jQuery Timepicker Plugin
Last Updated :
13 Jul, 2023
In this article, we will learn how to add a time picker to the jQueryUI Datepicker using the jQuery Timepicker-Addon image plugin.
Note: Please download the jQuery Timepicker plugin in the working folder and include the required files in the head section of your HTML code.
<link href=”http://code.jquery.com/ui/1.11.0/themes/smoothness/jquery-ui.css” rel=”stylesheet” type=”text/css”/>
<link href=”jquery-ui-timepicker-addon.css” rel=”stylesheet” type=”text/css”/>
<script src=”http://code.jquery.com/jquery-1.11.1.min.js”></script> <script src=”http://code.jquery.com/ui/1.11.0/jquery-ui.min.js”></script> <script src=”jquery-ui-timepicker-addon.js”></script>
Example 1: The following example demonstrates the instantiation of the jQuery datetimepicker() method of the plugin.
html
<!DOCTYPE html>
< html lang = "en"
xmlns =
< head >
< meta http-equiv = "Content-Type"
content = "text/html; charset=UTF-8" />
< title >Adding a Timepicker to
jQuery UI Datepicker</ title >
< style type = "text/css" >
body {
margin: 0;
padding: 20px;
border: 0;
background-color: #e9e9e9;
border-top: solid 10px #7ba9b2;
font: Arial, sans-serif;
}
.wrapper {
width: 800px;
border: solid 1px #eeeeee;
background-color: #ffffff;
padding: 20px;
margin: 0 auto;
}
</ style >
< link rel = "stylesheet"
media = "all"
type = "text/css"
href=
smoothness/jquery-ui.css" />
< link rel = "stylesheet"
media = "all"
type = "text/css"
href =
"jquery-ui-timepicker-addon.css" />
</ head >
< body >
< div class = "wrapper" >
< h2 >Adding a Timepicker to
jQuery UI Datepicker</ h2 >
< div class = "container" >
< p >Add a simple jQuery
UI datetimepicker</ p >
< div >
< input type = "text"
name = "dateTimePicker"
id = "dateTimePicker"
value = "" />
</ div >
</ div >
</ div >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src =
"jquery-ui-timepicker-addon.js" >
</ script >
< script type = "text/javascript" >
// Initialization of datetimepicker
$(function () {
$("#dateTimePicker").datetimepicker();
});
</ script >
</ body >
</ html >
|
Output:
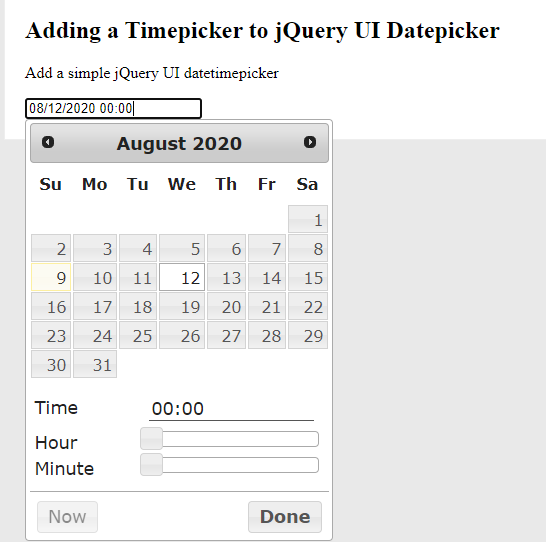
Adding a Timepicker to jQuery UI Datepicker: In the above HTML code, we can add following settings for “timeFormat”, “timezonelist” and for localization. There are many more options that can be used by the programmer depending on the need.
javascript
$( function () {
$( '#timePicker' ).datetimepicker({
timeFormat: "hh:mm tt" ,
timezoneList: [
{ value: -300, label: 'Eastern' },
{ value: -360, label: 'Central' },
{ value: -420, label: 'Mountain' },
{ value: -480, label: 'Pacific' }
]
});
$( '#timePicker' ).timepicker(
$.timepicker.regional[ 'en' ]
);
});
|
Example 2: The following example demonstrates the slider feature with step time intervals for an hour, minute, and seconds time. Please refer to the output for a better understanding. Once the slider is moved, it jumps in time intervals mentioned in the options settings for the time as shown below.
