JQuery map() Method
Last Updated :
11 Jul, 2023
This map() Method in jQuery is used to translate all items in an array or object to a new array of items.
Syntax:
jQuery.map( array/object, callback )
Parameters: This method accepts two parameters which are mentioned above and described below:
- array/object: This parameter holds the Array or object to translate.
- callback: This parameter holds the function to process each item against.
Return Value: It returns the array.
The below examples illustrate the use of map() method in jQuery:
Example 1: This example uses jQuery.map() method and return the square of the array element.
html
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" >
< title >JQuery | map() method</ title >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< h3 >JQuery | map() method</ h3 >
< b >Array = [2, 5, 6, 3, 8, 9]</ b >
< br >
< br >
< button onclick = "geek()" >
Click
</ button >
< br >
< br >
< b id = "root" ></ b >
< script >
function geek() {
let el = document.getElementById('root');
let arr = [2, 5, 6, 3, 8, 9];
let newArr = jQuery.map(arr, function (val, index) {
return {
number: val,
square: val * val
};
})
el.innerHTML = JSON.stringify(newArr);
}
</ script >
</ body >
</ html >
|
Output:
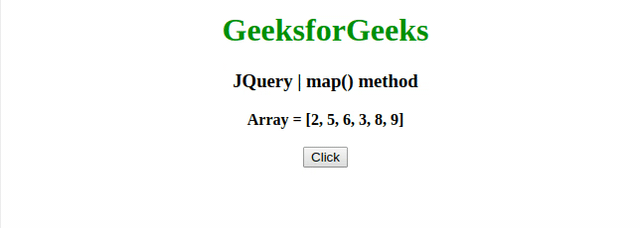
Example 2: This example use map() method to concatenate character ‘A’ with every character of the name.
html
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" >
< title >JQuery | map() method</ title >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< h3 >JQuery | map() method</ h3 >
< b >String = "Shubham"</ b >
< br >
< br >
< button onclick = "geek()" >
Click
</ button >
< br >
< br >
< b id = "root" ></ b >
< script >
function geek() {
let el = document.getElementById('root');
let name = "Shubham";
name = name.split("");
// New array of character and names
// concatenated with 'A'
let newName = jQuery.map(name, function (item) {
return item + 'A< br >';
})
el.innerHTML = newName;
}
</ script >
</ body >
</ html >
|
Output:
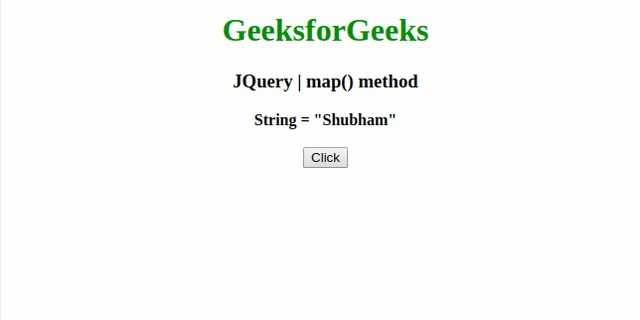
Share your thoughts in the comments
Please Login to comment...