How to Use SQLite Viewer Flask
Last Updated :
05 Jun, 2023
SQLite is a popular lightweight relational database management system that is used in a variety of applications. Flask is a popular web framework for building web applications in Python. SQLite Viewer Flask is a tool that allows you to view the contents of an SQLite database within a Flask application.
Flask is a web framework for building web applications in the Python programming language. It is a lightweight framework that provides the necessary tools and libraries to build web applications quickly and easily. In this article, we will learn how to use SQLite Viewer in Flask.
Step 1: Install Required Libraries
The first step is to install the required libraries. You will need to install Flask and SQLite3. You can install them using pip.
pip install Flask
pip install sqlite3
Step 2: Project Structure
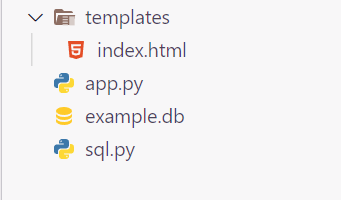
Project Structure
Step 3: Create an SQLite Database and insert values into the database
The next step is to create an SQLite database. You can use any SQLite management tool to create an SQLite database. For this example, we will create a simple database with a single table called “users” with columns for “id”, “name”, and “email”.
sql.py
Python3
import sqlite3
conn = sqlite3.connect( 'example.db' )
c = conn.cursor()
c.execute(
)
c.execute(
)
conn.commit()
conn.close()
|
Output:
Step 4: Create Flask Application
First, we set up the project using the following commands:
touch app.py
This is a Python code for a simple Flask web application that retrieves data from a SQLite database table called ‘users’ and renders it on an HTML template called ‘index.html’. The Flask app is created using the Flask class, and a route is defined for the default URL. When the route is accessed, the app connects to the database, retrieves all the data from the ‘users’ table, pass it as a variable to the ‘index.html’ template, and renders the template with the data. Finally, the app is run in debug mode using the run() method.
app.py
Python3
from flask import Flask, render_template
import sqlite3
app = Flask(__name__)
@app .route( '/' )
def home():
conn = sqlite3.connect( 'example.db' )
c = conn.cursor()
c.execute( 'SELECT * FROM users' )
users = c.fetchall()
conn.close()
return render_template( 'index.html' , users = users)
if __name__ = = '__main__' :
app.run(debug = True )
|
Step 5: Create an HTML Template
Finally, we need to create an HTML template that will display the contents of our SQLite database.
mkdir templates
cd templates
touch index.html
index.html
HTML
<!DOCTYPE html>
< html >
< head >
< title >SQLite Viewer Flask</ title >
</ head >
< body >
< h1 >Users</ h1 >
< table >
< tr >
< th >ID</ th >
< th >Name</ th >
< th >Email</ th >
</ tr >
{% for user in users %}
< tr >
< td >{{ user[0] }}</ td >
< td >{{ user[1] }}</ td >
< td >{{ user[2] }}</ td >
</ tr >
{% endfor %}
</ table >
</ body >
</ html >
|
Output
.png)
Output
Output GIF
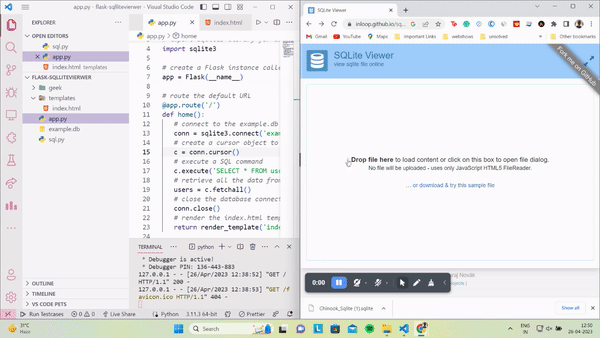
SQLite Viewer GIF
Share your thoughts in the comments
Please Login to comment...