Note: Please don’t forget to include the following libraries in your HTML code for the slider feature.
<script src=”i18n/jquery-ui-timepicker-addon-i18n.min.js”></script> <script src=”jquery-ui-sliderAccess.js”></script>
html
<!DOCTYPE html>
< html lang = "en"
xmlns =
< head >
< meta http-equiv = "Content-Type"
content = "text/html; charset=UTF-8" />
< title >Adding a Timepicker to
jQuery UI Datepicker</ title >
< style type = "text/css" >
body {
margin: 0;
padding: 20px;
border: 0;
background-color: #e9e9e9;
border-top: solid 10px #7ba9b2;
font: Arial, sans-serif;
}
.wrapper {
width: 800px;
border: solid 1px #eeeeee;
background-color: #ffffff;
padding: 20px;
margin: 0 auto;
}
</ style >
< link rel = "stylesheet"
media = "all"
type = "text/css"
href =
< link rel = "stylesheet"
media = "all"
type = "text/css"
href =
"jquery-ui-timepicker-addon.css" />
</ head >
< body >
< div class = "wrapper" >
< h2 >Adding a step interval
timepicker to jQuery UI Datepicker</ h2 >
< div class = "container" >
< p >Adding slider with step
intervals for hour, minute and seconds</ p >
< div >
< input type = "text"
name = "datePicker"
id = "datePickerID" value = "" />
</ div >
</ div >
</ div >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src =
"jquery-ui-timepicker-addon.js" >
</ script >
< script type = "text/javascript"
src =
"i18n/jquery-ui-timepicker-addon-i18n.min.js" >
</ script >
< script type = "text/javascript"
src =
"jquery-ui-sliderAccess.js" >
</ script >
< script type = "text/javascript" >
$(function () {
$("#datePickerID").datetimepicker();
$("#datePickerID").timepicker({
hourGrid: 4,
minuteGrid: 10,
timeFormat: "hh:mm tt",
addSliderAccess: true,
sliderAccessArgs: { touchonly: true },
stepHour: 2,
// hour interval step
stepMinute: 10,
// minute interval step
stepSecond: 10,
// second interval step
});
});
</ script >
</ body >
</ html >
|
Output:
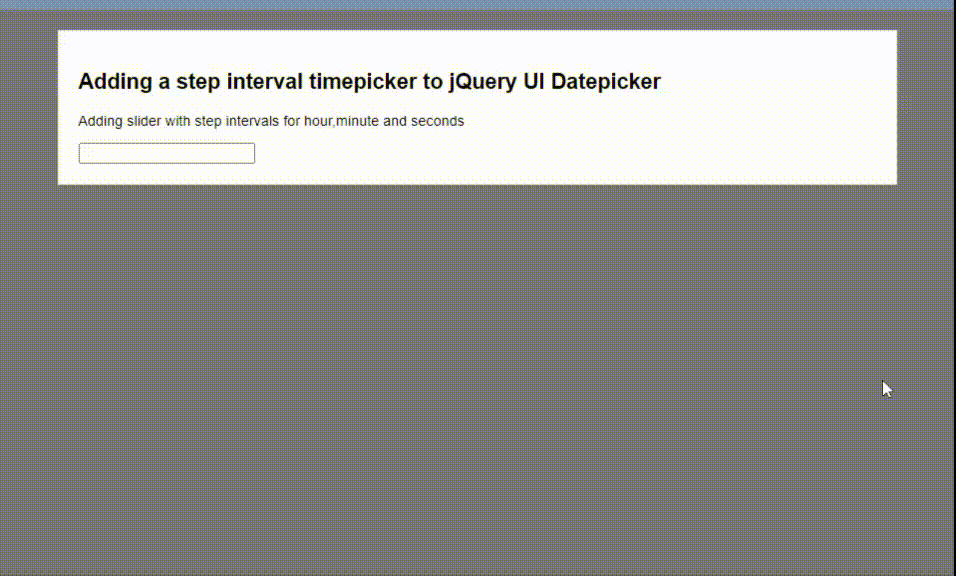
Example 3: The following example demonstrates adding a dropdown selector for the datetimepicker().
