How to set the Visibility of the RadioButton in C#?
Last Updated :
30 Jun, 2019
In Windows Forms, RadioButton control is used to select a single option among the group of the options. For example, select your gender from the given list, so you will choose only one option among three options like Male or Female or Transgender. In Windows Forms, you are allowed to adjust the visibility of the RadioButton using the Visible Property of the RadioButton.
If the value of this property is set to true, then RadioButton is visible on the form and if the value of this property is set to false, then RadioButton is not visible on the form. The default value of this property is true. You can set this property in two different ways:
1. Design-Time: It is the easiest way to set the visibility of the RadioButton as shown in the following steps:
2. Run-Time: It is a little bit trickier than the above method. In this method, you can set the visibility of the RadioButton control programmatically with the help of given syntax:
public bool Visible { get; set; }
The value of this property is of System.Boolean type. The following steps show how to set the visibility of the RadioButton dynamically:
- Step 1: Create a radio button using the RadioButton() constructor is provided by the RadioButton class.
// Creating radio button
RadioButton r1 = new RadioButton();
- Step 2: After creating RadioButton, set the Visible property of the RadioButton provided by the RadioButton class.
// Setting the visibility of the radio button
r1.Visible = true;
- Step 3: And last add this RadioButton control to the form using Add() method.
// Add this radio button to the form
this.Controls.Add(r1);
Example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp23 {
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e)
{
Label l = new Label();
l.AutoSize = true ;
l.Location = new Point(176, 40);
l.Text = "Select Post" ;
this .Controls.Add(l);
RadioButton r1 = new RadioButton();
r1.AutoSize = true ;
r1.Text = "Intern" ;
r1.Location = new Point(286, 40);
r1.Visible = true ;
this .Controls.Add(r1);
RadioButton r2 = new RadioButton();
r2.AutoSize = true ;
r2.Text = "Team Leader" ;
r2.Location = new Point(356, 40);
r2.Visible = true ;
this .Controls.Add(r2);
RadioButton r3 = new RadioButton();
r3.AutoSize = true ;
r3.Text = "Software Engineer" ;
r3.Location = new Point(470, 40);
r3.Visible = false ;
this .Controls.Add(r3);
}
}
}
|
Output:
Before setting the visibility of the RadioButtons:
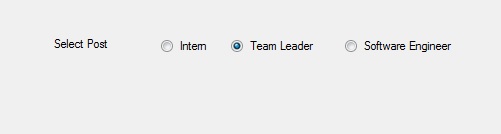
After setting the visibility of the RadioButtons:
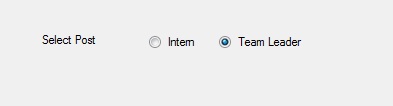
Share your thoughts in the comments
Please Login to comment...