How to Set & Retrieve Cookies using JavaScript ?
Last Updated :
13 Mar, 2024
In JavaScript, setting and retrieving the cookies is an important task for assessing small pieces of data on a user’s browser. This cookie can be useful for storing user preferences, session information, and many more tasks.
Using document.cookie
In this approach, we are using the document.cookie property to both set and retrieve cookies. When setting a cookie, we assign a string formatted as “name=value” to document.cookie, and to retrieve cookies, we split the cookie string and parse it into a key-value map.
Syntax:
document.cookie = "username=name;
expires=Thu, 18 Dec 2023 12:00:00 UTC; path=/";
Example: The below example uses document.cookie to set and retrieve cookies using JavaScript.
HTML
<!DOCTYPE html>
<head>
<title>Example 1</title>
</head>
<body>
<h1 style="color: green;">
GeeksforGeeks
</h1>
<h3>Using document.cookie</h3>
<input type="text" id="uInput"
placeholder="Enter cookie value">
<button onclick="setFn()">
Set Cookie
</button>
<button onclick="getFn()">
Get Cookie
</button>
<p id="cookieVal"></p>
<script>
function setFn() {
const value =
document.getElementById('uInput').value;
document.cookie =
`exampleCookie=${value}`;
alert('Cookie has been set!');
}
function getFn() {
const cookies =
document.cookie.split('; ');
const cookieMap = {};
cookies.forEach(cookie => {
const [name, value] = cookie.split('=');
cookieMap[name] = value;
});
const cookieVal = cookieMap['exampleCookie'];
document.getElementById('cookieVal').textContent =
`Cookie Value: ${cookieVal}`;
}
</script>
</body>
</html>
Output:
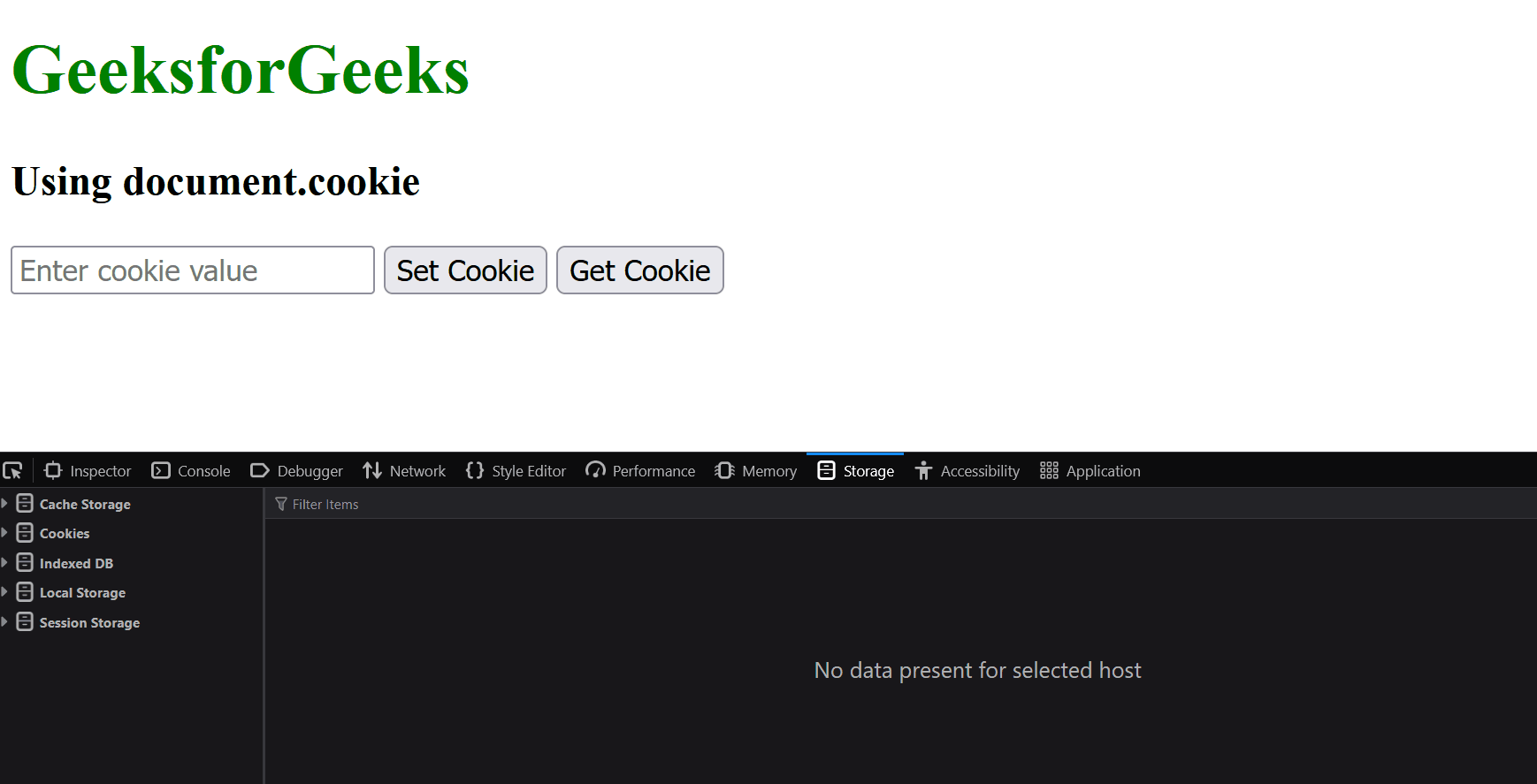
Using js-cookie library
In this approach, we are using the js-cookie library, which provides a simple way to manage cookies in JavaScript. This library has methods like Cookies.set() and Cookies.get() to easily set and retrieve cookies.