html
<!DOCTYPE html>
< html lang="en"
< head >
< meta http-equiv="Content-Type"
content="text/html; charset = UTF -8" />
< title >Adding a Timepicker to
jQuery UI Datepicker</ title >
< style type="text/css">
body {
margin: 0;
padding: 20px;
border: 0;
background-color: #e9e9e9;
border-top: solid 10px #7ba9b2;
font: Arial, sans-serif;
}
.wrapper {
width: 800px;
border: solid 1px #eeeeee;
background-color: #ffffff;
padding: 20px;
margin: 0 auto;
}
</ style >
< link
rel="stylesheet"
media="all"
type="text/css"
smoothness/jquery-ui.css"
/>
< link rel="stylesheet"
media="all"
type="text/css"
href="jquery-ui-timepicker-addon.css" />
</ head >
< body >
< div class="wrapper">
< h2 >Adding a Timepicker to
jQuery UI Datepicker</ h2 >
< div class="container">
< p >Use of dropdowns in datetime picker</ p >
< div >
< input type="text"
name="datePicker"
id="datePickerID"
value="" />
</ div >
</ div >
</ div >
< script type="text/javascript"
src=
</ script >
< script type="text/javascript"
src=
</ script >
< script type="text/javascript"
src="jquery-ui-timepicker-addon.js">
</ script >
< script type="text/javascript">
$(function () {
$("#datePickerID").datetimepicker({
controlType: "select",
oneLine: true,
timeInput: true,
timeFormat: "hh:mm tt",
});
});
</ script >
</ body >
</ html >
|
Output:
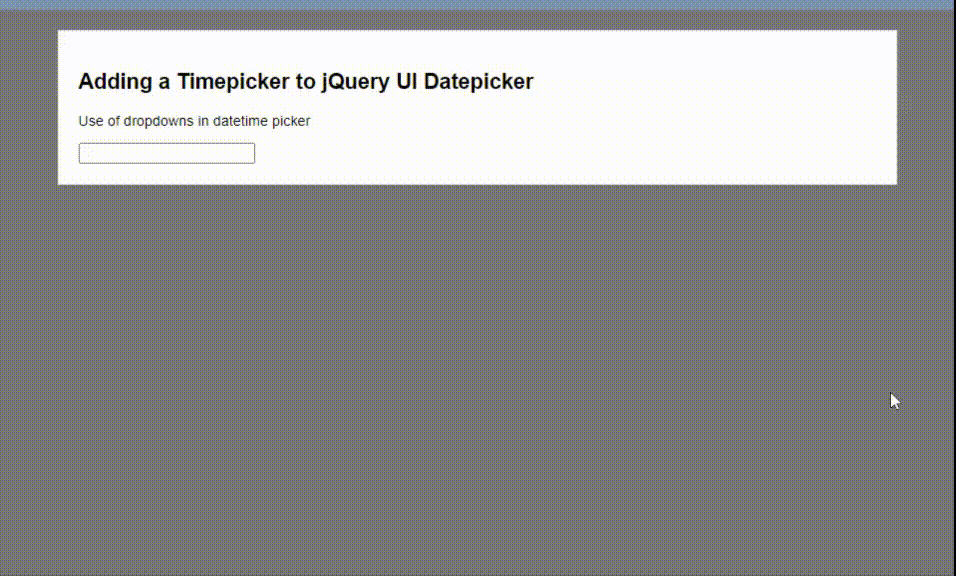
Share your thoughts in the comments
Please Login to comment...