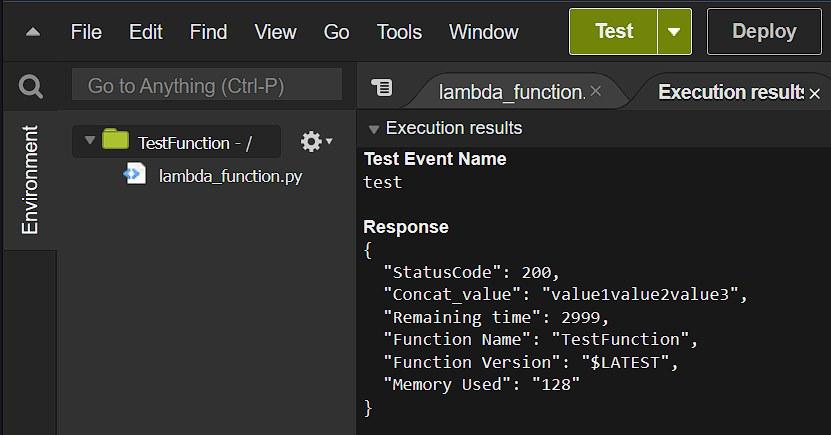
Python Modules
- AWS Lambda handler can import some python modules but not all as AWS Lambda supports only a handful of libraries to import. These libraries are:
- http
- os
- json
- datetime
- random
- logging
- re
- time
- If you want to use your custom libraries in AWS Lambdas then AWS Lambda Layers is the solution. It allows you to import your libraries which are under 250mb.
Conclusion
In conclusion, lambda_handler() is a method in the lambda_function.py class of AWS Lambda function which serves as an entry point of the lambda function and describes the behavior of the lambda function, that is, what it will be doing, what data it will be processing and how it will process the data and return it. The common flow of the lambda function is Extracting Data -> Process Data -> Return Data.
AWS Lambda Function Handler – FAQ’s
Can I Define Multiple Lambda Handler Functions In Python?
No, you cannot define multiple Lambda Handler functions in python. Lambda service requires you to specify the exact function to run whenever the function is invoked.
Can I Customize The Name Of My Handler Function?
Yes, you can customize the name of your lambda function but if you do, you need to tell the Lambda that you have changed the name of the entry function. It can be done inside the runtime settings of the Lambda function.
Can I Handle Errors In My Lambda Handler Function?
Yes, you can handle errors in your Lambda Handler function by using the ‘try’ – except’ statements returning the appropriate response whenever something goes wrong.
How Can I Call My AWS Lambda Function?
AWS Lambda functions are invoked by triggers. Triggers are any event that’s happening in other AWS Services, like calling an API, or Creating an object in Amazon S3 bucket, or updating an item in DynamoDB etc.
Is It Compulsory For Lambda Handler Function To Return Anything?
No, it’s not compulsory for Lambda Handler function to return values. It all depends upon the use-case.
Share your thoughts in the comments
Please Login to comment...