How to Style HTML Tables using Bootstrap ?
Last Updated :
10 Apr, 2024
In Bootstrap, the styling of tables can be done to enhance their appearance and layout, using classes like table, table-hover, table-bordered, and more. These classes provide options for responsive design, color schemes, borders, and hover effects, making tables visually appealing and user-friendly.
The below approaches can be used to style HTML tables using Bootstrap:
Using Table Utility Classes
In this approach, we are styling the HTML table by applying Bootstrap classes such as “table”, “table-hover”, “table-bordered”, and “table-dark” to create a responsive and visually appealing table layout.
Example 1: The below example uses Table Utility Classes to Style HTML Tables Using Bootstrap.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<link rel="stylesheet" href=
"https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<h1 style="color: green;">
Styling HTML Table using Bootstrap
</h1>
<h4>Example 1</h4>
<div class="card">
<div class="card-header bg-primary text-white">
<h4 class="mb-0">User Information</h4>
</div>
<div class="card-body">
<table class="table table-hover table-bordered">
<thead class="thead-dark">
<tr>
<th scope="col">#</th>
<th scope="col">Name</th>
<th scope="col">Email</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>GFG</td>
<td>gfg1@gfg.com</td>
</tr>
<tr>
<th scope="row">2</th>
<td>GFG2</td>
<td>gfg2@gmail.com</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
<script src=
"https://code.jquery.com/jquery-3.5.1.slim.min.js">
</script>
<script src=
"https://cdn.jsdelivr.net/npm/@popperjs/core@2.5.3/dist/umd/popper.min.js">
</script>
<script src=
"https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js">
</script>
</body>
</html>
Output:
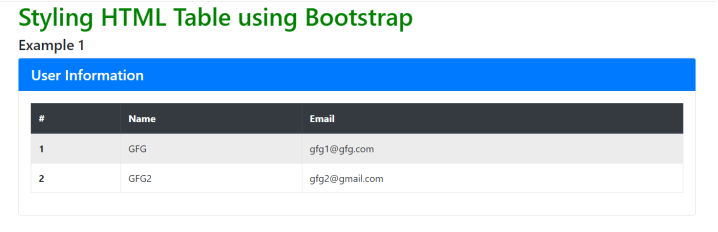
Using Animation and Bootstrap Hovering Classes
In this approach, we are styling the HTML table using Bootstrap classes such as “table”, “table-hover”, “table-bordered”, and “table-light” for a responsive and visually appealing layout. Additionally, we incorporate animations from the Animate.css library (“animate__animated”) with specific delays to create dynamic effects for table rows, along with contextual colors and text styles for enhanced visual presentation.
Example: The below example uses Animation and Bootstrap Hovering Classes to Style HTML Tables Using Bootstrap.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Example 2</title>
<link rel="stylesheet" href=
"https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<link rel="stylesheet" href=
"https://cdnjs.cloudflare.com/ajax/libs/animate.css/4.1.1/animate.min.css">
</head>
<body>
<div class="container">
<h1 class="text-success mt-4 mb-4">
Styling HTML table using Bootstrap
</h1>
<h4>
Example 2
</h4>
<div class="card">
<div class="card-header bg-primary text-white">
<h4 class="mb-0">User Information</h4>
</div>
<div class="card-body">
<div class="table-responsive">
