PostgreSQL is a general–purpose object–relational database management system and is open–source. It ensures data integrity features like constraints, transactions and foreign key support. In this article, We will understand How to update table rows in PostgreSQL using subquery using various methods, examples and so on.
Introduction to Update Statement in SQL
The UPDATE statement is used to modify the records of the table. With the help of the UPDATE Statement, we can easily update the already existing data in the table.
Basic Syntax for the UPDATE statement:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
[WHERE condition];
Explanation:
- table_name is the name of table from which records will updated.
- column1, column2, etc are the columns within the table that will updated.
- value1, value2, etc are the new values to be assigned to the respective columns.
- condition is the condition that we used to update table
Setting Up Environment
Let’s create table and adding some records into table. The below query creates two tables and inserts records in them:
CREATE TABLE test1
(
id INT,
name VARCHAR(20),
age INT
);
INSERT INTO test1 VALUES(1, 'Alex', 20);
INSERT INTO test1 VALUES(4, 'Jane', 34);
INSERT INTO test1 VALUES(9, 'Tyler', 11);
CREATE TABLE test2
(
id INT,
name VARCHAR(20),
age INT
);
INSERT INTO test2 VALUES(1, 'Austin', 25);
INSERT INTO test2 VALUES(5, 'Jesse', 34);
INSERT INTO test2 VALUES(9, 'Tyler', 23);
INSERT INTO test2 VALUES(3, 'Smith', 11);
Our table test1 after some data inserted into it look like:
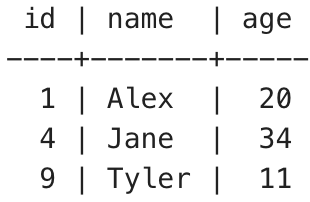
Output
Our table test2 after some data inserted into it look like:
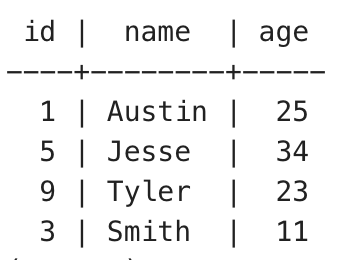
Output
Update Rows Using Subquery
We will use a UPDATE statement with a subquery to update tables based on some logic. The subquery allows us to interact with other tables during the update statement.
Example 1: Updating Based on Subquery Results
The following query updates the test1 table records which are present in test2 table with the values of the test2 table:
UPDATE test1
SET
name=t.name,
age=t.age
FROM (
SELECT * FROM test2
) t
WHERE test1.id=t.id;
Output:
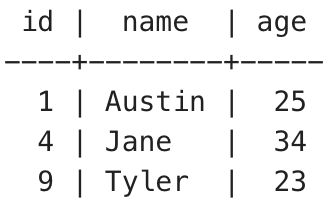
Output
Explanation: As we can see the records with ids 1 and 9 were updated in the table.
Example 2: Updating with JOIN Subquery Result
We will update the values of test1 using the value obtained by performing a join between test1 and test2 in the subquery. We take the least of the two ages in both the tables and add 2 to it. It defines the new age for the person.
UPDATE test1
SET
name=t.name,
age=t.age
FROM (
SELECT test2.id, test2.name, LEAST(test1.age, test2.age)+2 AS age FROM test2
JOIN test1
ON test1.id=test2.id
) t
WHERE test1.id=t.id;
Output:
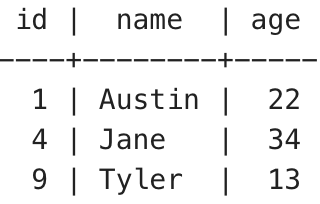
Output
Explanation: As we can see the records for 1 and 9 are updated.
Example 3: Updating with Aggregated Subquery Result
The following query updates the age of all the employees in test1 table to be the sum of all the ages in test2 value.
UPDATE test1
SET
age=t.age
FROM (
SELECT SUM(age) as age FROM test2
);
Output:
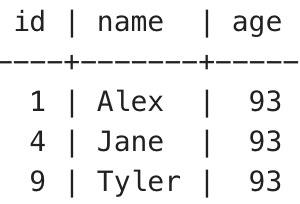
Output
Explanation: As we can see the age for all records has been updated to 93.
Technical Example
Let’s create some tables and insert some record into it for queries.
