How to use Selector Inheritance and Multiple Inheritance ?
Last Updated :
22 Sep, 2023
In this article, you will see how to use Selector Inheritance and Multiple Inheritance using SASS. In SASS(Syntactically Awesome Style Sheets), a CSS preprocessor, we can create selector inheritance and multiple inheritances through the use of mixins and extends. The problem with using inheritance with SASS is that here you have to use other
Selector Inheritance (Using SASS)
Selector inheritance in CSS describes how styles cascade from parent elements to child elements. Unless overridden by particular styles applied explicitly to the child components, a style applied to a parent element may be inherited by its descendant elements. This enables styles to spread throughout the DOM tree. SASS allows you to inherit attributes from other selectors, it also helps you write less code and/or combine fewer elements. To implement the selector inheritance using SASS you have to carry on the styles of one selector into some other selector’s set of CSS rules by using the @extend rule like in the syntax below.
Syntax:
.parent {
color: blue; /* Style applied to the parent element */
}
.child {
@extend .parent;
/* This is the child element which is a <p> */
/* The color property will be inherited from the parent div */
}
Multiple Inheritance (Using SASS)
Multiple inheritances in CSS refer to the capacity to apply styles to an element from various sources (classes or selectors). Although multiple inheritances are not natively supported by CSS, you can nevertheless get a comparable result by mixing classes or using multiple selectors. In CSS, using several classes on an element or applying styles to elements using different selectors can produce multiple inheritance-like effects.
With Sass, you can quickly reuse and mix styles from several selectors and accomplish multiple inheritances. To implement the multiple inheritance using SASS you have to carry on the styles of several selectors into another selector’s set of CSS rules by using the @extend rule multiple times inside the selector style rules extending the different selectors like in the syntax below.
Syntax:
.class1 {
/* Styles for class1 */
}
.class2 {
/* Styles for class2 */
}
.class3 {
@extend .class1;
@extend .class1;
/* Both the styles of Class 1 and Class 2 are
inherited and will be used in class 3 */
/* Styles for class3 */
}
Example 1: The code example demonstrates how we can implement the selector inheritance in SASS using the above-given syntax. Here the first <p> element inherits from the parent div and it stays different from the other <p> element using the @extend at-rule.
index.html:
HTML
<!DOCTYPE html>
< html >
< head >
< link rel = "stylesheet" href = "style.css" >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >
How to use Selector inheritance
and multiple inheritance?
</ h3 >
< br >
< div class = "parent" >
< p >
This is some text inside a paragraph.
< br >This paragraph inherits color
from the Parent Div
</ p >
< span >This is some text inside a span.</ span >
</ div >
< br >
< p >
This is the paragraph outside
the div container.
</ p >
</ body >
</ html >
|
styles.sass: This sass file contains the main stylesheet and is compiled into .css
CSS
*
margin : 1 rem
h 1
color : green
.parent
color : green
p
color : blue
.parent p
@extend .parent
font-weight : bold
|
The above sass code is compiled into CSS using SASS preprocessor in the command line like this:
sass styles.sass style.css
style.css: The compiled CSS code:
CSS
* {
margin : 1 rem;
}
h 1 {
color : green ;
}
.parent,
.parent p {
color : green ;
}
p {
color : blue ;
}
.parent p {
font-weight : bold ;
}
|
Output:
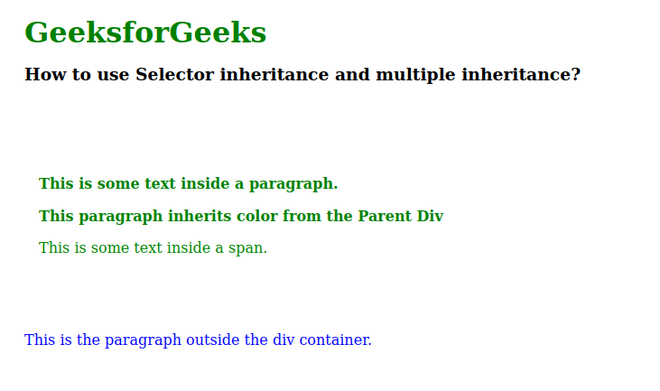
Example 2: The code example demonstrates the implementation of Multiple Inheritance using the above approach and syntax. Here the first container takes one .class3 class which inherits both the .class1 and .class2:
HTML
<!DOCTYPE html>
< html >
< head >
< link rel = "stylesheet" href = "style.css" >
</ head >
< body >
< h1 style = "color: green; margin-left: 0.5rem;" >
GeeksforGeeks
</ h1 >
< h3 style = "margin-left: 0.5rem;" >
How to use Selector inheritance
and multiple inheritance?
</ h3 >
< div class = "box" >
< div class = "class3" >
This is the container inheriting
all the classes together
</ div >
< img src =
alt = "" class = "class1 class2" >
</ div >
</ body >
</ html >
|
styles.sass: This sass file contains the main stylesheet and is compiled into .css
CSS
.box
display : flex
.class 1
height : 200px
margin : 1 rem
padding : 1 rem
.class 2
width : 200px
padding : 0.5 rem
color : green
.class 3
@extend .class 1
@extend .class 2
background-color : #e7e7e7
|
The above sass code is compiled into CSS using SASS preprocessor in the command line like this:
sass styles.sass style.css
style.css: The compiled CSS code:
CSS
.box {
display : flex;
}
.class 1 ,
.class 3 {
height : 200px ;
margin : 1 rem;
padding : 1 rem;
}
.class 2 ,
.class 3 {
width : 200px ;
padding : 0.5 rem;
color : green ;
}
.class 3 {
background-color : #e7e7e7 ;
}
|
Output:
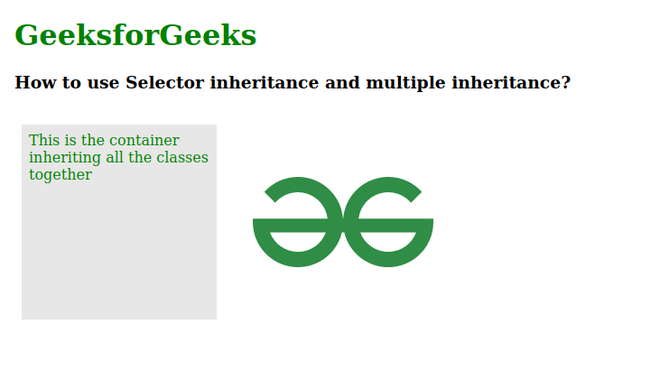
Share your thoughts in the comments
Please Login to comment...