<table class="table table-hover table-bordered">
<thead class="thead-light">
<tr>
<th scope="col">#</th>
<th scope="col">Name</th>
<th scope="col">Email</th>
</tr>
</thead>
<tbody>
<tr class="animate__animated
animate__bounceInDown
animate__delay-1s bg-info">
<th scope="row">1</th>
<td class="text-danger font-weight-bold">
GFG
</td>
<td>gfg1@gfg.com</td>
</tr>
<tr class="animate__animated
animate__fadeInUp
animate__delay-2s bg-warning">
<th scope="row">2</th>
<td class="text-success font-italic">
GFG2
</td>
<td>gfg2@gmail.com</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
<script src=
"https://code.jquery.com/jquery-3.5.1.slim.min.js">
</script>
<script src=
"https://cdn.jsdelivr.net/npm/@popperjs/core@2.5.3/dist/umd/popper.min.js">
</script>
<script src=
"https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js">
</script>
</body>
</html>
Output:
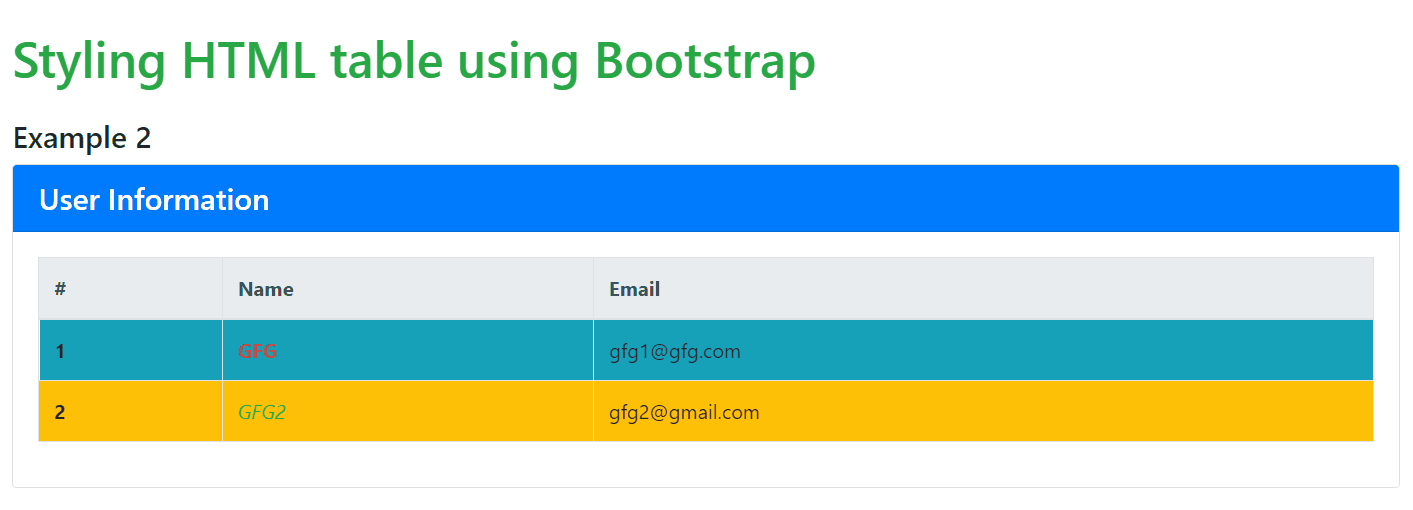
Using Bootstrap Table Plugin
In this approach, we are using Bootstrap table plugins to style HTML tables. The code includes Bootstrap and DataTables plugin for enhanced table functionality, such as sorting, searching, and pagination. This makes sure a visually appealing and interactive table layout with features provided by Bootstrap and DataTables integration.
Example: The below example uses Bootstrap Table Plugin to Style HTML Tables Using Bootstrap.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 3</title>
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<link rel="stylesheet" href=
"https://cdn.datatables.net/1.10.24/css/dataTables.bootstrap4.min.css">
</head>
<body>
<div class="container">
<h2 class="text-center my-4" style="color: green;">
Styling HTML Table using Bootstrap
</h2>
<h2 class="text-center my-4">
Using Bootstrap Table Plugins
</h2>
<div class="table-responsive">
<table id="example" class="table table-striped
table-bordered table-hover" style="width:100%">
<thead class="thead-dark">
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr>
<td>1</td>
<td>Ravi</td>
<td>ravi@gmail.com</td>
</tr>
<tr>
<td>2</td>
<td>Kavi</td>
<td>kavi@gmail.com</td>
</tr>
</tbody>
</table>
</div>
</div>
<script src=
"https://code.jquery.com/jquery-3.5.1.js">
</script>
<script src=
"https://cdn.datatables.net/1.10.24/js/jquery.dataTables.min.js">
</script>
<script src=
"https://cdn.datatables.net/1.10.24/js/dataTables.bootstrap4.min.js">
</script>
<script>
$(document).ready(function () {
$('#example').DataTable();
});
</script>
</body>
</html>
Output:
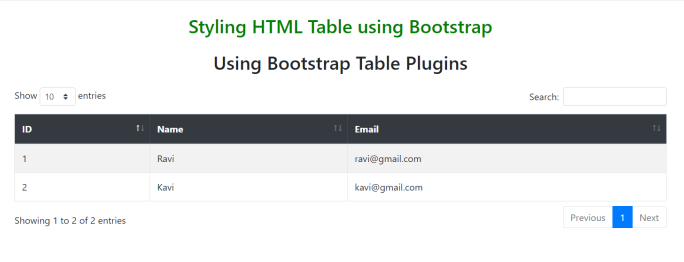
Using Bootstrap Theme
In this approach, we are using the Bootstrap Morph theme from Bootswatch to style the HTML table. The code includes the necessary Bootstrap classes for table formatting, such as table-hover for hover effects, along with specific classes like table-warning, table-success, and table-danger for row coloring. This makes a visually appealing and well-organized table layout with a themed design.
