Javascript Program to check idempotent matrix
Last Updated :
20 Dec, 2022
Given an N * N matrix and the task is to check matrix is an idempotent matrix or not.
Idempotent matrix: A matrix is said to be an idempotent matrix if the matrix multiplied by itself returns the same matrix. The matrix M is said to be an idempotent matrix if and only if M * M = M. In an idempotent matrix M is a square matrix.
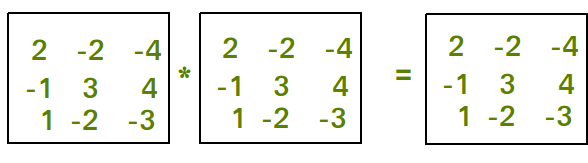
Examples:
Input : mat[][] = {{3, -6},
{1, -2}};
Output : Idempotent Matrix
Input : mat[N][N] = {{2, -2, -4},
{-1, 3, 4},
{1, -2, -3}}
Output : Idempotent Matrix.
Javascript
<script>
var N = 3;
function multiply(mat, res)
{
for ( var i = 0; i < N; i++)
{
for ( var j = 0; j < N; j++)
{
res[i][j] = 0;
for ( var k = 0; k < N; k++)
res[i][j] += mat[i][k] * mat[k][j];
}
}
return res;
}
function checkIdempotent(mat)
{
var res = Array.from(Array(N), ()=>Array(N).fill(0));
res = multiply(mat, res);
for ( var i = 0; i < N; i++)
{
for ( var j = 0; j < N; j++)
{
if (mat[i][j] != res[i][j])
return false ;
}
}
return true ;
}
var mat = [[2, -2, -4],
[-1, 3, 4],
[1, -2, -3]];
if (checkIdempotent(mat))
document.write( "Idempotent Matrix" );
else
document.write( "Not Idempotent Matrix." );
</script>
|
Output:
Idempotent Matrix
Time Complexity: O(N3)
Auxiliary Space: O(N2)
Please refer complete article on Program to check idempotent matrix for more details!
Share your thoughts in the comments
Please Login to comment...