jQuery GMaps Plugin
Last Updated :
13 Jul, 2023
jQuery provides GMaps Plugin which helps programmers to use Google maps in variety of ways. You have to download the required files in the working folder so that the programmer can include in the head section of the HTML structure page as implemented in the following programs.
jQuery GMaps Plugin download link: https://hpneo.dev/gmaps/
In the following examples, we are using a valid existing location’s latitude and longitude which can be got from entering a location address in the input control box from the below link. Please make a note of latitude and longitude of the user input address for further proceeding in the code.
https://www.latlong.net/
Example 1: The following example demonstrates the basic call of GMaps plugin based on latitude and longitude values to show the location in the map .
html
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" />
< title >jQuery GMaps Plugin</ title >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src = "gmaps.js" >
</ script >
< link rel = "stylesheet"
href =
< link rel = "stylesheet"
type = "text/css"
href = "examples.css" />
< style >
body {
text-align: center;
}
</ style >
< script type = "text/javascript" >
let map;
$(document).ready(function () {
map = new GMaps({
el: "#map",
lat: 21.164904,
lng: 81.324297,
zoomControl: true,
zoomControlOpt: {
style: "SMALL",
position: "BOTTOM_LEFT",
},
panControl: true,
streetViewControl: true,
mapTypeControl: true,
});
});
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksForGeeks
</ h1 >
< b >jQuery GMaps Plugin</ b >
< div class = "row" >
< div class = "span11" >
< div id = "map" ></ div >
</ div >
</ div >
</ body >
</ html >
|
Output :
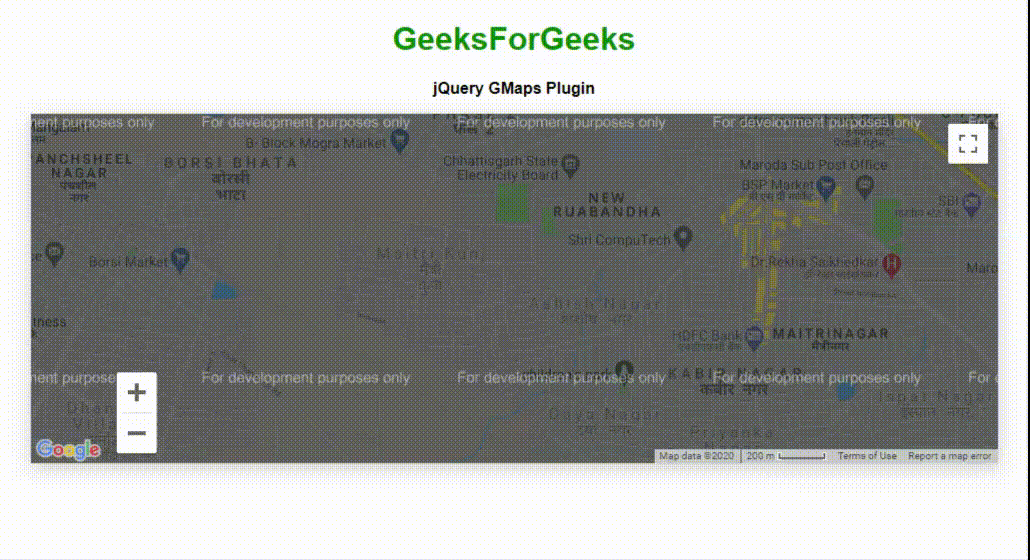
Example 2: The following program uses the GMap plugin to draw circle around the input location.