-- create department table
CREATE TABLE department (
id INT PRIMARY KEY NOT NULL,
name VARCHAR(100) NOT NULL
);
-- insert data
INSERT INTO department VALUES
(1, 'Engineering'),
(2, 'Sales'),
(3, 'Marketing');
Output:
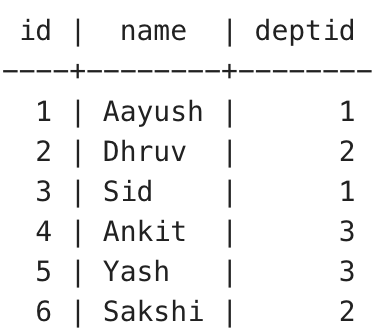
Output
The following query creates a employee table and inserts some records in it.
-- create employee table
CREATE TABLE employee (
id INT PRIMARY KEY NOT NULL,
name VARCHAR(100) NOT NULL,
deptId INT NOT NULL
);
-- insert data
INSERT INTO employee VALUES
(1, 'Aayush', 1),
(2, 'Dhruv', 2),
(3, 'Sid', 1),
(4, 'Ankit', 3),
(5, 'Yash', 3),
(6, 'Sakshi', 2);
Output:
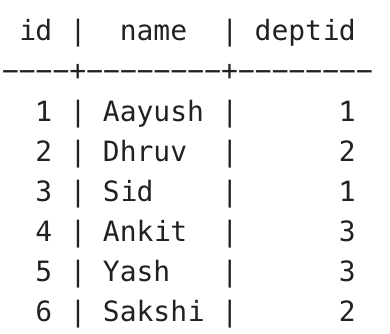
Output
The following query creates a salaries table and inserts some records in it.
-- create salary table
CREATE TABLE salary
(
id INT PRIMARY KEY NOT NULL ,
empId INT NOT NULL,
amount NUMERIC(10, 2) NOT NULL
);
-- insert data
INSERT INTO salary VALUES
(1, 1, 50000.00),
(2, 3, 55000.00),
(3, 2, 60000.00),
(4, 4, 52000.00),
(5, 6, 48000.00),
(6, 5, 45000.00);
Output:
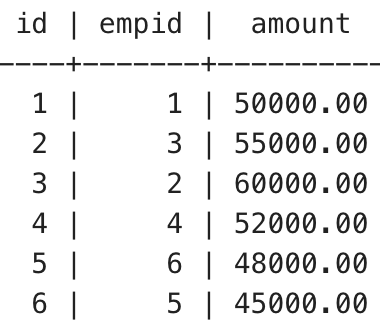
Output
Explanation: Our table has been created above.
We will update the salary of all the employees of Engineering department by 10%. We fetch the employee id for all the employees of Engineering department using a subquery and use that result in the UPDATE statement to update the salary.
UPDATE salary
SET amount = amount * 1.1
WHERE empId IN (
SELECT id
FROM employee
WHERE deptId = (
SELECT id
FROM department
WHERE name = 'Engineering'
)
);
Output:
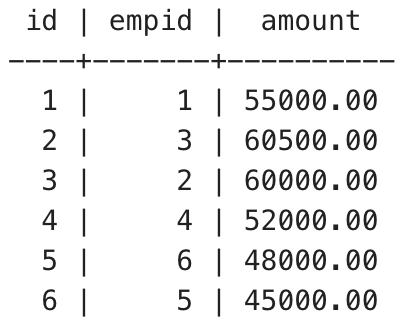
Output
Explanation: As we can see the salary of employee 1 whose name Aayush is increased from 50000 to 55000 and that of employee 3 whose name is Sid is increased from 55000 to 60500.
Let’s update the salary of each employee to average salary of their department.
UPDATE salary s
SET amount=t.avg_sal
FROM (
SELECT e.id, t.avg_sal FROM (
SELECT d.id, AVG(s.amount) AS avg_sal
FROM employee e, department d, salary s
WHERE e.deptId=d.id AND e.id=s.empId
GROUP BY d.id
) t, employee e
WHERE e.deptId=t.id
) t
WHERE s.empId=t.id;
Output:
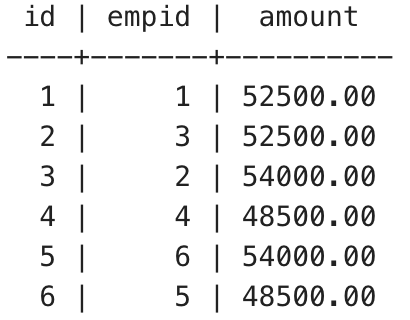
Output
Explanation: We can see the salary of each employee is updated in the salary table. We have write a two subqueries in the update statement and in the inner subquery we calculate the average salary for each department. In the outer subquery we select the average department salary for each employee.
Conclusion
In this article we covered how we can update table records in PostgreSQL using subquery. We understood how powerful using subquery alongside update statement is and can allow us to modify data in complex fashion.
Share your thoughts in the comments
Please Login to comment...