Example: The below example uses Bootstrap Theme to Style HTML Tables Using Bootstrap.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 4</title>
<link rel="stylesheet" href=
"https://cdnjs.cloudflare.com/ajax/libs/bootswatch/5.3.3/morph/bootstrap.min.css">
</head>
<body>
<div class="container">
<h2 class="text-center my-4" style="color: green;">
Styling HTML table using Bootstrap
</h2>
<h3 class="text-center my-4">
Using Bootstrap Theme
</h3>
<table class="table table-hover">
<thead>
<tr>
<th scope="col">ID</th>
<th scope="col">Name</th>
<th scope="col">Subject</th>
</tr>
</thead>
<tbody>
<tr class="table-warning">
<th scope="row">1</th>
<td>GFG1</td>
<td>HTML</td>
</tr>
<tr>
<th scope="row">2</th>
<td>GFG2</td>
<td>CSS</td>
</tr>
<tr class="table-success">
<th scope="row">3</th>
<td>GFG3</td>
<td>JavaScript</td>
</tr>
<tr class="table-danger">
<th scope="row">4</th>
<td>GFG4</td>
<td>Python</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
Output:
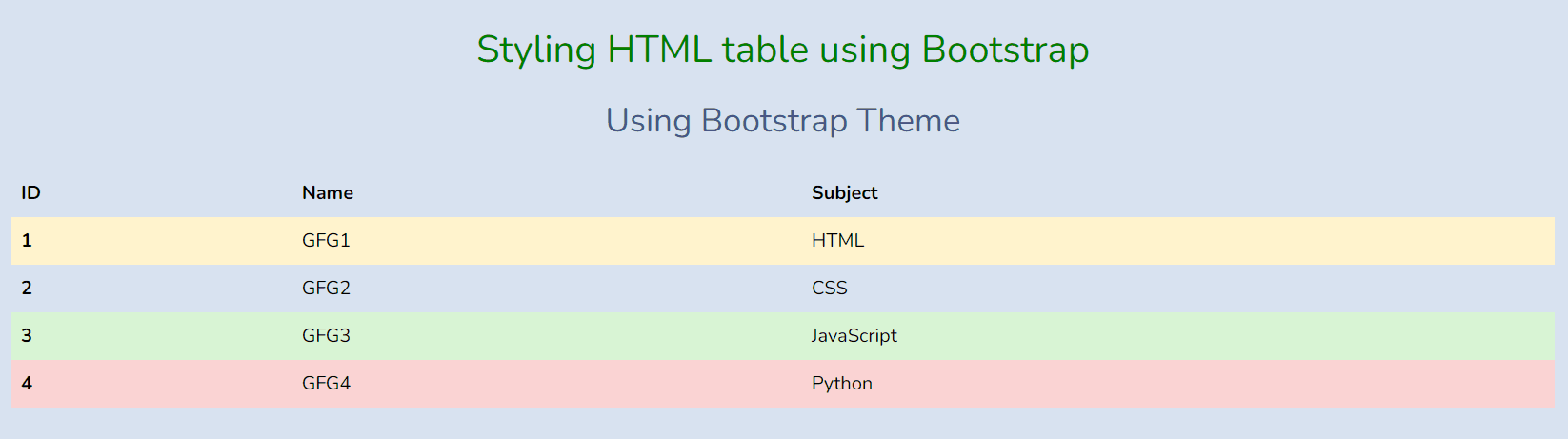
Share your thoughts in the comments
Please Login to comment...