JavaScript Program to Find Volume of a Cube
Last Updated :
15 Mar, 2024
A Cube is a 3-dimensional box-like figure represented in the 3-dimensional plane that has six-shaped faces. It has length, width and height of the same dimension.
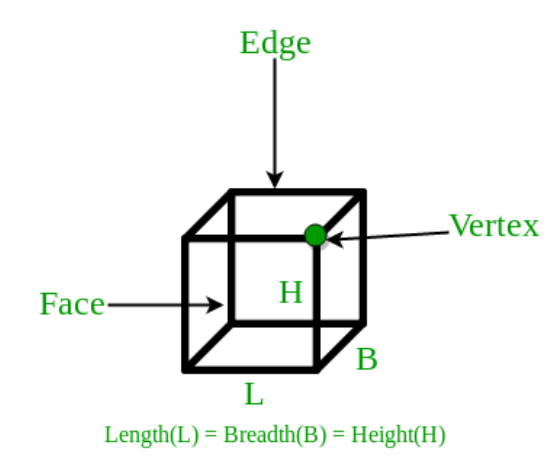
The Volume (V) of a Cube can be calculated using the given formula: Volume(V) = a * a * a
Where, a is the Edge of the Cube.
Example:
Input : 10
Output : Volume = 1000
Input : 5
Output : Volume = 125
In this Scenario, we will create a function that will hold the formula by taking edge of a cube (a) as a parameter. The function proceeds to calculate the Volume of the Cube using the formula (a * a * a). The calculated Volume is returned as the result of the function.
Syntax:
function volCube(a) {
return a * a * a;
}
Example: Below is the function to find Volume of Cube using the general formulae:
Javascript
function volCube(a) {
return a * a * a;
}
let a = 5;
console.log('Volume = '+volCube(a));
Output:
Volume = 125
Using Math.pow() function
The Math. pow() method is used to power a number i.e., the value of the number raised to some exponent. In this case, we need to pass a (edge) as base and exponent is 3.
Syntax:
Math.pow(base, exponent)
Parameters:
- base: It is the base number that is to be raised.
- exponent: It is the value used to raise the base.
Example: Below is the function to find Volume of Cube using Math.pow() function.
Javascript
let a = 5;
console.log('Volume = '+Math.pow(a,3));
Output:
Volume = 125
Exponentiation operator is represented by “**” which is used to find the power of the first operator raised to the second operator. We need to pass edge (a) as the first operator and 3 as the second operator.
Syntax:
a ** 3
Example: Below is the function to find Volume of Cube using the Exponentiation operator.
Javascript
let a = 5;
console.log('Volume = '+a ** 3);
Output:
Volume = 125
Using ES6 arrow function
ES6 Arrow functions enable us to write functions with simpler and shorter syntax. We can utilize this function that will return the Volume of a Cube. First we will define a function and pass a (edge) as an argument. we will write the mathematical expression that will return the volume of a cube. Finally, we will call the function.
Syntax:
volCube = (a) => {
return(a * a * a)
}
Example: Below is the function to find Volume of Cube using the arrow function.
Javascript
volCube = (a) => {
return(a * a * a)
}
console.log('Volume = '+volCube(5));
Output:
Volume = 125
Time complexity: O(1), as the execution time is constant and does not grow with the size of the input.
Auxiliary Space complexity: O(1) as it uses a constant amount of memory regardless of the input size.
Share your thoughts in the comments
Please Login to comment...