html
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" />
< title >jQuery GMaps Plugin</ title >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src = "gmaps.js" >
</ script >
< link rel = "stylesheet"
href =
< link rel = "stylesheet"
type = "text/css"
href = "examples.css" />
< style >
body {
text-align: center;
}
</ style >
< script type = "text/javascript" >
let map;
$(document).ready(function () {
map = new GMaps({
el: "#map",
lat: 17.4574683,
lng: 78.2822645,
});
let latitude = 17.4574683;
let longitude = 78.2822645;
circle = map.drawCircle({
lat: latitude,
lng: longitude,
radius: 451,
strokeColor: "#33FFAF",
strokeOpacity: 1,
strokeWeight: 4,
fillColor: "#33FFAF",
fillOpacity: 0.5,
});
for (let i in paths) {
bounds.push(paths[i]);
}
let arrayVar = [];
for (let i in bounds) {
latitudeLongitude =
new google.maps.LatLng(bounds[i][0], bounds[i][1]);
arrayVar.push(latitudeLongitude);
}
for (let i in paths) {
latitudeLongitude =
new google.maps.LatLng(paths[i][0], paths[i][1]);
arrayVar.push(latitudeLongitude);
}
map.fitLatLngBounds(arrayVar);
});
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksForGeeks
</ h1 >
< b >
Draw circle using GMaps Plugin
</ b >
< div class = "row" >
< div class = "span11" >
< div id = "map" ></ div >
</ div >
</ div >
</ body >
</ html >
|
Output :
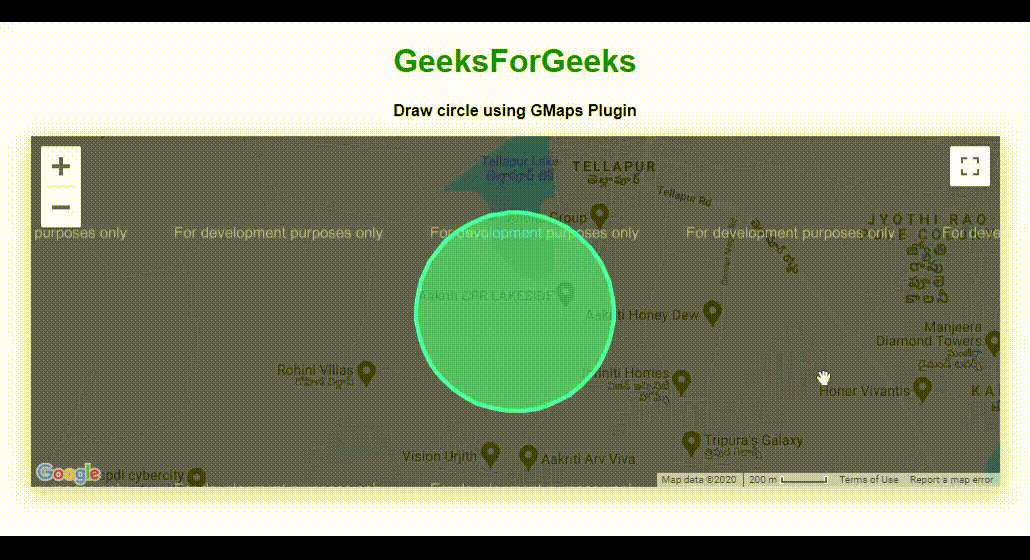
Example 3: The following program demonstrates the event handling feature of the plugin. It shows messages on click and drag events.
html
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" />
< title >jQuery GMaps Plugin Event handling</ title >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src = "gmaps.js" >
</ script >
< link rel = "stylesheet"
href =
< link rel = "stylesheet"
type = "text/css"
href = "examples.css" />
< style >
body {
text-align: center;
}
.eventClass {
width: 90%;
text-align: center;
font-weight: bold;
padding: 10px;
box-sizing: content-box;
}
</ style >
< script type = "text/javascript" >
let map;
$(document).ready(function () {
map = new GMaps({
el: "#map",
zoom: 15,
lat: 17.4574683,
lng: 78.2822645,
click: function (e) {
let info = "Click event occurred!";
$("#ClickEventDivID").text(info);
},
dragend: function (e) {
let info = "User dragged a location !";
$("#DragEventDivID").text(info);
},
});
});
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksForGeeks
</ h1 >
< b >
jQuery GMaps Plugin Event handling
</ b >
< div class = "row" >
< div class = "span11" >
< div id = "map" ></ div >
</ div >
</ div >
< br />
< br />
< div id = "ClickEventDivID"
class = "eventClass" >
</ div >
< div id = "DragEventDivID"
class = "eventClass" >
</ div >
</ body >
</ html >
|
Output :
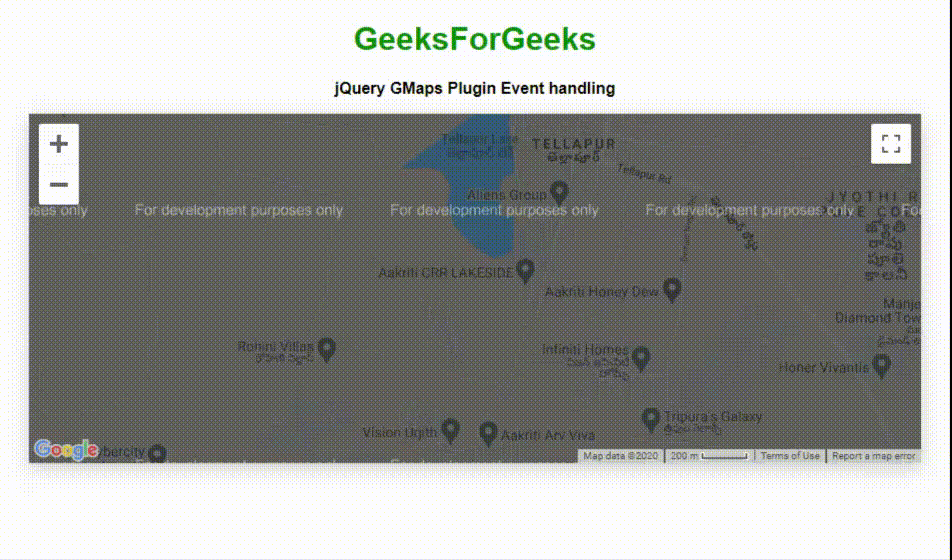
Example 4: The following example demonstrates OpenStreetMap map type of the GMap plugin.
