How to Implement a Distributed Caching System Using Java Networking?
Last Updated :
26 Feb, 2024
In Java, a distributed caching system is used to store frequently accessed data in the memory across multiple servers, improving the performance and scalability of the applications. We can implement the simple distributed caching system using Java networking, and it can implement multiple cache nodes communicating with each other to store and retrieve cached data.
Program to Implement a Distributed Caching System Using Java Networking
We can implement the simple distributed caching system using Java networking and it can implement the cache server and client server then client the server requests to the server then the server responds to the client.
Step-by-Step Implementation for Cache Server:
- Create the class named the CacheServer and write the main method into it.
- Create the server socket using ServerScoket and assign the port number 5000.
- Create the try block mechanism to handle the errors and print the statement server starting.
- Server creation is complete then the server accepts the client’s request and responds to the client.
- In this server, we can implement the simple logic of the sum of the number if invalid inputs return an invalid message.
Java
import java.net.*;
import java.io.*;
public class CacheServer
{
public static void main(String[] args)
{
try (ServerSocket serverSocket = new ServerSocket( 5000 ))
{
System.out.println( "Cache server running..." );
while ( true ) {
Socket socket = serverSocket.accept();
new CacheServerThread(socket).start();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
class CacheServerThread extends Thread
{
private Socket socket;
public CacheServerThread(Socket socket)
{
this .socket = socket;
}
public void run() {
try (
BufferedReader in = new BufferedReader( new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true )
) {
String inputLine;
while ((inputLine = in.readLine()) != null ) {
String[] numbers = inputLine.split( "," );
if (numbers.length == 2 ) {
int num1 = Integer.parseInt(numbers[ 0 ]);
int num2 = Integer.parseInt(numbers[ 1 ]);
int sum = num1 + num2;
out.println(sum);
} else
{
out.println( "Invalid input. Please provide two numbers separated by comma." );
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
|
Output:
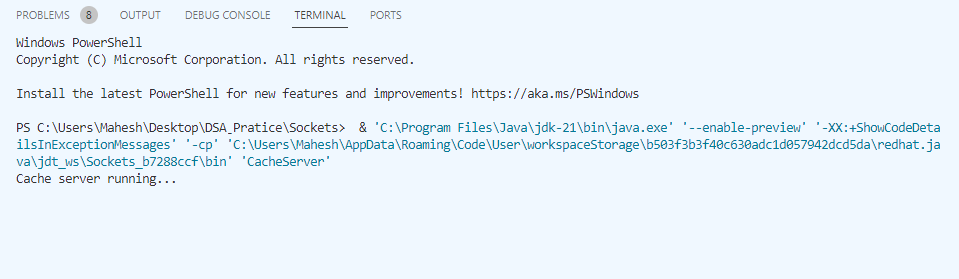
Explanation of the program:
The above program is the example of the cache server we can implement using the ServerSocket. It can implement the simple logic of the sum of two number when the client sends the request then cache server responds to the client return the results.
- ServerSocket: ServerSocket is the pre-defined class of the java networking sockets and it can be used to listens the incoming requests of the client’s connections on the specified port.
- Socket: It is a pre-defined class of the socket, and it can be establishing the client endpoint and connects to the server.
Client Program:
In this program, we can develop the simple client that can connect the server port 5000 to the cache server then send the request to the cache with two inputs then server process the request and response with results.
Java
import java.net.*;
import java.io.*;
public class CacheClient {
public static void main(String[] args)
{
try (
Socket socket = new Socket( "localhost" , 5000 );
BufferedReader userInput = new BufferedReader( new InputStreamReader(System.in));
BufferedReader in = new BufferedReader( new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true )
) {
System.out.println( "Enter two numbers separated by comma:" );
String input = userInput.readLine();
out.println(input);
String response = in.readLine();
System.out.println( "Sum received from server: " + response);
} catch (IOException e) {
e.printStackTrace();
}
}
}
|
Output:
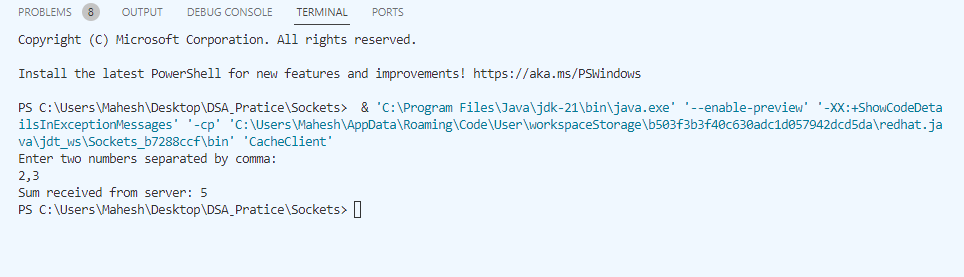
Explanation of the Program:
- The above program is the example of the client server in this program send the request to the server then server response to the client.
- In this example, client give the two inputs then server process the input return the process results to the client.
Share your thoughts in the comments
Please Login to comment...