Identifying Test files and Functions using Pytest
Last Updated :
08 Dec, 2023
The Python framework used for API testing is called Pytest. For executing and running the tests, it is crucial to identify the test files and functions. Pytest, by default, executes all the test files under a certain package which are of the format test_*.py or *_test.py, in case there is no test file mentioned. Similarly, for functions, all the functions of format test* are executed in case no function is specified.
Identifying Test Files using Pytest
We can identify test files by using Pytest. Below is the syntax of this.
Syntax: pytest -k substring_match -v
Here,
- substring_match: It is the substring to be matched while executing the tests in Pytest.
File Structure
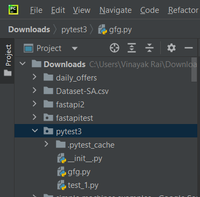
Example 1
In this example, we have created a package fastapi3, in which we have created two Python files, test_1.py and gfg.py. When we will run the tests, we will see only the tests of the file starting with test_, i.e., test_1.py will be executed.
Python3
import math
def test_check_floor():
num = 6
assert num = = math.floor( 6.34532 )
def test_check_equal():
assert 50 = = 49
|
gfg.py: Here, we are creating 2 test cases.
Python3
import math
def test_check_difference():
assert 99 - 43 = = 57
def test_check_square_root():
val = 8
assert val = = math.sqrt( 81 )
|
Output
Now, we will run the following command with substring_match as check as follows:
pytest -k check -v
Video Output
Identifying Test Functions using Pytest
We can identify test functions using Pytest. Below are the syntax for this.
Syntax: pytest main.py -k substring_match -v
Here,
- substring_match: It is the substring to be matched while executing the tests in Pytest.
Example 2
In this example, we have created a file gfg2.py where we have declared four functions, test_check_floor, check_equal, test_check_difference and check_square_root. When we will run the tests having check, then only the functions starting with test, i.e., test_check_floor and test_check_difference will be executed.
Python3
import math
def test_check_floor():
num = 6
assert num = = math.floor( 6.34532 )
def check_equal():
assert 50 = = 49
def test_check_difference():
assert 99 - 43 = = 57
def check_square_root():
val = 8
assert val = = math.sqrt( 81 )
|
Output
Now, we will run the following command with substring_match as check as follows:
pytest -k check -v
Video Output
Share your thoughts in the comments
Please Login to comment...