JavaScript Program to Find the Area of Sphere
Last Updated :
28 Dec, 2023
A sphere is a three-dimensional geometric shape that is perfectly round and symmetrical in all directions. It is defined as the set of all points in space that are equidistant (at an equal distance) from a central point. This central point is called the center of the sphere. In this article, we are going to learn how to find the area of the Sphere.
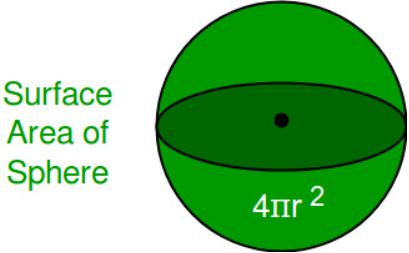
The area (A) of a sphere can be calculated using the given formula:
Area(A) = 4 * π * r2
where,
- π represents the mathematical constant Pi (approximately 3.14159),
- r represents the Radius of the Sphere
Example:
Input : radius = 5
Output: Area of the sphere: 314.16
Explanation: Using the formula we get 4*3.1416*5*5 = 314.16
Input : radius = -10
Output: Error: Invalid radius. Please enter a valid positive number.
Explanation: As the radius is negative which is not possible so it will show an error.
Approach:
- A function named calculateSphereArea is defined, which takes the radius of a sphere as an argument.
- The function begins by checking if the provided radius is a valid number and greater than or equal to 0.
- If the radius is not a valid number or is negative, the function returns an error message indicating that the radius is invalid.
- If the radius is valid, the function proceeds to calculate the area of the sphere using the formula 4Ï€r2.
- The calculated area is returned as the result of the function.
Example: Below is the function to find area of Sphere:
Javascript
function calculateSphereArea(radius) {
if (isNaN(radius) || radius < 0) {
return "Error: Invalid radius. Please enter a valid positive number." ;
}
let area = 4 * Math.PI * Math.pow(radius, 2);
return area;
}
let radius = 5;
let sphereArea = calculateSphereArea(radius);
if ( typeof sphereArea === 'number' ) {
console.log( "Area of the sphere: " + sphereArea.toFixed(2));
} else {
console.log(sphereArea);
}
|
Output
Area of the sphere: 314.16
Time complexity: O(1), as the execution time is constant and does not grow with the size of the input.
Auxilitary Space complexity: O(1) as it uses a constant amount of memory regardless of the input size.
Share your thoughts in the comments
Please Login to comment...