CDN Link:
Add this CDN link to your HTML document to use js-cookie library.
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/js-cookie/3.0.1/js.cookie.min.js">
</script>
Syntax:
Cookies.set('cookie-name', value);
const cVal = Cookies.get('cookie- name');
Example: The below example uses js-cookie library to set and retrieve cookies using JavaScript.
Javascript
<!DOCTYPE html>
<head>
<title>Example 2</title>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/js-cookie/3.0.1/js.cookie.min.js">
</script>
</head>
<body>
<h1 style="color: green;">
GeeksforGeeks
</h1>
<h3>Using js-cookie library</h3>
<input type="text" id="uInput"
placeholder="Enter cookie value">
<button onclick="setFn()">
Set Cookie
</button>
<button onclick="getFn()">
Get Cookie
</button>
<p id="cVal"></p>
<script>
function setFn() {
const value = document.
getElementById('uInput').value;
Cookies.set('newCookie', value);
alert('Cookie has been set!');
}
function getFn() {
const cVal = Cookies.get('newCookie');
document.getElementById('cVal').textContent =
`Cookie Value: ${cVal}`;
}
</script>
</body>
</html>
Output:
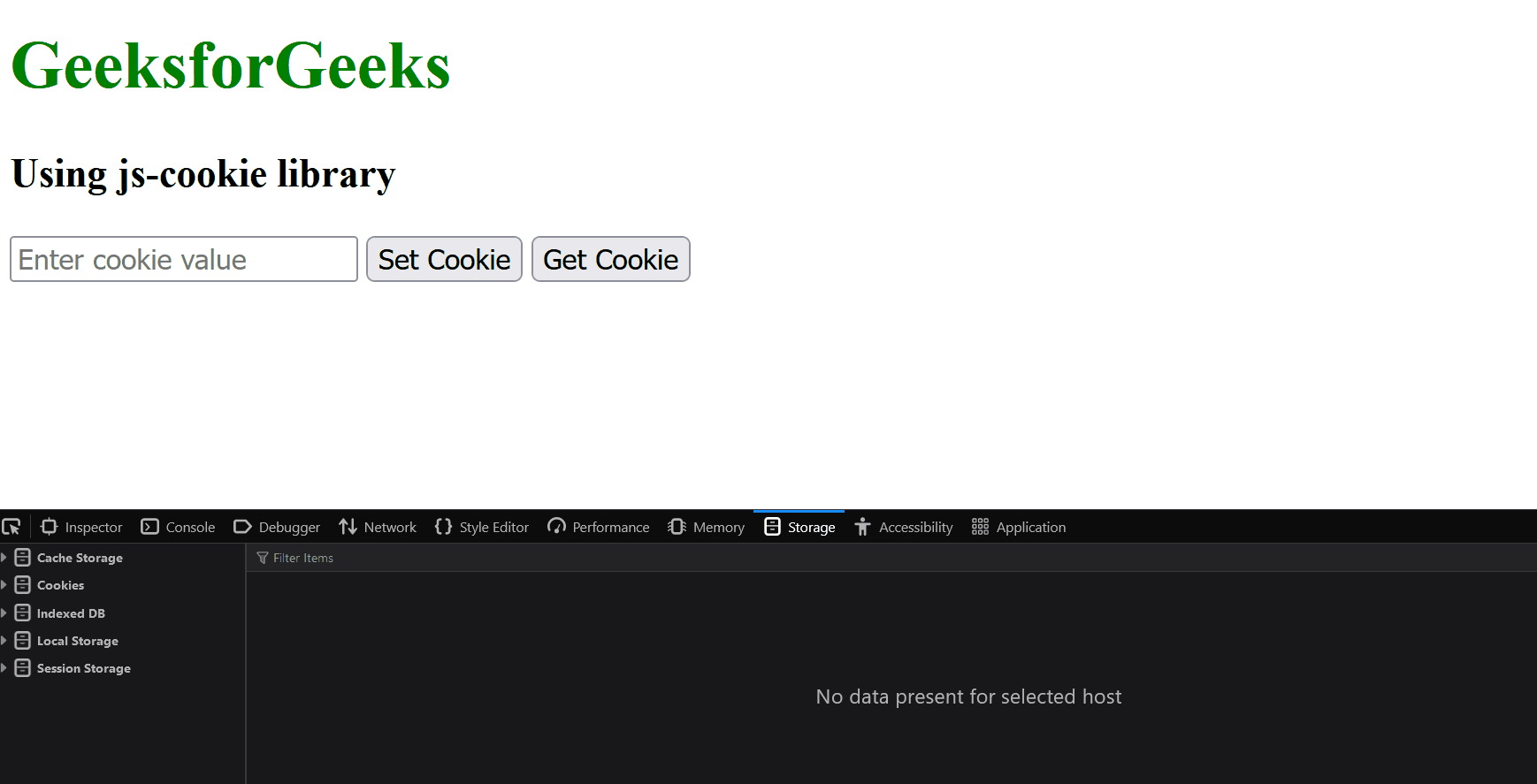
Share your thoughts in the comments
Please Login to comment...