JavaScript Methods to Increment/Decrement Alphabetical Letters
Last Updated :
20 Jun, 2023
The task is to increment/decrement alphabetic letters using ASCII values with the help of JavaScript.
Approach:
- Use charCodeAt() method to return the Unicode of the character at the specified index in a string.
- Increment/Decrement the ASCII value depending on the requirement.
- Use fromCharCode() method to convert Unicode values into characters.
Example 1: In this example, the character ‘a’ is incremented by clicking on the button.
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >
Click on the button to
increment the letter
</ title >
</ head >
< body >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< p >
Click on the button to
increment the letter.
</ p >
< button onclick = "GFG_Fun()" >
click here
</ button >
< p id = "GFG" ></ p >
< script >
var down = document.getElementById('GFG');
down.innerHTML = 'a';
function nextCharacter(c) {
return String.fromCharCode(c.charCodeAt(0) + 1);
}
function GFG_Fun() {
let c = down.innerHTML;
down.innerHTML = nextCharacter(c);
}
</ script >
</ body >
</ html >
|
Output:
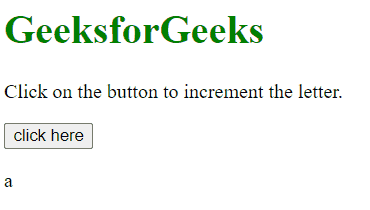
JavaScript methods to increment/decrement alphabetical letters
Example 2: In this example, the character ‘z’ is decremented by clicking on the button.
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >
Click on the button to
decrement the letter
</ title >
</ head >
< body >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< p >
Click on the button to
decrement the letter.
</ p >
< button onclick = "GFG_Fun()" >
click here
</ button >
< p id = "GFG" ></ p >
< script >
var down = document.getElementById('GFG');
down.innerHTML = 'z';
function nextCharacter(c) {
return String.fromCharCode(c.charCodeAt(0) - 1);
}
function GFG_Fun() {
let c = down.innerHTML;
down.innerHTML = nextCharacter(c);
}
</ script >
</ body >
</ html >
|
Output:
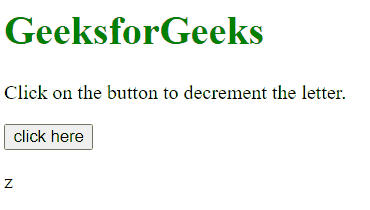
JavaScript methods to increment/decrement alphabetical letters
Share your thoughts in the comments
Please Login to comment...