Java String Literals as Constants or Singletons
Last Updated :
05 Feb, 2023
String is a sequence of characters, which is widely used in Java Programming. In the Java programming language, strings are objects. In Java, the virtual machine may only create a single String instance in memory, if we use the same string in other string variable declarations. Let us consider illustrations for the sake of understanding which is as follows:
Illustration 1:
String string1 = "GeeksForGeeks";
String string2 = "GeeksForGeeks";
In the above example, the java virtual machine will create only one instance of “GeeksForGeeks” in memory. There are two different variables, initialized to “GeeksForGeeks” string will point to the same String instance in memory. The figure below depicts example more accurately:-
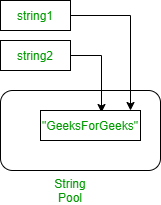
The string literal thus becomes a de facto constant or singleton. More precisely in java, objects representing Java String literals are obtained from a constant String pool which is internally kept by a java virtual machine. This means, that even classes from different projects compiled separately, but which are used in the same application may share constant String objects. The sharing happens at runtime and hence it is not a compile-time feature.
Illustration 2: If we want those two string variables point to separate String objects then we use the new operator as follows:
String string1 = new String("GeeksForGeeks");
String string2 = new String("GeeksForGeeks");
The above code will create two different memory objects in memory to represent them even if the value of two strings is the same. The image below depicts the use of new operator:
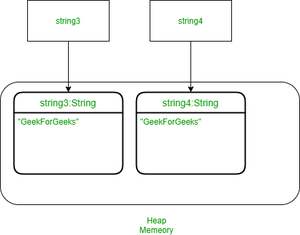
Note: From the image above it is clearly depicted that two different memory blocks are created for two different string which contains same value string.
Example 1:
Java
public class GFG {
public static void main(String[] args)
{
String string1 = "GeeksForGeeks" ;
String string2 = "GeeksForGeeks" ;
String string3 = new String( "GeeksForGeeks" );
String string4 = new String( "GeeksForGeeks" );
if (string1 == string2) {
System.out.println( "True" );
}
else {
System.out.println( "False" );
}
if (string1 == string3) {
System.out.println( "True" );
}
else {
System.out.println( "False" );
}
if (string4 == string3) {
System.out.println( "True" );
}
else {
System.out.println( "False" );
}
}
}
|
In the above program, a total of 3 objects will be created. Now, if we want to compare 2 string contents, we can use the equals method, and ‘==’ operator can be used to compare whether two string references are the same or not.
Example 2:
Java
public class GFG {
public static void main(String[] args)
{
String string1 = "GeeksForGeeks" ;
String string3 = new String( "GeeksForGeeks" );
System.out.println(string1.equals(string3));
System.out.println(string1 == string3);
}
}
|
What is constants in Java ?
- An element of program whose value can not be changed at the time of execution of program is called constant.
- It is also called literals.
- It may be int,float and character data type.
Rules for constructing integer constant:
- It must have atleast one digit.
- It must not have a decimal point.
- It may be positive or negative.
- No comma or blank space are allowed in integer constant.
Rules for constructing floating point constant:
- It must have atleast one digit.
- It must have a decimal point.
- It may be positive or negative.
- No comma or blank space are allowed in floating point constant.
Rules for constructing character constant :
- It is a single alphabet,digit or special symbol.
- The length of character constant is 1 character.
- Character constant is enclosed within single quotes (Example char c=’A’;).
Share your thoughts in the comments
Please Login to comment...