html
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" />
< title >jQuery GMaps Plugins Map Types</ title >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src =
</ script >
< script type = "text/javascript"
src = "gmaps.js" >
</ script >
< link rel = "stylesheet"
< link rel = "stylesheet"
type = "text/css"
href = "examples.css" />
< style >
body {
text-align: center;
}
</ style >
< script type = "text/javascript" >
let map;
$(document).ready(function () {
map = new GMaps({
el: "#map",
lat: 17.47514,
lng: 78.3003,
mapTypeControlOptions: {
mapTypeIds: ["hybrid", "roadmap", "satellite",
"terrain", "osm", "cloudmade"],
},
});
map.addMapType("osm", {
getTileUrl: function (coord, zoom) {
+ zoom + "/" + coord.x + "/" + coord.y + ".png";
},
tileSize: new google.maps.Size(256, 256),
name: "OpenStreetMap",
maxZoom: 15,
});
map.setMapTypeId("osm");
});
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksForGeeks
</ h1 >
< b >
jQuery GMaps Plugin Open Street Map
</ b >
< div class = "row" >
< div class = "span11" >
< div id = "map" ></ div >
</ div >
</ div >
</ body >
</ html >
|
Output :
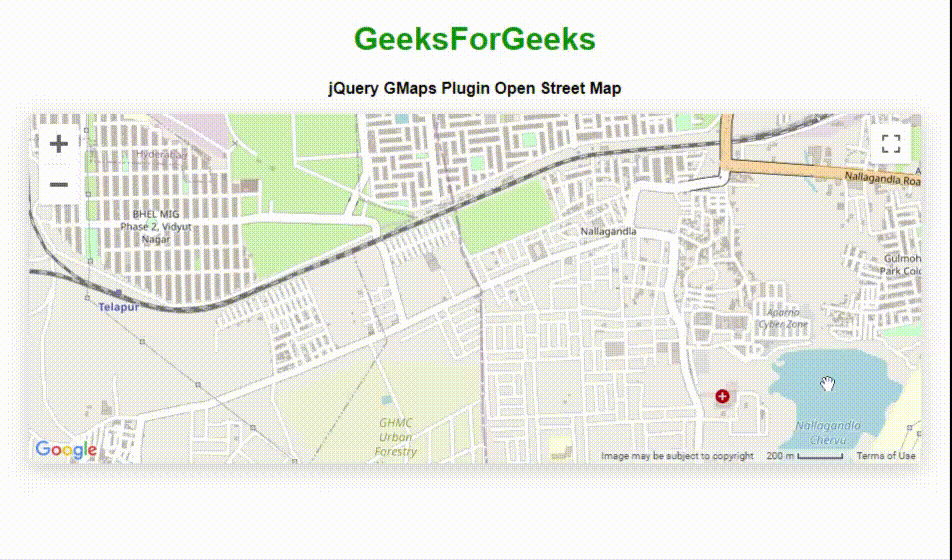
Example 5: The following example demonstrates adding layers to the location in map which is shown in the output image.
html
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" />
< title >jQuery GMaps Layers Maps</ title >
< script src =
</ script >
< script src =
</ script >
< script src = "gmaps.js" >
</ script >
< link rel = "stylesheet"
href =
< link rel = "stylesheet"
type = "text/css"
href = "examples.css" />
< style >
body {
text-align: center;
}
</ style >
< script type = "text/javascript" >
let map;
$(function () {
map = new GMaps({
el: "#map",
lat: 17.4574683,
lng: 78.2822645,
zoom: 3,
});
map.addLayer("weather", {
clickable: true,
});
map.addLayer("traffic");
});
</ script >
</ head >
< body >
< h1 style = "color: green" >
GeeksForGeeks
</ h1 >
< b >
jQuery GMaps Adding layers Feature
</ b >
< div class = "row" >
< div class = "span11" >
< div id = "map" ></ div >
</ div >
</ div >
</ body >
</ html >
|
Output :
There are many more variety of ways to make use of google maps using GMap plugin. The programmer can make use of all these depending on the application’s requirement.
Share your thoughts in the comments
Please Login to comment...